Question
JAVASCRIPT Class and Constructor Creation Book Class Create a script called library.js. In this file create a constructor function for a Book object. The Book
JAVASCRIPT
Class and Constructor Creation Book Class Create a script called library.js. In this file create a constructor function for a Book object. The Book object should have the following properties: Title Available: Boolean representing whether the book is checked out or not. The initial value should be false. Publication Date: Use a date object
Checkout Date: Use a date object Call Number: Make one up Authors: Should be an array of Author objects Author Class Create a constructor function for an object called Author. It should have a property for the first name and last name of the author.
Patron Class
Create a constructor function for an object called Patron. This represents a person who is allowed to check out books from the library.
Give it the following properties:
Firstname
Lastname
Library Card Number (Make one up)
Books Out: Make it an array
fine: Starts a 0.00 B. Methods to add Book Class Add a function to the Book prototype called "checkOut". The function will change the available property of the book from true to false and set the checkout date. The
checkout date should be set to the current date minus some random number of days.
Add a function to the Book prototype called "checkIn". The function will change the available property of the book from false to true. Add a function called isOverdue that checks the current date and the checked out date and if it's greater than 14 days it returns true
Patron Class Add a function to the Patron prototype called "read" that adds a book to it's books out property. Add a function to the Patron prototype called "return" that removes a book from it's books out property. C. Test Program Create 5 different books from the Book Class and store them in an array called catalog.
Create 5 different patrons from the Patron Class and store them in an array called patrons. Write a loop that simulates checkouts and checkins for a 3 month period. Every day iterate over the catalog, and every person in the patrons array. If the patron currently has the book checked out then check it in. If it is not checked out then add it to the patrons list of books via the patrons read method. If the book is overdue then add a fine of $5.00 to the patron returning it. At the end of the 3 month period, display each patron, the books they have currently checked out and any fine they may have.
This is what I have so far but I'm stuck. I can't get the loop to work and so far I'm able to use patron.booksOut.lenght to display the amount of books that the patron has checked out, but I would like to display the title. My isOverDue also doesn't work and I think it's because the way I have checkOut() coded. Can some one please help with that and explain why and how (with code) to accomplish that and I'll probably post comments after because I will have questions.
// Book class
class Book{ // Constructor that accepts titel, available, publicationDate, callNumber, authors constructor(title, available, publicationDate, callNumber, author){ this.title = title; this.available = available; this.publicationDate = publicationDate; this.callNumber = callNumber; this.author = author; this.checkoutDate = new Date(); }
// checkOut prototype checkOut(){ // Puts available to false this.available = false;
var temp = new Date(); temp.setDate(Math.floor(Math.random() * 25)); this.checkoutDate = temp; }
// checkIn prototype checkIn(){ // Sets the available variable to true after the book is checked in this.available = true; }
// isOverDue prototype isOverDue(){ // If the checkOutDate is greater than 14 than true will be returned charging the patron $5 if((this.checkOutDate - new Date())> 14){ return true; } // If not than false is returned return false; } }
// Author class class Author{ constructor(fName, lName){ // Adding the first and last name to this. object this.fName = fName; this.lName = lName; } };
// Patron class class Patron{ // Patron constructor using firstName, lastName, number, booksOut, and fine as arguments constructor(firstName, lastName, number, booksOut, fine){ this.firstName = firstName; this.lastName = lastName; this.cardNumber = number; this.booksOut = booksOut; this.fine = fine; }
// If a book is read than the book is pushed onto the booksOut arrau of the patron read(book){ this.booksOut.push(book);
} // If the patron returns the book than the book is removed from the booksOut of the patron returns(book){ this.booksOut.splice(this.booksOut.indexOf(book), 1); } };
// Creating two author objects var bob = new Author("Billy", "Bob"); var schwab = new Author("John", "Schwab"); // Adding these authors on to the authors array var authors = [bob, schwab];
// Creating five books var book1 = new Book("Hans und Peter", true, new Date(2004,05,02), 11, authors[1]); var book2 = new Book("Der Wilde Westen", true, new Date(2006,07,11), 22, authors[1]); var book3 = new Book("Peter Lustig", true, new Date(2009,11,25), 33, authors[1]); var book4 = new Book("The Secret arts", true, new Date(1984,01,16), 44, authors[0]); var book5 = new Book("Biography: Billy Bob", true, new Date(2010,09,03), 55, authors[0]); // Putting the books into the catalog array var catalog = [book1, book2, book3, book4, book5];
var patron1 = new Patron("Steven", "Doe",111, new Array(),0); var patron2 = new Patron("Ashton", "Doe",222, new Array(),0); var patron3 = new Patron("Justin", "Doe",333, new Array(),0); var patron4 = new Patron("Trystan", "Doe",444, new Array(),0); var patron5 = new Patron("Hugo", "Doe",555, new Array(),0); // Putting the patrons into the patrons array var patrons = [patron1, patron2, patron3, patron4, patron5];
// 90 days because in business 30 is considered one month var threeMonths = 90; /* Loop for 90 days each loop needs to check out a book to a patron and check it back in needs to check if it's over due and charge fine or not
for (i = 0; i < 90; i++){ // Random patron p = Math.floor(Math.random()*5); // Random book b = Math.floor(Math.random()*5); var currentPatron = patrons[p];
currentPatron.read(book[b]); currentPatron.checkOut(book[b]);
if (currentPatron.isOverDu){currentPatron.fine += 5;};
currentPatron.checkIn(book[b]); currentPatron.returns(book[b]);
} */ patron1.read(book3); book3.checkOut(book3);
console.log(patron1); console.log(" ");
var fine = patron1.fine; if(book3.isOverDue()){fine += 5;}
console.log(" ");
console.log(" Name Fine Books Out"); console.log(patron1.firstName + " " + patron1.lastName + " | $" + patron1.fine.toFixed(2) + " |\t " + patron1.booksOut.length); console.log(" ");
console.log(patron2.firstName + " " + patron2.lastName + " | $" + patron2.fine.toFixed(2) + " | " + patron2.booksOut.length); console.log(" ");
console.log(patron3.firstName + " " + patron3.lastName + " | $" + patron3.fine.toFixed(2) + " | " + patron3.booksOut.length); console.log(" ");
console.log(patron4.firstName + " " + patron4.lastName + "| $" +patron4.fine.toFixed(2) + " | " + patron4.booksOut.length); console.log(" ");
console.log(patron5.firstName + " " + patron5.lastName + " | $" + patron5.fine.toFixed(2) + " | " + patron5.booksOut.length);
Step by Step Solution
There are 3 Steps involved in it
Step: 1
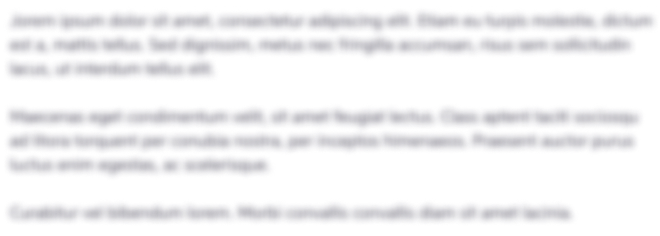
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started