Question
JAVA WHY DOES IT SAY 'cannot find symbol - class LocalDate' WHEN I TRY TO COMPILE question; Modify the supplied book shop system to include
JAVA
WHY DOES IT SAY 'cannot find symbol - class LocalDate' WHEN I TRY TO COMPILE
question;
Modify the supplied book shop system to include a private instance variable called publishDate in class Book to represent when the book was published. Use LocalDate as the variable type. Use a static variable in the Book class to automatically assign each new book a unique (incremental) id number. Create an array of Book variables to store references to the various book objects. In a loop, calculate the cost of each book type and the sum total of the order. If the book is of classic type and is more than 50 years old, give the cost a 35% discount. If the book is of novel or education type and is more than 5 years old, give the cost a 12% discount. Change the cost() method in all sub-classes of Book to throw a user defined Exception if the total cost is less than zero. This exception should print an error message showing the details of the problem. Modify the Test class to be able to handle exceptions. When an exception is encountered while calculating the cost of a book, the Test class should print out the error message and continue as normal with the next books. This implementation shoud be tested by creating books with negative cost.
answer:
Test.java
// Java core packages import java.text.DecimalFormat;
// Java extension packages import javax.swing.JOptionPane;
public class Test {
// test book hierarchy
public static void main(String args[]) {
Book book; // superclass reference
String output = "";
Novel novel = new Novel("Harry Potter", "J.K Rowling", 20.0, LocalDate.of(1992, 3, 26));
EducationBook educationbook = new EducationBook("My Maths 3", "James Jones", 15.6, 180654203, 4,
LocalDate.of(1913, 3, 26));
Classic classic = new Classic("The Great Gatsby", "F. Scott Fitzgerald", 35.0, LocalDate.of(1800, 3, 26));
DecimalFormat precision2 = new DecimalFormat("0.00");
// book reference to a novel
book = novel;
try {
output += book.toString() + " costs $" + precision2.format(book.cost()) + " " + novel.toString()
+ " costs $" + precision2.format(novel.cost()) + " ";
// book reference to a educationbook
book = educationbook;
output += book.toString() + " costs $" + precision2.format(book.cost()) + " " + educationbook.toString()
+ " costs $" + precision2.format(educationbook.cost()) + " ";
// book reference to a Classic
book = classic;
output += book.toString() + " costs $" + precision2.format(book.cost()) + " " + classic.toString()
+ " costs $" + precision2.format(classic.cost()) + " ";
} catch (Exception e) {
System.out.println(e.getMessage());
}
Book books[] = new Book[5];
books[0] = novel;
books[1] = educationbook;
books[2] = classic;
EducationBook educationbook1 = new EducationBook("My Maths 4", "James Jones", 15.6, 180654203, -4,
LocalDate.of(1913, 3, 26));
Classic classic2 = new Classic("The Greatest Harry", "F. SNEwewcott Fitzgerald", 35.0,
LocalDate.of(2018, 3, 26));
books[3] = educationbook1;
books[4] = classic2;
double totalCost = 0;
for (int i = 0; i
try {
if (books[i] instanceof Classic) {
if (books[i].getPublishDate().compareTo(LocalDate.of(books[i].getPublishDate().getYear() - 50,
books[i].getPublishDate().getMonth(), books[i].getPublishDate().getDayOfMonth())) > 0) {
totalCost = totalCost + (books[i].cost()*0.35 + books[i].cost());
} else {
totalCost = totalCost + books[i].cost();
}
} else {
if (books[i].getPublishDate().compareTo(LocalDate.of(books[i].getPublishDate().getYear() - 5,
books[i].getPublishDate().getMonth(), books[i].getPublishDate().getDayOfMonth())) > 0) {
totalCost = totalCost + (books[i].cost()*0.12 + books[i].cost());
} else {
totalCost = totalCost + books[i].cost();
}
}
output = output + "Book : "+(i+1)+books[i]+" , cost : "+books[i].cost() + " ";
}catch(Exception e) {
output = output + books[i].toString()+" Cost Error: "+e.getMessage() + " ";
}
}
output = output + "Total Cost : "+totalCost;
JOptionPane.showMessageDialog(null, output, "Demonstrating Polymorphism", JOptionPane.INFORMATION_MESSAGE);
System.exit(0);
}
} // end class Test
Book.java
// Abstract base class Book.
