Question
JAVA: Write an application to test the HuffmanTree class. Your application will need to read a text file and build a frequency table for the
JAVA: Write an application to test the HuffmanTree class. Your application will need to read a text file and build a frequency table for the characters occurring in that file. Once that table is built, create a Huffman code tree and then a string consisting of '0' and '1' digit characters that represents the code string for that file. Read that string back in and recreate the contents of the original file.
Here is my HuffTree class:
import java.io.PrintStream; import java.io.Serializable; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.util.*;
public class HuffmanTree implements Serializable { public static class HuffData implements Serializable { private double weight; private Character symbol;
public HuffData(double weight, Character symbol) { this.weight = weight; this.symbol = symbol; }
public Character getSymbol() {return symbol;} } protected BinaryTree
@Override public int compare(BinaryTree
public void buildTree(HuffData[] symbols) { Queue
// Build the tree. while (theQueue.size() > 1) { BinaryTree
private void printCode(PrintStream out, String code, BinaryTree
public void printCode(PrintStream out) { printCode(out, "", huffTree); } public String decode(String codedMessage) { StringBuilder result = new StringBuilder(); BinaryTree
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
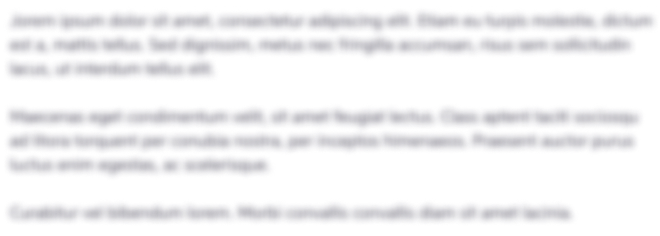
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started