Question
Just need a little help fixing a code. Everything runs fine for the most part, but there seems to be an issue when I use
Just need a little help fixing a code. Everything runs fine for the most part, but there seems to be an issue when I use the del command. It messes up the incrementing of the linked list. If you were to run the given code with the given input, you would see what I mean; the output does not match what it is supposed to be when using the prn command to print the current list. I have commented each function with what it should do.
The syntax for the insertAfter command is: ina num str
where ina is the name of the command(insertAfter), num is a positive integer, and str represents the text.
The syntax for the insertBefore command is: inb num str
The syntax for the deleteNode command is: del num
The syntax for the replaceText command is: rep num str
If entering the following commands:
inb 2 best
ina 5 is
inb 1 laughing
ina 3 is
del 4
del 3
rep 2 is
ina 5 medicine
inb 3 best
ina 1 best
ina 2 best
prn
end
The output should be:
inb 2 best
Text inserted at the beginning
ina 5 is
Text inserted at the end
inb 1 laughing
ok
ina 3 is
Such text exists already
del 4
No such index
del 3
Deleted
rep 2 is
Replaced
ina 5 medicine
Text inserted at the end
inb 3 best
Ok
ina 1 best
Such text exists already
ina 2 the
Ok
prn
1 laughing
2 is
3 the
4 best
5 medicine
end
Please comment where/what you fixed; I would like to learn.
The code to be worked on:
#include
if(cur!=NULL && cur->index == index) { struct Node* temp = cur; while(temp && strcmp(temp->text,text)!=0) temp = temp->next; if(temp && strcmp(temp->text,text) == 0) { printf("Such text exists already "); return; } } if(cur!=NULL && strcmp(cur->text,text) == 0) { printf("Such text exists already "); } else if(cur!=NULL && cur->index==index) { if(cur == *head) temp = newNode(1,text); else temp = newNode(index-1,text); if(prev==NULL) { temp->next = *head; *head = temp; } else { prev->next = temp; temp->next = cur; } cur = temp; while(cur) { int val = cur->index; cur = cur->next; if(cur) cur->index = val+1; } printf("Ok "); } else { temp = newNode(1,text); temp->next = *head; *head = temp; cur = temp; index=0; while(cur) { if(cur) cur->index = index+1; if(cur) cur = cur->next; } printf("Text inserted at the beginning "); } } //deleteNode function: //(a) If the list contains a node whose index is equal to the number specified in the command, then the node must be removed from the list, indexes of the list should be changed to keep increasing order, and the following message must be printed "Deleted". //(b) If the list does not contain a node whose index is equal to the number specified in the command, then the program must leave the list unchanged and the following message must be printed "No such index". void deleteNode(struct Node** head, int index) { struct Node* temp; if(*head == NULL) { printf("No Such index "); return; } struct Node *cur = *head , *prev = NULL; while(cur!=NULL && cur->index!=index) { prev = cur; cur = cur->next; } if(cur!=NULL && cur->index==index) { if(prev==NULL) { temp = *head; *head = (*head)->next; free(temp); } else { prev->next = cur->next; free(cur); } printf("Deleted "); } else printf("No Such Index "); } // replaceText function: //(a) If the list contains a node whose index is equal to the number specified in the command, then the node text must be replaced with the text specified in the command, and the following message must be printed "Replaced". //(b) If the list does not contain a node whose index is equal to the number specified in the command, then the program must leave the list unchanged, and the following message must be printed "No such index". void replaceText(struct Node* head, int index, char *text) { if(head==NULL) { printf("No Such Index "); return; } struct Node *cur = head; while(cur!=NULL && cur->index!=index) cur = cur->next; if(cur==NULL) { printf("No Such Index "); } else { free(cur->text); cur->text = (char*)malloc(sizeof(char)*(strlen(text+1))); strcpy(cur->text,text); printf("Replaced "); } } // function to print all the node's data of linkedlist void printList(struct Node* node) { if(node==NULL) { printf("List is Empty "); return; } while(node) { printf("%d\t\t%s ",node->index,node->text); node = node->next; } } // main method to test above functions int main() { struct Node* head = NULL; char cmd[4]; char name[256]; int index; while(1){ scanf("%s",&cmd); if(strcmp(cmd,"ina")=='\0') { scanf("%d %s",&index,&name); insertAfter(&head , index,name); } else if(strcmp(cmd,"inb")=='\0') { scanf("%d %s",&index,&name); insertBefore(&head,index,name); } else if(strcmp(cmd,"del")=='\0') { scanf("%d",&index); deleteNode(&head, index); } else if(strcmp(cmd,"rep")=='\0') { scanf("%d %s",&index,&name); replaceText(head,index,name); } else if(strcmp(cmd,"prn")=='\0') { printList(head); } else break; } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
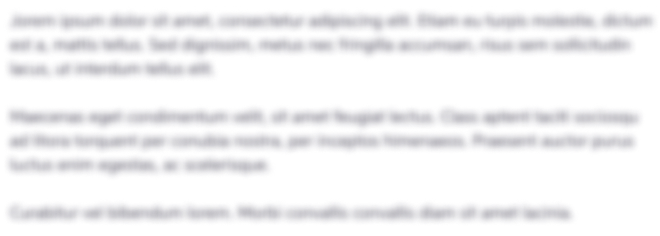
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started