Question
Learning Objectives - Modular Programming - Implementing Value-returning Functions - and Implementing Void Functions Definition 1: A Quadratic Equation is a second-order polynomial equation in
Learning Objectives
- Modular Programming
- Implementing Value-returning Functions
- and Implementing Void Functions
Definition 1: A Quadratic Equation is a second-order polynomial equation in a single variable x.
ax^2 + bx + c =0
with a not equal to 0. a is referred to as the coefficient of the quadratic term, b, the coefficient of the linear term, and c, the constant term. Because a quadratic equation is a second-order polynomial equation, the fundamental theorem of algebra guarantees that it has two solutions. These solutions may both be real or complex.
Definition 2. The quantity D= b^2- 4ac is called the discrimant of a quadratic equation.
Definition 3. The axis of symmetry of a parabola is a vertical line that divides the parabola into two congruent halves. Given any horizontal line that intersects the parabola at two points, both points are equidistant from the axis of symmetry. The axis of symmetry of a parabola is the vertical line x = -b/2a.
Definition 4. A vertex of a parabola is the point at which it crosses the axis of symmetry. It is the lowest point (minimum) when the parabola is concave upward and the highest point (maximum) when it is concave downward. The vertex of a parabola is the point (-b/2a , -b^2/4a + c)
Definition 5. The x-intercepts are the points at which the parabola crosses the x-axis; that is, the points (x, y) such that y is 0. The x-coordinates of the x-intercepts are the roots of the equation. A quadratic equation may have zero, one or two intercepts. The y-intercept is the point at which the parabola crosses the y-axis; that is, the point (x, y) such that x is 0. The x-intercepts, when they exists, are (-b+sqrtD/2a, 0) and (-b-sqrtD/2a, 0), where D is the discriminant of the quadratic equation. The y-intercept is (0, c), where c is the constant term.
To facilitate easy program design, implementation and maintenance, especially when writing large programs whose overall task can be disaggregated into several subtasks, a modular design should be used. This is the approach that you will use while writing this program.
We can view this problem as one involving two branches: 1. The coefficient of the quadratic term is 0: This is a trivial case; the program prints a message indicating that the quadratic term must be non-zero and terminates. 2. The coefficient of the quadratic term is non-zero: The program does two things. (a) It prints the equation in standard form. (b) It solves the equation and determines all of its attributes.
Generating a String Representation of a Quadratic Equation Your program will have a value-returning function that returns a string representation of a quadratic equation in standard form. To generate a string representation of the equation, a stringstream object can be used. A string stream object is similar to the iostream cout object. However, it does not output to the monitor but to a string. Suppose a stringstream variable ss is declared for a string stream. You can then use ss just as you would use cout. To retrieve the string stored in ss, ss.str() is used. To use string stream, you must use the sstream preprocessor include directive. The insertion operator can be used with a string stream object just as it is used with cout.
1. When inserting the quadratic term in the string stream, if its coefficient is 1, do not insert the coefficient; insert the term as x2. If its coefficient is -1, insert the term as -x2. For any other coefficient a, insert the term as ax2, where the positive sign is not inserted when a is positive.
2. When inserting the linear term, if its coefficient is 0, do not insert the term. If its coefficient is -1, insert the term as - x. If its coefficient is 1, insert the term as + x. For any other coefficient b, insert the term as |b|x, where |b|, the absolute value of b, is inserted along with its sign.
3. Finally, insert the constant term, |c|, along with its sign only when it is non-zero.
For example when a = 3, b = 0 and c = 7 the string -3x2 + 7 = 0 would be returned and when the parameters for a quadratic equation are a = 3, b = 4.2 and c = 0,3x2 - 4.2x = 0 would be returned.
/** * Gives a string representation of a quadratic equaition * in standard form. * @param qCoef the coefficient of the quadratic term. * @param linCoef the coefficient of the linear term * @param cTerm the constant term * @return a string representing a quadratic equation in * standard form. */ string quadToString(double qCoef, double linCoef, double cTerm)
Solving a Quadratic Equation
For any quadratic equation, its roots may fall into one of these categories:
1. The root is real and its irrational part of the solution is 0: x= {-b/2a} 2. The roots are real and the irrational part of the solution is positive: x= {-b+sqrtD/2a, -b-sqrtD/2a}
3. The roots are complex: x= {-b/2a + sqrt|D| / |2a| i, -b/2a - sqrtD/ |2a| i} where, i is a symbol representing the imaginary number (i = sqrt-1).
