Question
Language C++ I need help completing this. I already have hw8.cpp file. I only need MyVector.cpp and MyVector.h. The instructions are after hw8.cpp... hw8.cpp: #include
Language C++
I need help completing this. I already have hw8.cpp file. I only need MyVector.cpp and MyVector.h. The instructions are after hw8.cpp...
hw8.cpp:
#include // Bring the whole implementation file into this file. Gets around the issues // with seperate compilation and template classes while still allow the use // of multiple files #include "MyVector.h" using namespace std;
template void subMenu(MyVector &list) { T elem; int sz, sel; bool badIndex = true;
do { cout << endl; cout << " MyVector Sub-Menu "; cout << "======================================================================== "; cout << "1. Print the capacity and size of the list "; cout << "2. Clear all the elements on the list "; cout << "3. Add an element to the end of the list "; cout << "4. Remove the last element from the list and print its value "; cout << "5. Use the index operator to set and print the value of an element "; cout << "6. Use the reserve function to change the number of elements allocated "; cout << "7. Use the resize function to change the number of elements in use "; cout << "8. Search for a given element in the list "; cout << "9. Print the current contents of the list "; cout << "0. Return to the main menu "; cout << "======================================================================== "; cout << "Enter selection: "; cin >> sel; // If you use integer selections, you should have the following to prevent // your code from going into an infinite loop if they happen to enter a // letter instead of a digit while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "The selection must be an integer. Enter selection: "; cin >> sel; } cout << endl;
switch(sel) { case 0: // Return to main menu. Do nothing but print a new line. cout << endl; break;
case 1: // Print the capacity and size of the list if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
cout << "Capacity: " << list.capacity() << endl; cout << "Size: " << list.size() << endl; break;
case 2: // Clear all the elements in the list if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
list.clear(); cout << "The list has been cleared. "; break;
case 3: // Add an element using push_back cout << "Please enter an element to add: "; cin >> elem; while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "Invalid element entered. Please enter a new element: "; cin >> elem; }
// push_back can trigger an OutOfMemory error, so have it in a try block try { list.push_back(elem); } catch(OutOfMemory) { cout << "Unable to allocate space for " << elem; cout << ". Returning to main menu. "; return; // exits out of subMenu function }
cout << elem << " added to the back of the list. "; break;
case 4: // Delete the last element from the list if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
cout << list.pop_back() << " was deleted from the list. "; break; case 5: // Use the index operator to set/change an element if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
// Two errors may occur. They may give us a bad index or they may give // us a non-integer. The badIndex bool flag will check for the first // while cin.fail() checks for a non-integer. while(badIndex) { cout << "The valid indices are 0 to " << (list.size() - 1) << endl; cout << "Enter an index to access: "; cin >> sz; if(cin.fail()) { // Got a non-integer cin.clear(); cin.ignore(20, ' '); cout << "You must enter an integer only! "; } else { // Got an integer, try and see if it is good. try { cout << list[sz]; cout << " is the current value for index " << sz << endl; badIndex = false; // exit the loop } catch(InvalidIndex) { cout << "The index " << sz << " is invalid! "; } } } // Now we have a good index and we've printed the old value to the // screen. We can now update it without worrying about a try block. // Also, reset badIndex back to true so the next time they select // option 5, it will work badIndex = true; // Go into index loop on next Option 5 selection cout << "Enter the new value for index " << sz << ": "; cin >> elem; // Check for input failure and ask again while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "Invalid value. Please re-enter a new value: "; cin >> elem; } // Use the index operator to update the value list[sz] = elem; cout << "Index " << sz << " is now " << list[sz] << endl;
break;
case 6: // Call the reserve function. Check for OutOfMemory exceptions cout << "How many elements do you want in the list? "; cin >> sz; while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "You must enter an integer! "; cout << "How many elements do you want in the list? "; cin >> sz; }
try { list.reserve(sz); } catch(OutOfMemory) { cout << "There is not enough memory to reserve " << sz; cout << " elements! Returning to main menu... "; return; }
cout << "The list's capacity is now " << list.capacity() << endl; break;
case 7: // Call the resize function. Check for OutOfMemory exceptions cout << "What is the new size of the list? "; cin >> sz; while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "You must enter an integer! "; cout << "What is the new size of the list? "; cin >> sz; }
try { list.resize(sz); } catch(OutOfMemory) { cout << "There is not enough memory to reserve " << sz; cout << " elements! Returning to main menu... "; return; }
cout << "The list's size is now " << list.size() << endl; break;
case 8: // Search for a given element in the list if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
cout << "What value do you wish to search for? "; cin >> elem; // Check for input failure and ask again while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "Invalid value. Please re-enter a new value: "; cin >> elem; }
// Call the find function and store its results in sz sz = list.find(elem); // Compare sz to -1 if(sz == -1) { cout << elem << " was not found in the list. "; } else { cout << elem << " was found at index " << sz << endl; } break;
case 9: // Output the list contents if(list.empty()) { cout << "The list is empty. Please use one of the other options. "; break; }
cout << "The list contains: " << list << endl; break;
default: cout << "That is an invalid selection! "; } }while(sel != 0); }
int main() { int sel; MyVector iList; MyVector dList; MyVector cList;
