Question
Language: Java GroceryBill class: /** models a grocery bill to which the cost of items can be added and removed */ public class GroceryBill {
Language: Java
GroceryBill class:
/** models a grocery bill to which the cost of items can be added and removed */ public class GroceryBill { }
GroceryBillTester class: /** * Tester for the GroceryBill class * * @author Kathleen O'Brien */ public class GroceryBillTester { public static void main(String[] args) { GroceryBill cart = new GroceryBill(); cart.add(10.25); cart.add(11.75); cart.add(25.50); System.out.println(cart.getSubtotal()); System.out.println("Expected: 47.5"); cart.remove(5.50); cart.add(3); System.out.println(cart.getSubtotal()); System.out.println("Expected: 45.0"); } }
Complete the GroceryBill class which models the checkout process at a grocery store. You are given starter code and a GroceryBillTester class which creates a GroceryBill and calls its methods.
In this class, we want to be able to add the cost of an item to the bill and remove a specific amount from the bill. We also want to able able to get the subtotal.
What does the class need to remember? That is the instance variable. What data type should it be (int, double, String)?
GroceryBill has a no-argument constructor which initializes the instance variable (subtotal) to 0. Remember that a constructor has the same name as the class.
It has the following methods:
public void add(double amount) adds this cost to the subtotal for this GroceryBill
public void remove(double amount) subtracts this cost from the subtotal for this GroceryBill
public double getSubtotal() gets the subtotal for this GroceryBill
Provide Javadoc for the class, the constructor, methods, parameters and return values.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
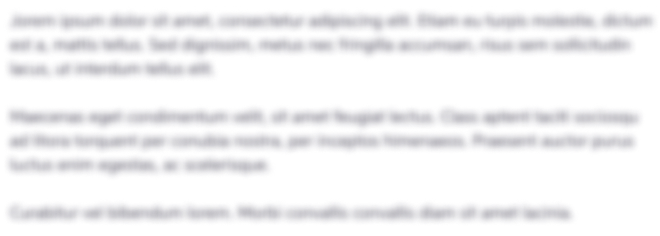
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started