Question
Lab 06 - Stonks In this lab, you will create a program that models a stock market based around the fictional cryptocurrency FazCoin. Step 1:
Lab 06 - Stonks
In this lab, you will create a program that models a stock market based around the fictional cryptocurrency FazCoin.
Step 1: Self-Explanation
Explain in your own words what is happening in go(). Put some comments above the different methods being called so that before you start coding, you know the big picture of how the game is going to work.
Step 2: Coding
Take a look at the javadoc. Implement all methods, constructors, and vairables listed for each class. Some have already been provided for you, some you will need to write yourself. Make sure to test your methods as you complete each one.
Step 3: Playtest
Now that you have completed the methods and tested them individuallly, it is time to playtest the program in its entirety.
StonkAppMain.java
public class StonkAppMain {
StonkMarket sm = new StonkMarket(); StonksAppView view = new StonksAppView(); Wallet w;
public void go(){ view.startScreen(); walletInitializer(); view.printMenu(); view.walletInfo(w, sm); String action = view.getAction("What would you like to do? "); while(!action.startsWith("x")) { parseAction(action); view.walletInfo(w, sm); action = view.getAction("What would you like to do? "); } }
public void walletInitializer(){ String walletChoice = view.getAction("Would you like to start with the default wallet, or enter your own? " + "Enter [d]efault or [c]ustom: "); if(walletChoice.startsWith("d")){ w = new Wallet(); } else{ int fazCoin = Integer.parseInt(view.getAction("So you want to invest more! " + "How much FazCoin do you already own? ")); double usd = Double.parseDouble(view.getAction("How much money in USD do you plan to work with? ")); w = new Wallet(fazCoin, usd); } }
public void parseAction(String action) { if (action.startsWith("b")) { int num = Integer.parseInt(view.getAction("How much FazCoin would you like to buy? ")); purchaseFazCoin(num); } else if (action.startsWith("s")) { int num = Integer.parseInt(view.getAction("How much FazCoin would you like to sell? ")); sellFazCoin(num); } else if (action.startsWith("n") || action.startsWith("d")) { //Don't do anything } else { System.out.println("Please enter a valid command."); } }
public void purchaseFazCoin(int numFazCoin){
}
public void sellFazCoin(int numFazCoin){
}
public static void main(String[] args) { StonkAppMain m = new StonkAppMain(); m.go(); }
}
StonkMarket.java
import java.util.Random;
public class StonkMarket {
private double exchangeRate = 1; private static final Random RANDY = new Random();
public double fazCoinToUSD(int fazCoin){ return (fazCoin * getExchangeRate()) / 100.0; }
}
StonkAppView.java
import java.util.Scanner;
public class StonksAppView {
//Placed as instance variable for memory and to make sure there aren't conflicts accessing System.in private final Scanner scanner = new Scanner(System.in);
public void startScreen(){ //Can add splashscreen System.out.println("Welcome to the stonk market."); }
public void printMenu() { System.out.println("Type \"X\" to exit at any time."); System.out.println("[S]ell stonks"); System.out.println("[B]uy stonks"); System.out.println("Do [N]othing"); System.out.println(); }
public String getAction() { return scanner.nextLine().toLowerCase(); }
public String getAction(String msg) { System.out.println(msg); return getAction(); }
public void walletInfo(Wallet w, StonkMarket sm){ System.out.printf("You currently own %d FazCoin, worth $%.2f USD.%n", w.getFazCoin(), sm.fazCoinToUSD(w.getFazCoin())); System.out.printf("Current exchange rate for FazCoin is %.2f USD per 100 FazCoin.%n", sm.changeExchangeRate()); System.out.printf("You have $%.2f in your wallet.%n", w.getUSDollars()); System.out.println(); }
}
Wallet.java
public class Wallet {
private int fazCoin; private double USDollars;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
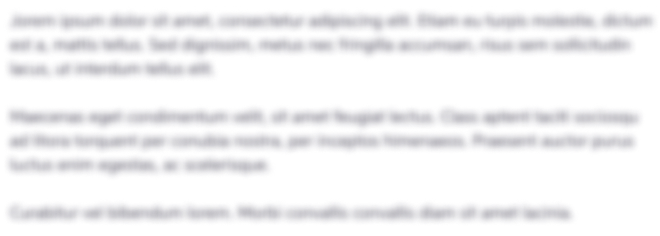
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started