public abstract class Book {
private String bookTitle;
private String bookAuthor;
private LocalDate publishDate;
private static int bookId = 0;
private int id;
// constructor
public Book(String title, String author, LocalDate pubDate) {
bookTitle = title;
bookAuthor = author;
publishDate = pubDate;
bookId++;
id = bookId;
}
// get bookTitle
public String getbookTitle() {
return bookTitle;
}
// get bookAuthor
public String getbookAuthor() {
return bookAuthor;
}
public int getId() {
return id;
}
public String toString() {
return id + " " + bookTitle + " " + bookAuthor + " "+ publishDate.toString();
}
public LocalDate getPublishDate() {
return publishDate;
}
public abstract double cost() throws Exception;
}
EducationBook.java
// EducationBook class derived from Book
public final class EducationBook extends Book {
private double bookCost; // cost of the book
private int bookIsbn; // the unique identifier of the education book
private int quantity; // number of this book required
// constructor for class EducationBook
public EducationBook(String title, String author, double cost, int isbn, int quantity,LocalDate pubDate) {
super(title, author,pubDate); // call superclass constructor
setBookCost(cost);
setIsbn(isbn);
setQuantity(quantity);
}
// set EducationBook's cost
public void setBookCost(double cost) {
bookCost = cost;
}
// set EducationBook's isbn
public void setIsbn(int isbn) {
bookIsbn = (isbn > 0 ? isbn : 0);
}
// set EducationBook's quantity sold
public void setQuantity(int totalSold) {
quantity = totalSold;
}
// determine EducationBook's total cost
public double cost() throws Exception {
if(bookCost * quantity
throw new Exception("Total Book Cost cannot be negative.");
}
return bookCost * quantity;
}
// get String representation of EducationBook's details
public String toString() {
return "Education Book: " + super.toString();
}
} // end class EducationBook
Classic.java
// Definition of class Classic
public final class Classic extends Book {
private double bookCost; // cost of the book
// constructor for class Classic
public Classic(String title, String author, double cost, LocalDate pubDate) {
super(title, author, pubDate); // call superclass constructor
setBookCost(cost);
}
// Set the cost of the book
public void setBookCost(double cost) {
bookCost = cost;
}
// Get the Classic book's cost
public double cost() throws Exception {
if(bookCost
throw new Exception("Total Book Cost cannot be negative.");
}
return bookCost;
}
public String toString() {
return "Classic: " + super.toString();
}
}
Novel.java
// Novel class derived from Book.
public final class Novel extends Book {
private double bookCost;
// constructor for class Novel
public Novel(String title, String author, double cost, LocalDate pubDate) {
super(title, author, pubDate); // call superclass constructor
setBookCost(cost);
}
// set Novel's cost
public void setBookCost(double cost) {
bookCost = cost;
}
// get Novel's cost
public double cost() throws Exception {
if(bookCost
throw new Exception("Total Book Cost cannot be negative.");
}
return bookCost;
}
// get String representation of book details
public String toString() {
return "Novel: " + super.toString();
}
} // end class Novel
output;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
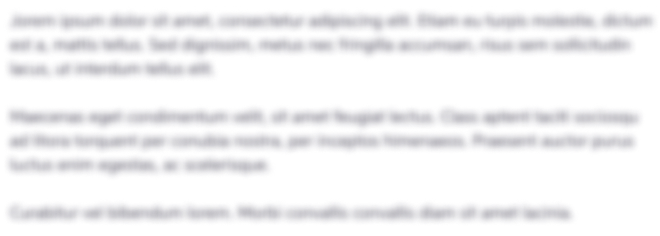
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started