Question
(JAVA) Use starter code MetaCollection.java and Test.java. The code must work with Test.java and finally everything must be put in a package called booklist. Side
(JAVA) Use starter code MetaCollection.java and Test.java. The code must work with Test.java and finally everything must be put in a package called booklist.
Side note for some reason the formatting is a bit funky on this post thus some parts that seem cut off are there you just have to side scroll with the mouse or the arrow keys.
Starter Code:
******************************************************************************
MetaCollection.java
package booklist;
import java.util.AbstractCollection;
import java.util.Iterator;
import java.util.Collection;
import java.util.ArrayList;
public class MetaCollection
//keep reference added collections in this list
private final ArrayList
public void addCollection(Collection
}
@Override
public Iterator
return new JoinedIter();
}
@Override
public int size() {
}
}
******************************************************************************
Test.java
import booklist.ListUtil;
import booklist.MetaCollection;
import booklist.PhoneUtil;
import java.math.BigInteger;
import java.util.*;
import java.util.function.Predicate;
public class Test {
public static void main(String[] args) {
//test phone book
MapphoneBook = new HashMap<>();
phoneBook.put("Alice", new BigInteger("2131231234"));
phoneBook.put("Bob", new BigInteger("3101231234"));
phoneBook.put("Charles", new BigInteger("2121231234"));
System.out.println("Original phone book");
for (Map.Entryentry: phoneBook.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
System.out.println("Updated phone book");
PhoneUtil.prependOne(phoneBook);
for (Map.Entryentry: phoneBook.entrySet())
System.out.println(entry.getKey() + ": " + entry.getValue());
Sets1 = new HashSet<>();
Collections.addAll(s1, 11,-22,33,-44);
ArrayListl1 = new ArrayList<>();
Collections.addAll(l1, 1.1, 2.2, 3.3);
System.out.println("Merge two Collections");
Collectionc1 = ListUtil.merge(s1, l1);
for (Object n : c1)
System.out.println(n);
System.out.println("Select positive entries from s1");
//select from a Collection
for (Integer i : ListUtil.select(s1,new Pred()))
System.out.println(i);
Sets2 = new HashSet<>();
Collections.addAll(s2, 1,2,3);
ArrayListl2 = new ArrayList<>();
Collections.addAll(l2, 10);
//create a MetaCollectionfrom Collection s
MetaCollectionmc1 = new MetaCollection<>(s2, l2, s2);
l2.add(20);
System.out.println("Meta collection output 1");
System.out.println(mc1.size()); //output 8
for (Integer i : mc1)
System.out.println(i);
MetaCollectionmc2 = new MetaCollection<>();
mc2.addCollection(new HashSet<>());
mc2.addCollection(new PriorityQueue<>());
mc2.addCollection(new ArrayList<>());
System.out.println("Meta collection output 2");
System.out.println(mc2.size()); //output 0
for (Number i : mc2) //no output
System.out.println(i);
//see how changing one Collectionaffects another Collection
ArrayListl3 = new ArrayList<>();
l3.add(new Complex(1,2));
ArrayListl4 = new ArrayList<>();
l4.add(new Complex(2,3));
ArrayListl5 = ListUtil.merge(l3, l4);
Complex cpx = l5.get(0);
cpx.real = 0;
cpx.imag = 0;
l5.set(1, new Complex(8,9));
System.out.println("Why do we have the following output?");
System.out.println(l3.get(0)); //0.0+0.0i
System.out.println(l4.get(0)); //2.0+3.0i
System.out.println(l5.get(0)); //0.0+0.0i
System.out.println(l5.get(1)); //8.0+9.0i
}
}
class Pred implements Predicate{
@Override
public boolean test(Number n) {
return n.doubleValue()>=0;
}
}
class Complex {
public double real, imag;
public Complex(double real, double imag) {
this.real = real;
this.imag = imag;
}
@Override
public String toString() {
return real + "+" + imag + "i";
}
}
*************************************************************************************
Imagine you have a Mapthat represents a phone book. Until now, you stored all (US) phone numbers as the usual 10 digit number. (These numbers do not start with a 0.)
