Question
*JAVA* This program will take the classic tongue-twister Peter Piper picked a peck of pickled peppers. and generate a tongue twister phrase based on user
*JAVA*
This program will take the classic tongue-twister "Peter Piper picked a peck of pickled peppers." and generate a tongue twister phrase based on user input for a last name, a unit of measurement and a vegetable. For all three cases, the user input may be multiple words.
Once read, the user input will be modified to fit the following rules:
1) last name: No leading or trailing whitespace; the first character will be upper case and the rest lower case.
2) unit of measurement and vegetable: No leading or trailing whitespace; all the characters in lower case.
Consider the following example with interleaved input (Zhang meter pumpkin , where ' ' is a new line) and output:
Enter your last name: Zhang Enter a unit of measurement: meter Enter the name of a vegetable: pumpkin A tongue twister: Peter Zhang picked a meter of pickled pumpkins.
Note that in this program, unlike the previous ones, the prompts are not followed by a newline. That is, the input is:
Zhang meter pumpkin
and the output is:
Enter your last name: Enter a unit of measurement: Enter the name of a vegetable: A tongue twister: Peter Zhang picked a meter of pickled pumpkins.
Suggestion: Methods in the Scanner class (next() and nextLine()), String class (trim(), substring(), toUpperCase(), charAt(), etc.) and Character class may be helpful for this program.
As the problems get more complicated, it is good to divide them up into smaller, easier to handle parts. Let's break this problem down into two parts: (1) a method for formatting the last name and (2) input and output in the main method.
(1) a) Copy the following method into your SillyString class.
/** * This method returns the name with the first letter * in upper case and the rest lower case. Any spaces at * the beginning or end are removed. Does not change * spaces in the middle of the string. * For multiple word names, only the first letter of the * first word should be upper case, all the rest lower case. * @param name a name that may be mixed case. * @return the name in proper case. */ public static String properCase(String name) { //FILL IN BODY }
b) Before implementing the properCase method, think about the different types of values that might be passed in to name. Copy the following method into your SillyString class and replace the /*FIX ME*/ with 3 different calls to the properCase method. Each call should test a different type of input to the method. You should have a minimum of 3 tests, but you may have more.
/** * Runs tests on the properCase method. */ public static void testProperCase() { System.out.println(/*FIX ME*/); System.out.println(/*FIX ME*/); System.out.println(/*FIX ME*/); }
c) Implement the properCase method and use the testProperCase method to test it before submitting.
(2) Complete the main method so that the output is produced and the input is read as described above.
Thanks to Bob Roos, Allegheny College for inspiration for this assignment.
Given:
import java.util.Scanner;
public class SillyString {
public static void main(String[] args) {
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
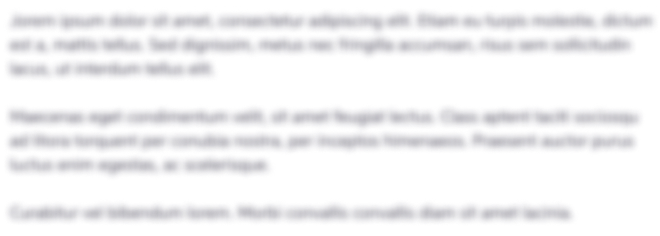
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started