Question
Lab Part 1 - IP Address Builder Tool Class This lab is loosely based on a common tool used in industry that many developers and
Lab Part 1 - IP Address Builder Tool Class
This lab is loosely based on a common tool used in industry that many developers and security professionals use or build themselves. This has application across the networking fields and the security field as well as the developer field.
Functionality:
The utility will be interactive with the user in that it will get information from the user and generate a list of IP Addresses between two given IP Addresses. This list will be saved out to a file that could later be used as input into an IP address scanner or tester or other security tool that might need a list of IP Addresses.
The utility should ask the user for two separate IP Addresses. The way it will ask is up to you whether you ask 1 octet at a time or all at once with dotted decimal notation. Each of those two methods will have ramifications on how you approach the rest of the utility. The output of the utility will be to a fixed name file in the same folder where the utility is running. We will not get fancy and have it where you can place the file anywhere and name it anything. A true utility would have that capability but not for this lab. The output will be a list of IP Addresses in dotted decimal notation (127.0.0.1 for example) with one address appearing on each line.
Programming Specifics:
You are to create a class in a file by itself so that the instructor when grading COULD import it into a program and use it. The class name should be StudentIPAddress. The class will have at least 1 instance variable (common to refer to these as properties in other Object Oriented Programming Languages) for each of the 4 octets in the IP address. Note in dotted decimal notation, there are 4 numbers and 3 periods. The 4 numbers are referred to as octets. Octet1 through Octet4. Remember the naming convention with the beginning underscore as mentioned in the text for instance variables.
The class will have the need to have a method called DottedDecimalNotation that returns the dotted decimal notation of the IP Address stored in the instance. The usage would look something like this: print(ClassVariable.DottedDecimalNoation()). The str(ClassVariable) should show the same dotted decimal notation as the method. You may find it useful to have the method and the __str__ linked in some fashion (okay blatant hint there).
The class should have a method in it called NextAddress that when used will increase the IP Address stored in the instance to the next address in line. This will automatically roll over to the next higher number should one of the octets max out at 255. If the IP Address is at the max of 255.255.255.255, it will roll over to the 256.256.256.256 invalid IP address. The method should return a Boolean True or False. It will be True if going to the next IP Address produced a valid IP Address and False if going to the next IP Address produces the 256.256.256.256 or any other invalid IP Address.
The class should smart enough to not try and build an invalid IPV4 address. Which means that each octet is limited to the numbers 0 to 255. So the address range is from 0.0.0.0 to 255.255.255.255. There is no such thing as a valid 756.523.195.463 IP Address. When the user tries to create an invalid address, the class will set ALL the octets to 256 thus producing the 256.256.256.256 IP Address.
A second method will then be created called ValidIPAddress that returns True or False. True if the IP address if valid and False if it is not. For the purposes of this lab, an IP Address will be considered valid if each of the 4 octets is between 0 and 255. This means that is you do a ValidIPAddress test on the 256.256.256.256 address it should return false. Use the boolean values of True and False, not the string of "true" or string of "false".
The last method that is required to be written will most likely be the hardest. It will require the input of a second IP address item. Yes, you will be dealing with 2 IP Address items at the same time. The method will be called IsSmaller. IsSmaller will return true if the IP Address of the instance is smaller then the IP Address of the instance passed into the method. The usage would look like this: if (classvariable.IsSmaller(otherclassvariable) == True): This means if the information in the classvariable instance is smaller than the information the otherclassvariable instance, a result of True would be returned and if not, False would be returned. Which can then be checked in an if or while statement. An IP Address will be considered smaller if the first IP Address comes before the second IP address. You will need to check each of the 4 octets for numeric order.
This little bit of logic should help you along your way, if the first ip address octet on the first address is smaller than the first ip address octet on the 2nd address, then the first address is smaller. If that first octet is larger on the first IP address than the 2nd IP address's first octet, then the first IP address is not smaller. If the first octet on each is equal, you have to move to the 2nd octet on each address and repeat the comparisons and do this all the way down to the fourth octet. In the fourth octet, if the two are equal, then the first address is NOT smaller than the second address.
