Question
Language: C++ Write a class Rational to represent rational numbers. (a) Your class should have private member variables for the numerator and denominator. (b) Your
Language: C++
Write a class Rational to represent rational numbers.
(a) Your class should have private member variables for the numerator and denominator.
(b) Your class should have two constructors; one that takes no arguments, and one that takes two arguments.
(c) Write a Getter (Accessor) and Setter (Mutator) for each of the member variables. Perform error checking for denominator Setter.
(d) Add a public member function to your class:
void Print();
If numerator is 5 and denominator is 8, it should print 5/8".
(e) Add a public member function to your class:
void Reduce();
A member function that puts the rational into its most reduced form. For instance, it would change 24/36 to 2/3.
(f) Implement += as a member function (you are NOT required to reduce your fraction).
Rational& operator +=(const Rational& rhs);
Modifies the Rational it is called on to add rhs.
(g) Implement * operator as a non-member function. You are NOT required to reduce your fraction.
Rational operator *(const Rational& lhs, const Rational& rhs);
Returns a new Rational that is the product of the two Rationals passed in.
(h) Implement + operator as a non-member function. You are NOT required to reduce your fraction.
Rational operator +(const Rational& lhs, const Rational& rhs);
Returns a new Rational that is the sum of the two Rationals passed in.
(i) Implement == operator as a non-member function.
bool operator ==(const Rational& lhs, const Rational& rhs);
Returns true if a==b and false if not. Note; if a is 6/8 and b is 3/4, it should return true.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
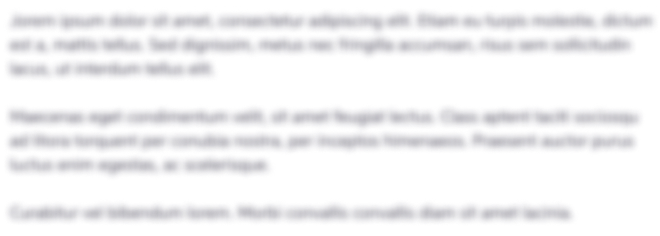
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started