Question
Language: C++ In this project, you are requested to implement queue by using a linked list (not an array). Implement a template class Queue as
Language: C++
In this project, you are requested to implement queue by using a linked list (not an array).
Implement a template class Queue as defined by the following skeleton:
template
class Queue
{
private:
NodeType
NodeType
public:
Queue( ); // default constructor: Queue is created and empty
Queue(const Queue
// for a deep copy
void MakeEmpty(); // Queue is made empty; you should deallocate all
// the nodes of the linked list
bool IsEmpty( ); // test if the queue is empty
bool IsFull( ); // test if the queue is full; assume MAXITEM=5
int length( ); // return the number of elements in the queue
void Print( ); // print the value of all elements in the queue in the sequence
// from the front to rear
void Enqueue(ItemType x); // insert x to the rear of the queue
// Precondition: the queue is not full
void Dequeue(ItemType &x); // delete the element from the front of the queue
// Precondition: the queue is not empty
~Queue(); // Destructor: memory for the dynamic array needs to be deallocated
};
In you main( ) routine, you need to test your class in the following cases:
Queue
int x;
IntQueue.MakeEmpty();
IntQueue.Dequeue(x);
IntQueue.Enqueue(10);
IntQueue.Enqueue(20);
IntQueue.Enqueue(30);
IntQueue.Enqueue(40);
cout << "int length 3 = " << IntQueue.length() << endl;
IntQueue.Dequeue(x);
cout << "int length 4 = " << IntQueue.length() << endl;
cout << The int queue contains: << endl;
IntQueue.Print();
if(IntQueue.IsFull() == false)
cout << The int queue is not full ! << endl;
else
cout << The int queue is full ! << endl;
Queue
float y;
FloatQueue.MakeEmpty();
FloatQueue.Dequeue(y);
FloatQueue.Enqueue(7.1);
cout << "float length 3 = " << FloatQueue.length() << endl;
FloatQueue.Enqueue(2.3);
cout << "float length 4 = " << FloatQueue.length() << endl;
FloatQueue.Enqueue(3.1);
FloatQueue.Dequeue(y);
cout << The float queue contains: << endl;
FloatQueue.Print();
Queue
cout << The float queue 2 contains: << endl;
FloatQueue2.Print();
FloatQueue.MakeEmpty();
cout << The float queue 3 contains: << endl;
FloatQueue2.Print();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
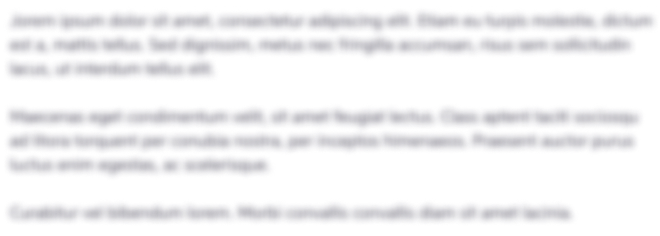
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started