Question
Language in C++ Objective: Design a program that uses two-dimensional arrays, classes and objects Assignment: Design a program to play the LoShuSquare (Links to an
Language in C++
Objective:
Design a program that uses two-dimensional arrays, classes and objects
Assignment:
Design a program to play the LoShuSquare (Links to an external site.) Game.
Define a class LoShuSquare with the necessary data and functions to play the game. Use a 2-D int array with three rows and three columns as the game grid. This will be a member variable in your class. Even though it is a 3x3 array, you should define a constant for the size of the square and use it when defining the array and when referring to the size in related functions. The class should also have the following functions:
- A constructor to initialize the grid with zeros.
- A function to fill a specific square with a number 1-9, given row and column.
- For example, fill (0, 0, 4) will change the upper leftmost square to 4. Make sure the function only accepts numbers 1-9. Also, the numbers on the square must be unique (no repetitions allowed). Please note that if you allow repetitions, a matrix filled out with the same number may turn out to be considered a Lo Shu Square even though it is not.
- A function to print the board on the screen. This doesn't have to be fancy, but I must be able to recognize a square with 9 numbers on the screen.
- A function that returns true if the grid is a Lo Shu Square. This function should call individual private functions that check for rows, columns and diagonals separately.
- The main function that tests your game.
Have fun!
Testing/Output:
Test your program with numbers that form a Lo Shu square and numbers that don't. Run a test with all numbers being the same, like all 1's. Take a screenshot of the output of your program and upload it with your source code.
Turn in:
- LoShuSquare.h (or .hpp) and LoShuSquare.cpp with the implementation of your class, main.cpp. A screenshot of the rounds of the game ending in a success and one ending a a failure.
Please add headers explaining the function used, and attach a file of the screenshots!
Thank you!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
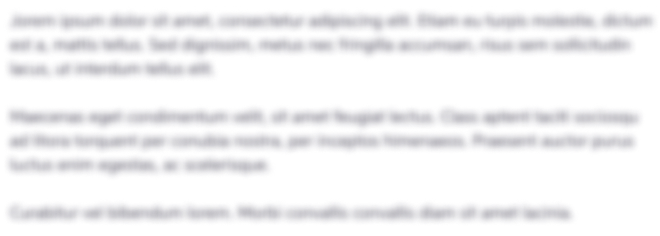
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started