Question
JAVA use the concepts in concurrent programming to write a basic multi-threaded program. You will be creating a program that simulates the sound of soldiers
JAVA
use the concepts in concurrent programming to write a basic multi-threaded program. You will be creating a program that simulates the sound of soldiers marching: Left, Left, Left, Right, Left.
You will need one class to print Left and one to print Right, as well as a class to drive the program.
LeftThread.java
public class LeftThread extends Thread { public void run() { for(int i = 0; i < 10; i++) { //TODO: Print "Left" //TODO: Print "Left" //TODO: Yield and allow right thread an opportunity to take over //TODO: Print "Left" } }
RightThread.java
public class RightThread /*TODO: What should this extend?*/ { public void run() { //TODO: Use LeftThread as template to print "Right" and yield for left thread //Make sure this process repeats 10 times like in the LeftThread } }
MilitaryMarching.java
public class MilitaryMarching { public static void main(String[] args) { //TODO: Create left and right thread objects //TODO: Start left and right thread objects try { //TODO: Join left and right thread objects } catch(InterruptedException e) { e.printStackTrace(); } } }
Is there a problem with this implementation? Why does it sometimes give the wrong output?
This implementation will likely give you the right result the majority of the time, however sometimes LeftThread and RightThread will be running on different processor cores. In this case, yielding will not work because there is no other process on the same processor to yield to.
What would we need to do to fix this problem?
To fix this issue, we will need to poll our threads. This means, we would need variables in each of our threads corresponding to the other thread to keep track of whether it is done or not. Our LeftThread would need a boolean, done, and a reference to our RightThread, right. Or RightThread would also need a boolean, done, and a reference to our LeftThread, left. We can keep track of whether the other thread is done before progressing into our print statements. Once we print, we can set our current thread to done so that the other thread knows to take over.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
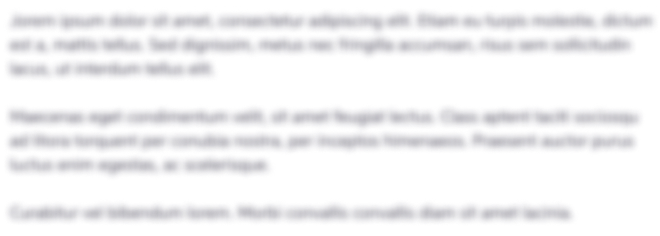
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started