do { cout << endl; cout << " Welcome to the CS2020 MyVector Menu "; cout << "============================================== "; cout << "1. Test the MyVector class for integers "; cout << "2. Test the MyVector class for doubles "; cout << "3. Test the MyVector class for characters "; cout << "0. Exit the program "; cout << "============================================== "; cout << "Enter selection: "; cin >> sel; // If you use integer selections, you should have the following to prevent // your code from going into an infinite loop if they happen to enter a // letter instead of a digit while(cin.fail()) { cin.clear(); cin.ignore(20, ' '); cout << "The selection must be an integer. Enter selection: "; cin >> sel; }
switch(sel) { case 0: cout << "Good bye! "; break; case 1: // Call the subMenu with an integer subMenu(iList); break; case 2: // Call the subMenu with a double subMenu(dList); break; case 3: // Call the subMenu with a character subMenu(cList); break; default: cout << "That is an invalid selection! "; } }while(sel != 0);
return(0); }
================================================================================================================
Below are the instructions my teacher provided us:
Template Classes and Exceptions - MyVector The purpose of this asssignment is to create a template class for a dynamically sized array. This will be similar to the C++ vector class from the standard template library (STL).
You will create three files in total: MyVector.h, MyVector.cpp, and hw8.cpp.
You will be creating a template class called MyVector. This class will support a partially filled dynamic array of any datatype. Since it is a partially filled dynmic array, it will have three member variables: a pointer for the array (template pointer), an integer for the current count of elements, and an iteger for the array's capacity. The capacity will represent the total amount of allocated index the array may contain. The count will represent the current amount of elements within the array and the value will always be less than or equal to the capacity.
In addition, you will also create two empty exception classes:
OutOfMemory - If any memory allocation fails (caught a bad_alloc), throw an OutOfMemory object. Rethrow this exception to main if a member function catches this exception InvalidIndex - If an index less than 0 or greater than count-1 is given to the index operator, the InvalidIndex exception will be thrown. Note, the index operator should check count, not capacity.
MyVector constructors and destructor
Default constructor - Create an empty array that has no memory allocated to it. Zero out count and capacity. Constructor that takes an integer - The integer is the requested number of elements for the array. Allocate the requested number of elements to the array and set BOTH the count and the capacity to this integer. Initialize all elements in the allocated array to 0. Use the class's resize member function to handle memory allocation. Be sure to rethrow the OutOfMemory exception if caught when attempting to allocate. Constructor that takes an array and an integer - The array passed to the constructor will be the source of data for the MyVector array. The integer passed to the constructor will be both the count and the capacity of this MyVector object. Allocate the requested number of elements and then copy the source data from the passed array to this object's array. Use the class's reserve member function to handle memory allocation. Be sure to rethrow the OutOfMemory exception if caught when attempting to allocate. Copy constructor - Copy the capacity and count from the source object to the current object. Allocate the requested number of elements. Then copy the elements from the source object to the current object. Use the class's reserve member function to handle memory allocation. Use the class's reserve member function to handle memory allocation. Be sure to rethrow the OutOfMemory exception if caught when attempting to allocate. Destructor - If there is memory allocated to the current object, deallocate the memory using the delete command. operators
= (assignment) - Takes a source MyVector object by reference. Check if the source object is the current object ( A = A;) and do nothing if that is the case and return. It deletes any elements currently allocated the current object. It then reallocates based on the capacity of the source object and copies all of the source object's data over to the current object. Use the class's reserve member function to handle memory allocation. Be sure to rethrow the OutOfMemory exception if caught when attempting to allocate. << (output) - This will print all the in-use elements (as indicated by count) to the given output stream. [] (index) - This take an integer for the requested index and validate that the index is in use (as indicated by count). If so, it will return a reference to the element at that index (so it will be writable). If not, it will throw an InvalidIndex exception. public member functions
int capacity() - Returns the current capacity of the object. int size() - Returns the current number of elements in-use for the object, e.g. the count. bool empty() - Returns true if the object is empty; false otherwise. void clear() - Sets the value of all elements to 0. Do NOT deallocate the array. void reserve(int) - The given integer is the requested capacity of the current object. If the requested capacity is greater than the current capacity of the object, reallocate the array and then set capacity to the new value. Set all the newly allocated elements to 0 but do NOT overwrite any of the existing elements. Also, do NOT affect count in this function. This is purely to allocate more capacity to the object. During allocation, if a bad_alloc is caught, throw an OutOfMemory exception. void resize(int) - The given integer is the requested count for the current object. All elements between the requested count and the current count will be set to 0. The count will be affected in the following fashion. If the requested count is less than the current count (shrink), reset count to the requested count and do NOT affect capacity. If the requested count is greater than the current count and less than or equal to the current capacity, reset count to the requested value and do NOT affect capacity. If the requested count is greater than the capacity (grow), reallocate the array to the requested count, then set both count and capacity to the requested count. Only reset capacity when the requested count is greater than the current capacity. During allocation, if a bad_alloc is caught, throw an OutOfMemory exception.