Here are a couple examples:
127.0.0.1 is smaller than 129.36.26.3
127.0.0.1 is not smaller than 126.99.12.3
127.0.0.1 is not smaller than 126.255.255.255
127.0.0.1 is not smaller than 127.0.0.1
127.0.9.12 is smaller than 127.0.10.1
Be sure that all methods have the appropriate help features using whatever appropriate terms you wish in the help. That means set up the docstrings in the class.
Do not use any returns to break out of loops or leave function/methods early. Program all of these items to use boolean style controls (ie True and False) to exit loops and return from methods. Only a single return should be used in methods or functions you create for this lab. One way into and one way out of each function or method.
Lab Part 2 - Use the Class you Built
Now that you have written the class, you will use it in your own python program. Notice the class you build did not have any input or output to a file or asking the user to enter an IP address. That is to be done in this program portion of the lab. This program should ask the user to enter the IP address from which to start from and the IP address which to stop at. Use the Start address and create an instance of your class and a 2nd instance for the ending address. Then loop through the addresses from start to finish using the NextAddress method and the IsSmaller method in controlling that loop. Make sure the user does not get to make an invalid IP Address so you will need to filter the data they enter. The program is to loop until they enter valid start and finish IP addresses. Do not start making any list items until they have entered valid IP addresses. Each address you create that is valid needs to be written into a file. The name of the file will be fixed at LabIPAddresses.txt. Each address is to appear in that file on a separate line.
A couple of other User Interface items that need to be in the program. If they entered nothing for an octet in the first IP address, it assumes the minimum number 0. If they enter nothing for an octet in the ending IP address, it assumes the maximum 255. If they do not enter a beginning address, it will assume 0.0.0.0 and if they do not enter an ending address, it will assume 255.255.255.255. It may be easier for you to program entering each of the 4 octets individually so you do not have to split apart a string to pull the numbers that way.
If you allow them to enter the IP Address as a single string like 0.0.34.5 then you will have to also be able to handle if they enter something like 34.5 or ..34.5 or .34.5, etc. That parsing while possible will be difficult to do.
A second user interface item to be included is some kind of progress notation for the user to watch as the program progresses. While the output is going to the file, something needs to appear on the screen to let the user know how the process is progressing. The progress needs to identify that the process has started and that it has ended with messages to the user. It also needs to display some progress message along the way. You are not to print something on the screen for each IP Address created though. Have some reasonable point along the way for it to show progress. This could be a message ever 100 or 1000 addresses written. If you want a MAJOR challenge (on your own of course) you could write code to calculate the number of IP Addresses and write an update message ever 5 percent. That is not assigned but would make for a good practice for you outside of the class.
Here are some items that are not to be done or seen in this program. First off, all of this has to be done in your code. You cannot find some "IP Address" tool kit out on the web and utilize it. You have to write all this code. This also means no native Python IP Address tools can be used either. As the GUI, is somewhat problematic at times, no GUI Interface for this program either. This tool can easily be done with some for loops without any use of creating a class. You are not to go down that route. The class is created, it is to be used in this program with the methods to be used for controlling the loops.
Do you legwork up front and write out the logic. It will help you as you go along. You may choose to write it as a single program and then move it into the class if you want but that may take longer in the long run. Test incrementally as you go along instead of tying to get it all programmed and then test. Do not wait until the last hour it is due before starting.
Testing? Do not forget to test and capture screen shots of your testing. Show the program interface and the progress for at least 3 ranges. For one range go about 250 or so addresses. For a second range, do 1,000 addresses and the final range do a small range of 1. You can do more tests but these three are required to be shown in the submission document. It is highly advised that you do not try and 0.0.0.0 to 255.255.255.255 range. While it covers everything, it will create a significantly LARGE file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
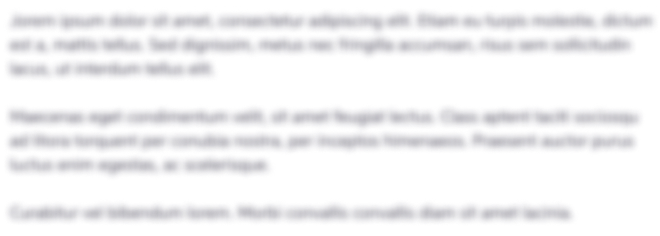
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started