Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Langauge: Java Instructions: Complete these steps: Set up a project in your preferred development environment using the provided Java sources. Complete the various TODO items
Langauge: Java
Instructions:
Complete these steps:
- Set up a project in your preferred development environment using the provided Java sources.
- Complete the various TODO items to get your output to match the provided sample.
- Create two more concrete animal classes and add two or three instances of them to the zoo.
- Paste the updated output into file output.txt and include in the project root folder
Code:
AbstractAnimal.java
public abstract class AbstractAnimal implements Animal { private double weight; private final String name; public AbstractAnimal(final String name, final double weight) { // TODO initialize the instance variables - very important } public String getName() { return name; } public double getWeight() { return weight; } public abstract double getDailyFoodPercentage(); public void doDailyFeeding() { // TODO increase weight according to daily % } public void doDailyExercise() { // TODO decrease weight according to daily % } }
Animal.java
public interface Animal { String getName(); double getWeight(); double getDailyFoodPercentage(); void doDailyFeeding(); void doDailyExercise(); }
Elephant.java
public class Elephant extends AbstractAnimal { public Elephant(final String name, final double weight) { // TODO your job } @Override public double getDailyFoodPercentage() { return 0.1; } // TODO override toString so that the elephant reports its name (as in the output) }
output.txt
Roll call This is Elephant Huey weighing 505.2 kg This is Elephant Dewey weighing 612.9 kg Ready for feeding Today we used 111.81 kg of food Ready to check weight This is Elephant Huey weighing 555.72 kg This is Elephant Dewey weighing 674.19 kg Ready to work out Ready to check weight This is Elephant Huey weighing 505.2 kg This is Elephant Dewey weighing 612.9 kg
Zoo.java
public class Zoo { public static void main(final String[] args) { // create animal instances final Animal[] zoo = new Animal[] { new Elephant("Huey", 505.2), new Elephant("Dewey", 612.9) // TODO define two more animal classes // TODO create instances thereof here }; System.out.println("Roll call"); for (int i = 0; i < zoo.length; i ++) { final Animal current = zoo[i]; System.out.println("This is " + current + " weighing " + current.getWeight() + " kg"); } System.out.println("Ready for feeding"); double food = 0; for (int i = 0; i < zoo.length; i ++) { final Animal current = zoo[i]; food += current.getDailyFoodPercentage() * current.getWeight(); current.doDailyFeeding(); } System.out.println("Today we used " + food + " kg of food"); // TODO check weight again // TODO exercise // TODO check weight one last time } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
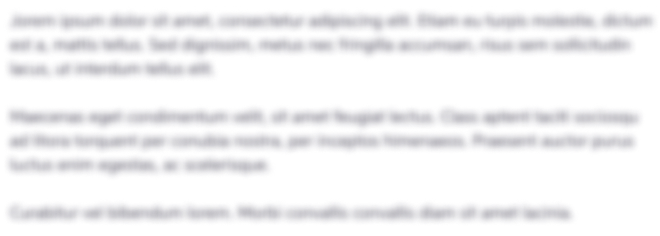
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started