Language is C++
I have to create a Magic Square Solver based on code I have already created and I'm not sure what to do since my code is incomplete. Could you modify my code so that it works with the assignment and teach me what to do? I would appreciate it if you explained each step of the process and used comments to explain what is going on; so that I can learn what the code is doing. Please read the assignment and don't skip over anything. I will happily upvote if your expertise really helps me.
MY CODE:
#include #include #include #include using namespace std;
const int MAX_SIZE = 100;
char puzzle[MAX_SIZE][MAX_SIZE]; int puzzle_int[MAX_SIZE][MAX_SIZE];
bool isCompletePuzzle(int** puzzle, int size) {
for (int i = 0; i
bool isValidPuzzle(int** puzzle, int size) {
}
bool solvePuzzle() {
}
int valueOf(char ch) { if (isupper(ch)) { // Check and convert uppercase characters to lowercase to get correct value. ch = tolower(ch); } if ((ch >= '0') && (ch
vector toDigits(string a) { vector result(a.length()); for (unsigned int i = 0; i void displayDecimalDigits(vector& a) { //Function to display decimal digits for (unsigned int i = 0; i int main() { string filename;
// Prompt user for filename cout > filename;
// Open file ifstream infile; infile.open(filename.c_str()); if (!infile) { cout
char ch; int sum = 0; int charcount = 0; while (infile.get(ch)) { if (isalnum(ch) || (ispunct(ch))) { charcount++; if (isalnum(ch)) { cout
// Read in puzzle infile >> charcount;; for (int i = 0; i > puzzle[i][j]; cout
// Check if number of entries is a perfect square int root = sqrt(charcount); if (root * root != charcount) { cout
// Display puzzle as integers cout
return 0; } }
ASSIGNMENT:
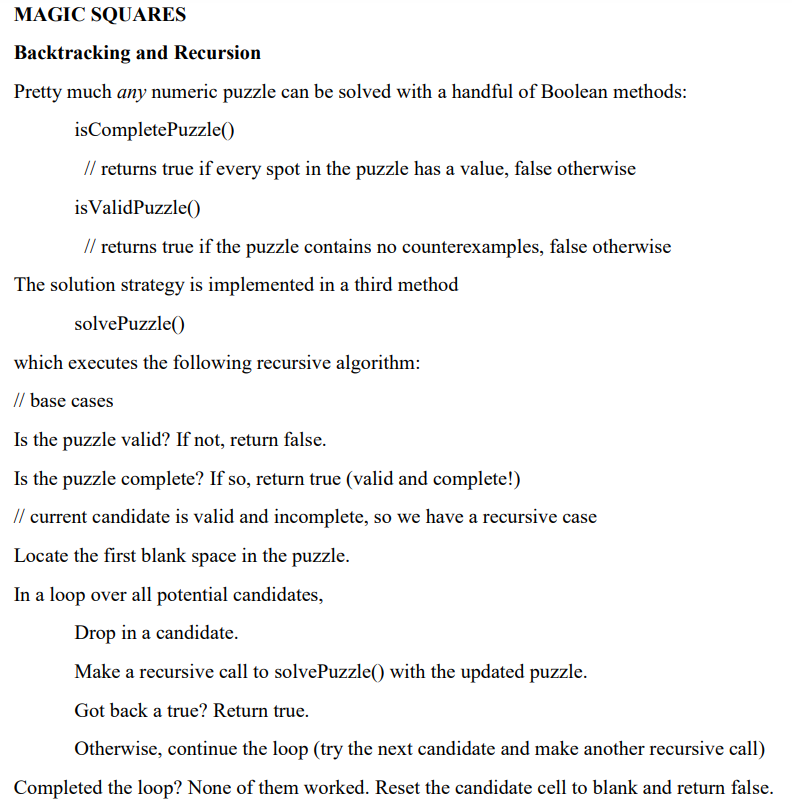
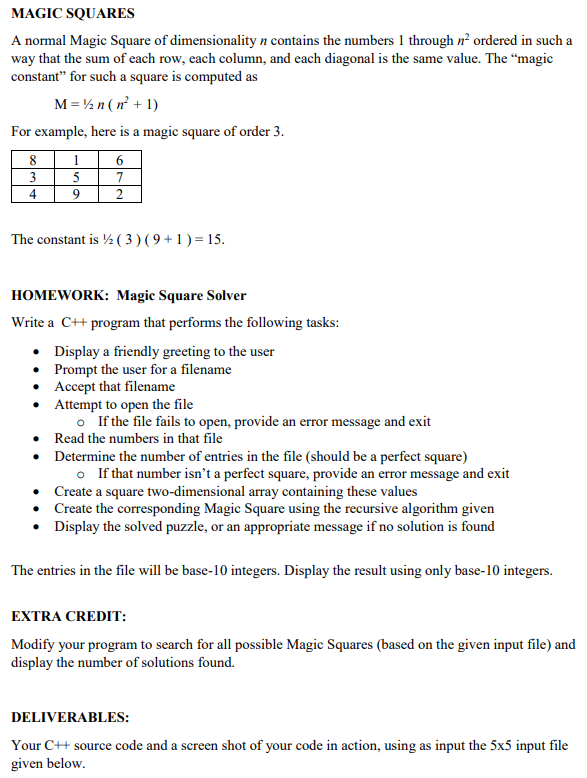
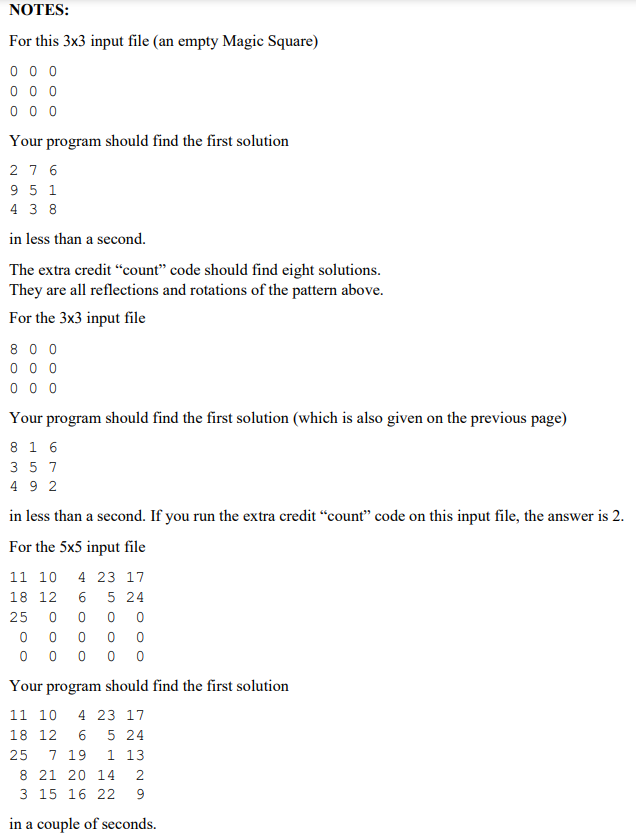
MAGIC SQUARES Backtracking and Recursion Pretty much any numeric puzzle can be solved with a handful of Boolean methods: isCompletePuzzle () // returns true if every spot in the puzzle has a value, false otherwise isValidPuzzle() // returns true if the puzzle contains no counterexamples, false otherwise The solution strategy is implemented in a third method solvePuzzle() which executes the following recursive algorithm: // base cases Is the puzzle valid? If not, return false. Is the puzzle complete? If so, return true (valid and complete!) // current candidate is valid and incomplete, so we have a recursive case Locate the first blank space in the puzzle. In a loop over all potential candidates, Drop in a candidate. Make a recursive