Question
*JAVA* This is an object oriented programming exercise. Create a PhoneBook class. The class should have a private HashMap instance variable. When an object of
*JAVA*
This is an object oriented programming exercise.
Create a PhoneBook class.
The class should have a private HashMap instance variable. When an object of the PhoneBook class is constructed, it should read a serialized HashMap object from file and assign that HashMap object to the HashMap instance variable. (Use the serialized HashMap object from your first exercise as the initializing file).
Give the class the following methods:
- A method with a loop that asks the user to provide a name. The HashMap object is consulted and the matching phone number is returned. The loop should keep performing until the user tells it to stop.
- A method with a loop that requests name and phone number pairs. They are stored in the HashMap object. The loop should keep performing until the user tells it to stop.
- A method that serializes the HashMap object to file.
- A method that reads the Hashmap object back from file. The reconstituted Hashmap object will be assigned to the Hashmap instance variable.
You should have a main method that is your actual program. That program instantiates a Phonebook object. Then it should display a menu that gives the user the options to get phone numbers, assign names and phone numbers, and exit. When you exit, the HashMap object should be saved to file by serialization.
PHONEBOOK CLASS(I have not created a main yet):
import java.util.*; import java.io.*;
public class PhoneBook {
{ HashMap phonebook = null; try { FileInputStream fis = new FileInputStream("phonebook.ser"); ObjectInputStream ois = new ObjectInputStream(fis); phonebook = (HashMap) ois.readObject(); ois.close(); fis.close(); } catch (IOException ioe) { ioe.printStackTrace(); } catch (ClassNotFoundException c) { System.out.println("Class not found"); c.printStackTrace(); } System.out.println("Deserializing Finished. Phonebook Data: "); Set set = phonebook.entrySet(); Iterator iterator = set.iterator(); while (iterator.hasNext()) { Map.Entry read = (Map.Entry) iterator.next(); System.out.print("Name: " + read.getKey() + " | Phone Number: "); System.out.println(read.getValue()); } } }
HERE is the main method of a similar program:
import java.util.*; import java.io.*;
public static void main(String[] args) { boolean goOn = true; HashMap
Scanner sc = new Scanner(System.in); do { System.out.print("Please enter a name: "); String name = sc.nextLine(); String phoneNumber = contacts.get(name); if (phoneNumber != null) { System.out.println("Phone Number: " + phoneNumber); } System.out.print("Do you want to continue (Y or N): "); char inputC = sc.next().charAt(0); if (Character.toLowerCase(inputC) == 'n') { goOn = false; } sc.nextLine(); } while (goOn); try { FileOutputStream fos = new FileOutputStream("phonebook.ser"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(contacts); oos.close(); fos.close(); System.out.printf("Phonebook data is saved in phonebook.ser"); } catch (IOException ioe) { ioe.printStackTrace(); } goOn = false; System.out.print(" Do you want to deserialize the data? (Y or N): "); char inputC = sc.next().charAt(0); if (Character.toLowerCase(inputC) == 'n') { goOn = false; } else { goOn = true; } sc.nextLine(); if (goOn == true) { HashMap
Step by Step Solution
There are 3 Steps involved in it
Step: 1
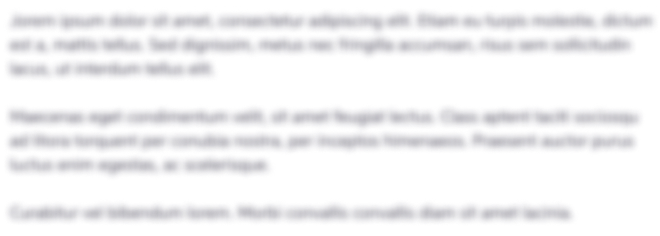
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started