Question
Language = C++ Task 1: Linked List Template Class Your first task is to replace the various Linked List collection classes in the code with
Language = C++
Task 1: Linked List Template Class
Your first task is to replace the various Linked List collection classes in the code with a single LinkedList? Template class that will be able to store any objects. You will create a LinkedList.h file that contains the class definition as well as the function definitions. Once implemented this template will be used in place of the CustomerList and VehicleList? class (storing Customer and Vehicle pointers, respectively). Your LinkedList template must be consistent with the previously used classes (ie. must implement the same member functions and override the same operators). There is one function in the CustomerList class that we will not provide in our generic template, the get? function. The only place this function is called from is the Shop? class. Modify the getCustomer? function in the Shop? class that calls this get? function so the functionality is preserved (ie. use the provided functions and operators provided by the LinkedList? template to provide this functionality).
---------------------------------CustomerList.cc-------------------------------------------------
#include
#include "CustomerList.h"
CustomerList::CustomerList() : head(0) { }
CustomerList::~CustomerList() { Node *currNode, *nextNode;
currNode = head;
while (currNode != 0) { nextNode = currNode->next; delete currNode->data; delete currNode; currNode = nextNode; } }
CustomerList& CustomerList::operator+=(Customer* newCust) { Node *currNode, *prevNode; Node* newNode = new Node; newNode->data = newCust; newNode->next = 0;
currNode = head; prevNode = 0;
while (currNode != 0) { if (*(newNode->data) < *(currNode->data)) break; prevNode = currNode; currNode = currNode->next; }
if (prevNode == 0) { head = newNode; } else { prevNode->next = newNode; }
newNode->next = currNode;
return *this; }
CustomerList& CustomerList::operator-=(Customer* cust) { Node *currNode, *prevNode;
currNode = head; prevNode = 0;
while (currNode != 0) { if (currNode->data == cust) break; prevNode = currNode; currNode = currNode->next; }
if (currNode == 0) return *this;
if (prevNode == 0) { head = currNode->next; } else { prevNode->next = currNode->next; }
delete currNode;
return *this; }
Customer* CustomerList::get(int id) { Node *currNode = head;
while (currNode != 0) { if(currNode->data->getId() == id) { return currNode->data; } currNode = currNode->next; }
return 0; }
int CustomerList::getSize() const { Node *currNode = head; int size = 0;
while (currNode != 0) { currNode = currNode->next; size++; } return size; }
Customer* CustomerList::operator[](int i) { if(i < 0 || i > (getSize()-1)) { return 0; } else {
Node *currNode = head;
for (int j = 0; j < i; j++) { currNode = currNode->next; }
return currNode->data; } }
//void CustomerList::toString(string& outStr) ostream& operator<<(ostream& output, CustomerList& cl) { for (int i = 0; i < cl.getSize(); i++) { output << (*(cl[i])); } return output;
}---------------------------------CustomerList.h-------------------------------------------------
#ifndef CUSTOMERLIST_H #define CUSTOMERLIST_H
#include "Customer.h"
class CustomerList { friend ostream& operator<<(ostream&, CustomerList&);
class Node { friend class CustomerList; private: Customer* data; Node* next; };
public: CustomerList(); ~CustomerList(); //void add(Customer*); //void toString(string&); int getSize() const; Customer* get(int); CustomerList& operator+=(Customer*); CustomerList& operator-=(Customer*); Customer* operator[](int);
private: Node* head; };
---------------------------------VehicleList.cc-------------------------------------------------
#include
#include "VehicleList.h"
VehicleList::VehicleList() : head(0) { }
VehicleList::~VehicleList() { Node *currNode, *nextNode;
currNode = head;
while (currNode != 0) { nextNode = currNode->next; delete currNode->data; delete currNode; currNode = nextNode; } }
//void VehicleList::add(Vehicle* newVeh) VehicleList& VehicleList::operator+=(Vehicle* newVeh) { Node *currNode, *prevNode; Node* newNode = new Node; newNode->data = newVeh; newNode->next = 0;
currNode = head; prevNode = 0;
while (currNode != 0) { if (*(newNode->data) > *(currNode->data)) break; prevNode = currNode; currNode = currNode->next; }
if (prevNode == 0) { head = newNode; } else { prevNode->next = newNode; }
newNode->next = currNode; }
VehicleList& VehicleList::operator-=(Vehicle* v) { Node *currNode, *prevNode;
currNode = head; prevNode = 0;
while (currNode != 0) { if (currNode->data == v) break; prevNode = currNode; currNode = currNode->next; }
if (currNode == 0) return *this;
if (prevNode == 0) { head = currNode->next; } else { prevNode->next = currNode->next; }
delete currNode;
return *this; }
int VehicleList::getSize() const { Node *currNode = head; int size = 0;
while (currNode != 0) { currNode = currNode->next; size++; } return size; }
Vehicle* VehicleList::operator[](int i) { if(i < 0 || i > (getSize()-1)) { return 0; } else {
Node *currNode = head;
for (int j = 0; j < i; j++) { currNode = currNode->next; }
return currNode->data; } }
//void VehicleList::toString(string& outStr) ostream& operator<<(ostream& output, VehicleList& vl) { for (int i = 0; i < vl.getSize(); i++) { output << (*(vl[i])); } return output;
}
---------------------------------VehicleList.h-------------------------------------------------
#ifndef VEHICLELIST_H #define VEHICLELIST_H
#include "Vehicle.h"
class VehicleList { friend ostream& operator<<(ostream&, VehicleList&);
class Node { friend class VehicleList; private: Vehicle* data; Node* next; };
public: VehicleList(); ~VehicleList(); //void add(Vehicle*); //void toString(string&); int getSize() const; VehicleList& operator+=(Vehicle*); VehicleList& operator-=(Vehicle*); Vehicle* operator[](int);
private: Node* head; };
#endif
----------------------------------------LinkedList.h---------------------------------------------
//add here
Step by Step Solution
There are 3 Steps involved in it
Step: 1
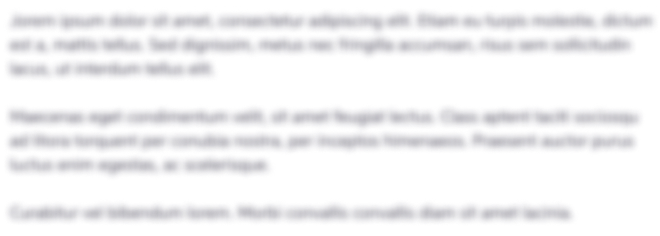
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started