call to solvePuzzle () with the updated puzzle. Got back a true? Return true. Otherwise, continue the loop (try the next candidate and make another recursive ca Completed the loop? None of them worked. Reset the candidate cell to blank and return fa A normal Magic Square of dimensionality n contains the numbers 1 through n2 ordered in such a way that the sum of each row, each column, and each diagonal is the same value. The "magic constant" for such a square is computed as M=1/2n(n2+1) For example, here is a magic square of order 3. The constant is 1/2(3)(9+1)=15. HOMEWORK: Magic Square Solver Write a C++ program that performs the following tasks: - Display a friendly greeting to the user - Prompt the user for a filename - Accept that filename - Attempt to open the file - If the file fails to open, provide an error message and exit - Read the numbers in that file - Determine the number of entries in the file (should be a perfect square) If that number isn't a perfect square, provide an error message and exit - Create a square two-dimensional array containing these values - Create the corresponding Magic Square using the recursive algorithm given - Display the solved puzzle, or an appropriate message if no solution is found The entries in the file will be base-10 integers. Display the result using only base-10 integers. EXTRA CREDIT: Modify your program to search for all possible Magic Squares (based on the given input file) and display the number of solutions found. DELIVERABLES: Your C++ source code and a screen shot of your code in action, using as input the 55 input file given below. For this 33 input file (an empty Magic Square) 000000000 Your program should find the first solution 294753618 in less than a second. The extra credit "count" code should find eight solutions. They are all reflections and rotations of the pattern above. For the 33 input file 800000000 Your program should find the first solution (which is also given on the previous page) 816 357 492 in less than a second. If you run the extra credit "count" code on this input file, the answer is 2. Dnotha EuE innow tin Your program should find the first solution in a couple of seconds. MAGIC SQUARES Backtracking and Recursion Pretty much any numeric puzzle can be solved with a handful of Boolean methods: isCompletePuzzle () // returns true if every spot in the puzzle has a value, false otherwise isValidPuzzle() // returns true if the puzzle contains no counterexamples, false otherwise The solution strategy is implemented in a third method