Question
Language is Java Write a class named Octagon that extends GeometricObject and implements the java.lang. Comparable interfaces, and overrides Object.toString() :String. Assume that all eight
Language is Java
Write a class named Octagon that extends GeometricObject and implements the java.lang. Comparable interfaces, and overrides Object.toString() :String. Assume that all eight sides of the octagon are of equal length. The area A of a regular octagon of side length a is given by A = 2*cot(pi/8)*a2. When implementing the toString() include the side value and area as part of the return value. When implementing Comparable use the value of the area in the comparison process. Write a test program that reads in set of octagon side values from a file (data.txt), creates an Octagon using the side, and adds them to list: ArrayList
Files to upload
: Octagon.java, Test.java, report.txt
data.txt
: 5,0,7.a,3.2,9.7,2.4,3,2
//Algorithm for test program
main:
print Name, Lab Number, and Date
open data.txt as input, report.txt as output
while not eof:
read side
create an octagon using side
add theoctagon to list
print(list) //side, area, and perimeter for each Octagon
sort(list)
print(list) //side, area, and perimeter for each Octagon
close files
The code for GeometricObject is provided here:
public abstract class GeometricObject {
private String color = "white";
private boolean filled;
private java.util.Date dateCreated;
/** Construct a default geometric object */
protected GeometricObject() {
dateCreated = new java.util.Date();
}
/** Construct a geometric object with color and filled value */
protected GeometricObject(String color, boolean filled) {
dateCreated = new java.util.Date();
this.color = color;
this.filled = filled;
}
/** Return color */
public String getColor() {
return color;
}
/** Set a new color */
public void setColor(String color) {
this.color = color;
}
/** Return filled. Since filled is boolean,
* the get method is named isFilled */
public boolean isFilled() {
return filled;
}
/** Set a new filled */
public void setFilled(boolean filled) {
this.filled = filled;
}
/** Get dateCreated */
public java.util.Date getDateCreated() {
return dateCreated;
}
/** Return a string representation of this object */
public String toString() {
return "created on " + dateCreated + " color: " + color +
" and filled: " + filled;
}
/** Abstract method getArea */
public abstract double getArea();
/** Abstract method getPerimeter */
public abstract double getPerimeter();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
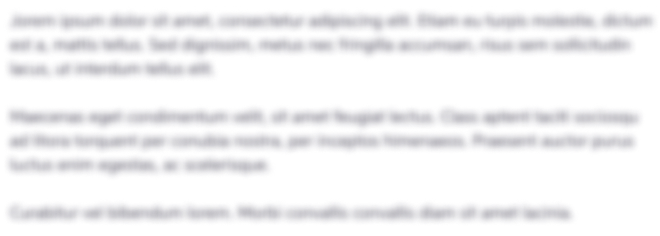
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started