Question
Java You have been given a simple API to buy and print postage. Write a program that submits each incoming order to the API and
Java
You have been given a simple API to buy and print postage. Write a program that submits each incoming order to the API and then prints the shipping label.
Unfortunately each call to the shipping label API is SLOW To compensate for this, use CONCURRENCY so that your program can be making several calls to the API at one time.
We also need to keep track of our expenses, so make sure to calculate how much money is being spent on shipping.
Starter Code:
1) ShippingService.java
https://drive.google.com/open?id=1SknHyOgUL5gH07hHJQQ_MZjsbTdSCDCe
2) ShippingLabel.java
https://drive.google.com/open?id=1aeUvOarp_7wc5jCU6vDATejZeZsJPXLX
Details:
The attached code simulates an API that generates shipping labels. Include it with your code.
1) Create an "Order" class to represent an incoming order with the following fields
a. Customer Name
b. Customer Address
c. Pounds of Chocolate
2) Create a list to hold incoming orders and populate it with at least 6 orders
3) Create an ExecutorService instance to manage threads
4) Write a Runnable class to process each order by calling the API to generate a shipping label
a) "Print" each label by printing it to the console
i. Although ShippingLabel does have a toString() method, it is not very readable. Print the shipping label in a more user-friendly way.
ii. Watch out for concurrency problems!
b) Keep track of the total cost of all shipments
i. There are many ways to do this - use any mechanism you like, but make sure that you account for any possible race conditions
5) Cleanly shutdown the ExecutorService
6) Print the total cost after all orders have been filled
Step by Step Solution
There are 3 Steps involved in it
Step: 1
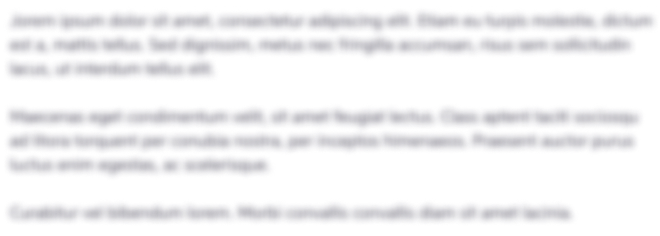
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started