solvePuzzle() which executes the following recursive algorithm: // base cases Is the puzzle valid? If not, return false. Is the puzzle complete? If so, return true (valid and complete!) // current candidate is valid and incomplete, so we have a recursive case Locate the first blank space in the puzzle. In a loop over all potential candidates, Drop in a candidate. Make a recursive call to solvePuzzle () with the updated puzzle. Got back a true? Return true. Otherwise, continue the loop (try the next candidate and make another recursive ca Completed the loop? None of them worked. Reset the candidate cell to blank and return fa A normal Magic Square of dimensionality n contains the numbers 1 through n2 ordered in such a way that the sum of each row, each column, and each diagonal is the same value. The "magic constant" for such a square is computed as M=1/2n(n2+1) For example, here is a magic square of order 3. The constant is 1/2(3)(9+1)=15. HOMEWORK: Magic Square Solver Write a C++ program that performs the following tasks: - Display a friendly greeting to the user - Prompt the user for a filename - Accept that filename - Attempt to open the file - If the file fails to open, provide an error message and exit - Read the numbers in that file - Determine the number of entries in the file (should be a perfect square) If that number isn't a perfect square, provide an error message and exit - Create a square two-dimensional array containing these values - Create the corresponding Magic Square using the recursive algorithm given - Display the solved puzzle, or an appropriate message if no solution is found The entries in the file will be base-10 integers. Display the result using only base-10 integers. EXTRA CREDIT: Modify your program to search for all possible Magic Squares (based on the given input file) and display the number of solutions found. DELIVERABLES: Your C++ source code and a screen shot of your code in action, using as input the 55 input file given below. For this 33 input file (an empty Magic Square) 000000000 Your program should find the first solution 294753618 in less than a second. The extra credit "count" code should find eight solutions. They are all reflections and rotations of the pattern above. For the 33 input file 800000000 Your program should find the first solution (which is also given on the previous page) 816 357 492 in less than a second. If you run the extra credit "count" code on this input file, the answer is 2. Dnotha EuE innow tin Your program should find the first solution in a couple of seconds