Question
Lab 8: Binary Numbers The Binary Class Tasks The program should allow the user to convert decimal numbers to binary numbers as long as the
Lab 8: Binary Numbers
The Binary Class
Tasks
The program should allow the user to convert decimal numbers to binary numbers as long as the user desires. You should implement two methods: one for converting decimal numbers to binary numbers, and another for printing binary numubers. The binary numbers will be represented as int arrays. In addition, you should use the getInt method from p. 348 (3rd edition; or 338 for 2nd edition) of your textbook.
Your program should ask the user to enter a positive int. Continue asking the user until a positive int is entered. Use the book's getInt method rather than calling console.nextInt().
This int should then be converted to a binary number, storing the 0s and 1s in an array. Then the array should be printed starting from the last index to index 0.
Your program should have a while loop so that the user can continue to enter integers. Ask the user whether he or she wants to continue. Here is an example interaction (user input is underlined).
Enter a positive integer: foo
Not an integer; try again.
Enter a positive integer: -1
Not positive; try again.
Enter a positive integer: bar
Not an integer; try again.
Enter a positive integer: 0
Not positive; try again.
Enter a positive integer: 13
13 decimal is equal to 1101 binary
Do you want to continue (yes or no)? yes
Enter a positive integer: 42
42 decimal is equal to 101010 binary
Do you want to continue (yes or no)? no
You answered no. Have a nice day.
Details
Representing Binary Numbers as Arrays
Consider the binary number 111010. Your program will use an array to store the individual bits of this binary number in the following way.
index | 0 | 1 | 2 | 3 | 4 | 5 |
value | 0 | 1 | 0 | 1 | 1 | 1 |
Note that the bits are stored in reverse order. For example, the 0 bits in 111010 are stored at index 0 and index 2 in the array.
Method for Converting a Decimal Number to Binary
This method should have an integer parameter and return an integer array.
public static int[] convertToBinary(int decimal)
First, determine how many bits are needed, that is, the length of the array to store the bits. This can be done by a loop that repeatatly divides the parameter value by 2 until it reaches zero. However, we should make sure that the original value is saved for the next part of the process.
Save decimal in decimalCopy.
Initialize len to 0.
Loop until decimal is 0.
Divide decimal by 2.
Increment len.
Store decimalCopy in decimal.
Seccond, convert the integer value to a binary number based on the following pseudocode. It first creates a bit array of length len (see p. 427 for examples of creating arrays). Then index is initialized to 0. Similar to the previous loop, this loop also repeatedly divides decimal by 2 until it is 0. In this case, before each division, the body of the loop tests whether the int is odd or not. If it is odd, then a 1 is stored in the array at the index; else a 0 is stored in the array at the index. The array is the return value of the method.
Create an int array with length len.
Initialize index to 0.
Loop until decimal is zero:
If the value is odd: then store a 1 in the array at index, else store a 0 in the array at index,
Divide decimal by 2.
Increment index.
Return the array.
Method for Printing a Binary Array
This method should have an integer array parameter and return nothing. It should use System.out.print to print the values in the array.
public static void printBinaryArray(int[] binary)
To print a binary array, start at the last index (the array length minus one) and go backward to index 0. If the array is named foo, then the for loop would look like:
for (int i = foo.length - 1; i >= 0; i--) {
// do stuff with foo[i]
}
Do not print any spaces between the bits.
Rubric
Your program should compile without any errors. A program with more than one or two compile errors will likely get a zero for the whole assignment.
The following criteria will also be used to determine the grade for this assignment:
[2 points] If your submission includes the following:
The main method prints "Lab 8 written by [...]".
Your submission was a Zip file named lab8.zip containing a folder named lab8, which contains the other files.
If your Java program was in a file named Binary.java.
If the output of your Java program was in a file named BinaryOutput.java.
If your program contains a comment that describes what the program does and contains a comment describing each method.
If your program is indented properly.
[3 Points] For a correct implementation of the method that converts an integer (assumed to be positive) into an array of integers, in which each element is either 0 or 1, representing the value in binary.
[3 Points] For a correct implementation of the method that prints a binary array. The method should print from the last index of the array to the first.
[3 Points] For repeatedly prompting until the user enters a positive number.
[3 Points] For not crashing if the user enters a string instead of a number (use the getInt method from p. 338 of your textbook).
[3 Points] For a valid while loop in your main method that asks the user (yes or no) if they wish to continue. If the user enters "yes", the loop should continue.
[3 Points] For a main method that ties these all together to make a working program.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
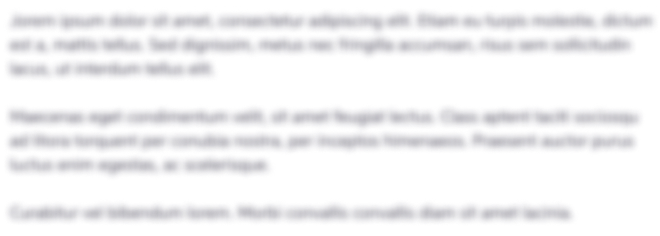
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started