void push_back(element) - Add an element to the end of the array. Increment count. If there is no space on the array for the new element, reallocate the array to add 10 more spaces (e.g. to capacity + 10), then add the element. Don't update capacity unless you need to reallocate.
element pop_back() - Remove the last element from the array. Decrement count and return the value of the last element. In the array, the value of the last element will be changed to 0 (e.g. if the MyVector is currently 5 2 9 8, pop_back would return 8 and the MyVector would be 5 2 9 0). Don't affect capacity in this function. int find(element) - Search the array and see if any of its elements match the given element. If a match is found, return the index at which the match was found. If no match is found, return -1 (since this is an invalid index, it serves as a sentinel for the "not found" condition). Only search the in-use elements (as indicated by count).
Main function
Use the following menu program for your main function. This is a nested menu which means you will have two loops. The outer loop will print the main menu while the inner loop will print the sub-menu that was selected in the outer loop. When the program is started, the main menu is presented. The sub-menu will only be shown when the user selects that sub-menu off the main menu.
To implement the nested menus, have the main menu in the main() function. Each option in the main menu will call another function which will display and process the sub-menu. The main menu appears as follows:
Welcome to the CS2020 Homework 8 Menu ============================================== 1. Test the MyVector class for integers 2. Test the MyVector class for doubles 3. Test the MyVector class for characters
0. Exit the program ==============================================
The only difference in the sub-menus is what datatype they are processing. Implement the sub-menus as a single, global template function that takes a single template dummy parameter. The purpose of this dummy parameter is to instantiate the function as either the integer sub-menu, the double sub-menu or the character sub-menu. In this sub-menu function, declare a MyVector object of the templated datatype. You may need additional template or normal variables to support the menu operations. Present the following menu to the user to manipulate the MyVector object:
MyVector Sub-Menu ======================================================================== 1. Print the capacity and size of the list 2. Clear all the elements on the list 3. Add an element to the end of the list 4. Remove the last element from the list and print its value 5. Use the index operator to set and print the value of an element 6. Use the reserve function to change the number of elements allocated 7. Use the resize function to change the number of elements in use 8. Search for a given index in the list 9. Print the current contents of the list
0. Return to the main menu ========================================================================
Each menu option is testing a member function or operator in the MyVector class. Option 1 will print the results of capacity() and size(). Option 2 will call the clear() function. Option 3 will call the push_back() function. Option 4 calls the pop_back() function. Option 5 uses the index operator. Option 6 uses the reserve() function. Option 7 uses the resize() function. Option 8 uses the find() function. Option 9 uses the output operator.
When the user gives option 0, return back to the main menu by exitting this function. This will switch back to main()'s scope. As long as your main menu loop is coded correctly, this should trigger the main menu being printed again so the user can test another MyVector object.
For options 1, 2, 4, 8 and 9, if the list is currently empty (empty() returns true), print out an error message about the list being empty so the operation could not be completed. Otherwise, perform the indicated operation.
For options 3, 6 and 7, be sure to use a try/catch block to check for the OutOfMemory exception being thrown. If there is an allocation failure, do NOT exit the program. Instead, return back to the main menu by exitting the sub-menu function.
For option 3, prompt the user for the new element and then use the push_back() function to add the element to the list.
For option 5, use a try/catch block to check for an invalid index being given. You can use the logic in main() for Lab 8 as inspiration for how to implement this option. Once the user gives a valid index, print the current value of the element at that index to the screen then ask the user for the new value of the element at that index. Read in the user's response and set the element at that index to the new value.
For option 6, prompt the user for the new capacity of the MyVector and then pass that to the reserve() function. For option 7, prompt the user for the new count of the MyVector and then pass that to the resize() function.
For option 8, prompt the user for the element they wish to search for. Use the find() function in the MyVector object. If find returns -1, tell the user that the element was not found. Otherwise, tell the user that the element was found at the returned index.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
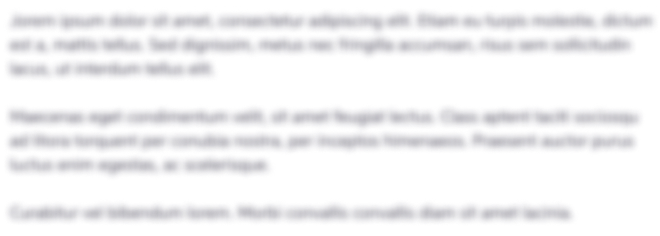
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started