One day, your business partner from London gives you the phone number 44-020-1234-1234, and you realize that you need to add a 1 to the beginning of all existing phone number entries to indicate that they are US phone numbers. (1 is the country code for the US.)
Write the function
public static void prependOne(Mapm)
that adds a 1 to the beginning of each 10-digit BigInteger. Place this function within the utility class PhoneUtil.
*************************************************************************************
Read the documentation of java.util.function.Prediateand java.util.AbstractCollection . Note that default methods are methods that you do not have to implement.
*************************************************************************************
Write a utility class ListUtil that provides the following 2 methods.
public staticArrayList merge(Collection extends E> c1, Collection extends E> c2)
merge returns an ArrayListthat contains all elements of c1 and c2. You will find the addAll method of List useful. *************************************************************************************
public staticArrayList select(Collection extends E> coll, Predicate super E> pred)
select returns an ArrayListthat contains all elements of coll for which pred.test() evaluates to true.
*************************************************************************************
Write the generic class MetaCollection, which serves as a concatenation of many Collection s. Make MetaCollection inherit AbstractCollection .
For efficiency reasons,
private ArrayList> collectionList;
is the only Collectionyou may use as a field. In particular, you may note create additional Collection s.
*************************************************************************************
Write the constructor
public MetaCollection(Collectionc_arr)
which takes in a variable number of Collections, and initializes the MetaCollection to be the concatenation of them.
*************************************************************************************
Write the method
public void addCollection(Collectioncoll)
so that it adds coll to the MetaCollection. (So there are 2 ways to add a Collection to the MetaCollection . One is via the constructor and the other is via addCollection().)
*************************************************************************************
Implement the method
public int size()
so that it returns the sum of the sizes of the Collections the MetaCollection consists of.
*************************************************************************************
Implement the method
public Iteratoriterator()
So that it simply returns a JoinedIter instance.
*************************************************************************************
Write the private inner class
private class JoinedIter implements Iterator
A JoinedIter instance iterates through all Es of the Collections the MetaCollection consists of.
*************************************************************************************
Remark. Do not define the class as
private class JoinedIterimplements Iterator //dont do this
If you do so, the generic type E of the inner class hides the generic type E of the top-level class, which is not what you want.
*************************************************************************************
Hint. Let JoinedIter have a field private int itrCounter. Initialize itrCounter to 0 and increment it every time next() is called. Then hasNext() can return (itrCounter ************************************************************************************* Hint. JoinedIter must use an Iteratorwhen iterating through a given Collection . JoinedIter can keep track of which Collection within collectionList it is currently going through with an Iterator > or an index stored as an int. *************************************************************************************Remark. The two-tiered structure of this problem is initially confusing, but a correct solution can be simple. If your code gets long and complicated, try to see if you can simplify your logic, As a guideline, JoinedIter can be written with 20 lines of code.*************************************************************************************In the last lines of Test.java, we haveArrayListl3 = new ArrayList<>(); l3.add(new Complex(1,2));ArrayListl4 = new ArrayList<>(); l4.add(new Complex(2,3));ArrayListl5 = ListUtil.merge(l3, l4); Complex cpx = l5.get(0);cpx.real = 0;cpx.imag = 0;l5.set(1, new Complex(8,9));System.out.println("Why do we have the following output?");System.out.println(l3.get(0)); //0.0+0.0iSystem.out.println(l4.get(0)); //2.0+3.0iSystem.out.println(l5.get(0)); //0.0+0.0iSystem.out.println(l5.get(1)); //8.0+9.0iThink about why the 0th entry of 13 did change while the 0th entry of 14 did not.*************************************************************************************
Step by Step Solution
There are 3 Steps involved in it
Step: 1
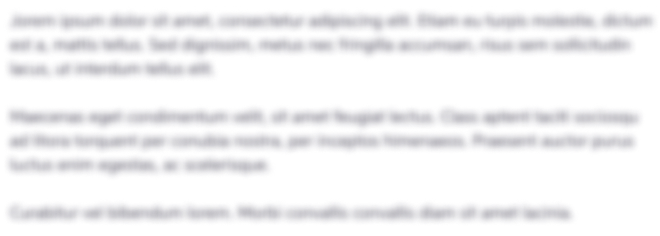
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started