Question
JAVA YAHTZEE PROGRAM: How do I add the following (left column) to the existing code (right column)? Having a hard time understanding some of the
JAVA YAHTZEE PROGRAM: How do I add the following (left column) to the existing code (right column)?
Having a hard time understanding some of the wording and getting my program to run without any errors. Thank you!
AiPlayer class | Questions to answer: Update method selectCategory() to do the following Add parameter of class Roll based on updated IPlayer interface Comment out or delete the statement that throws the UnsupportedOperationException Implements abstract method selectDice() from superclass Player, remove any autogenerated body Implements abstract method calculateScore() from superclass Player, remove any autogenerated body | Exisiting Code:
| |
Constants class | Update the class to include two constants as follows ZERO set equal to the value of 0 MAX_ROLLS set equal to the value of 3 |
| |
Die class |
| ||
Game class | Update method playGame() to do the following Create a local variable of data type int called roll initialized to the value of 0 In the for loop that loops through the players of the gamePrior to the call to player.rollDice(dice)instantiate an instance of class Roll that will store the selected dice of the player for each roll for the reference object described in the previous step, call method removeDice() passing as an argument the reference object to empty the ArrayList of class Die Add a while loop based on the condition that the selected dice ArrayList size is less than the maximum number of dice AND the player has not exceeded the maximum number of rolls Inside the while loop should be the followingThe call to player.rollDice(dice) Call to method selectDice() on class Player passing as argumentsThe original Roll object reference The Roll object for the dice to keep The int for the current roll number Increment the local variable roll of data type int After the while loop, call method selectCategory() in class Player passing as an argument the reference object of class Roll that represents the kept dice by the player |
| |
HumanPlayer class | Add a custom constructor that does the following Access level modifier public Empty parameter list Instantiates the member variable of class ScoreCard Update method selectCategory() to do the followingAdd parameter of class Roll based on updated IPlayer interface representing the dice the player selected to keep Prompt the player to select a category Using an instance of class Scanner, receive the players selection Loop until the player selects a valid category, data validation must check forUsing try/catch exception handling verify the player entered an integer Verify the player entered a valid value based on the 13 categories to select from After the player selects a valid category call method calculateScore() passing as argumentsthe instance of class roll received as a parameter the int of the category selected Add method calculateScore to do the followingAccess level modifier public Return type void Parameter listInstance of class Roll representing the dice the player selected Primitive data type int representing the selected category If the category selected is part of the upper section, referencing the member variable of class ScoreCard call the getter for UpperSection and call method evaluateCategory() passing as arguments the instance of class Roll representing the kept dice and the category selected If the category selected is part of the upper section, referencing the member variable of class ScoreCard call the getter for LowerSection and call method evaluateCategory() passing as arguments the instance of class Roll representing the kept dice and the category selected Output to the console the total score from class ScoreCard Add method selectDice() to do the followingAccess level modifier public No return type Parameter listInstance of class Roll representing the member variable instantiated in class Game Instance of class Roll representing the object instantiated for the dice to keep Primitive data type int representing the current roll number of the player Prompt the player to select the dice they want to keep based on the index value displayed in front of the die value, offer an option to end the current turn of their roll (note: each player gets three rolls per turn) Loop until the player enters that they are done selecting and their selection was validIf the player selects that they are doneCheck if all dice have been selected AND they are on their third rollIf truetransfer all remaining dice in the original Roll instance to the keep Roll instance remove each die from the original Roll instance Update the variable monitoring if the player is done by setting it to true Break out of the looping structure ElseInside a try/catch exception handlerParse the received data from the player so it is an integer representing the index to the ArrayList of Die Get the Die instance from the ArrayList and store as an instance of class Die Add the Die to the instance of class Roll representing the keep dice Remove the Die from the instance of class Roll representing the original dice Loop through the collection of Die to keep in the instance of class Roll that represents the keep dice and output to the console If the size of the ArrayList of Die in class Roll is the maximum number of dice, break out of the looping structure | package core; public class HumanPlayer extends Player { @Override public void rollDice(Roll r) { for(Die die : r.getDice()) { die.rollDie(); } } @Override public void selectCategory() { throw new UnsupportedOperationException("Not supported yet!"); } } | |
IPlayer interface | Update the method signature for method selectCategory() so that it has one parameter of class Roll | package core; public interface IPlayer {public void rollDice(Roll r);public void selectCategory(); } |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
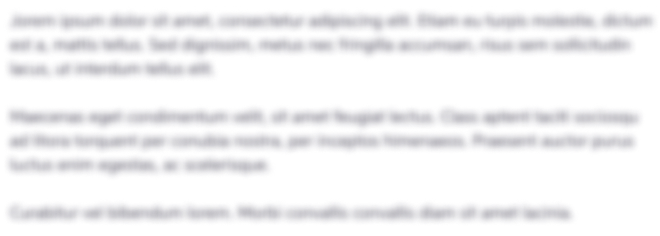
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started