Observe that the solution of a quadrativ equation consists of two parts:
rationalPart= -b/2a and irrationalPart= sqrt|D|/|2a|
1. When the solution is real (not complex) and the irrational part of the solution is 0, the equation has only one root, rationalP art. The equation has only one x-intercept whose x-coordinate is rationalP art.
2. When the solutions are real and the irrational part of the solution is positive, the equation has two real roots, rationalP art + irrationalP art and rationalP art irrationalP art.
3. When the solutions are complex, the roots of the equation are rationalP art + irrationalP arti and rationalP art irrationalP arti, where rationalP art should be displayed only when it is non-zero. Also, the equation has no x-intercepts.
To solve the quadratic equation, you will define two additional sub-functions between the using directive and the main functions:
/** * Computes the discriminant of a quadratic equation with * the specified parameters. * @param qCoef the coefficient of the quadratic term. * @param linCoef the coefficient of the linear term * @param cTerm the constant term * @return the discriminant of a quadratic equation */ double discriminant(double qCoef, double linCoef, double cTerm)
/** * Computes the rational and irrational parts of the solutions * of a quadratic equation with the specified parameters * @param qCoef the coefficient of the quadratic term. * @param linCoef the coefficient of the linear term * @param cTerm the constant term * @param rat the rational part of the solution * @param irrat the irrational part of the solution * @param cmplx indicates whether or not the roots are complex; * true when the roots are complex and false if they are real. */ void solve(double qCoef, double linCoef, double cTerm, double& rat, double& irrat, bool& cmplx)
Write a C++ program call QuadraticSolver that prompts the user for the coefficient of the quadratic term, the coefficient of the linear term, and the constant term of a quadratic equation. The program then invokes the quadToString function with the relevant arguments to display the quadratic equation and determines its discriminant, roots, axis of symmetry, vertex, x-intercepts and y-intercept, invoking the appropriate sub-functions, where applicable. It also determines whether the parabola is concave upward or downward. A parabola is concave upward if the coefficient of its quadratic term is positive and concave downward when the coefficient of the quadratic term is negative.
Write the program incrementally. Write a preliminary version of the program so that it prints a message indicating that the equation is not quadratic if the input for the quadratic coefficient is 0 and calls the quadToString function with the relevant arguments to print the quadratic equation in standard form when a non-zero coefficient is entered for the quadratic term. You can then define the discriminant and solve sub-functions and add code to compute the roots and all the properties of the equation, calling the appropriate sub-functions where applicable. You may also want to incrementally add the code for solving the equation: First, the code to solve an equation whose irrational part is 0, second, the code to solve the equation when it has two real roots and, finally, the code to solve the equation when its roots are complex. Typical sample runs of the program should appear as shown below:
Listing 1: Sample Run
1 Enter the coefficient of the quadratic term -> 1
2 Enter the coefficient of the linear term -> -6
3 Enter the constant term -> 9
4
5 For the quadratic equation x ^2 - 6x + 9 = 0:
6
7 Discriminant: 0.000
8 Axis of Symmetry : x = 3.000
9 Vertex : (3.000 , 0.000)
10 y - intercept: (0.000 , 9.000)
11 x - intercept: (3.000 , 0.000)
12 Shape : Concave upward
13 Root : x = {3.00000}
Listing 2: Sample Run
1 Enter the coefficient of the quadratic term -> -4
2 Enter the coefficient of the linear term -> 0
3 Enter the constant term -> 64
4
5 For the quadratic equation -4x ^2 + 64 = 0:
6
7 Discriminant: 1024.000
8 Axis of Symmetry : x = 0.000
9 Vertex : (0.00000 , 64.000)
10 y - intercept: (0.00000 , 64.000)
11 x - intercepts: (4.000 , 0.000) and ( -4.000 , 0.000)
12 Shape : Concave downward
13 Roots : x = { -4.000 , 4.000}
Listing 3: Sample Run
1 Enter the coefficient of the quadratic term -> 3
2 Enter the coefficient of the linear term -> 45
3 Enter the constant term -> 0
4
5 For the quadratic equation 3x ^2 + 45 x = 0:
6
7 Discriminant: 2025.00000
8 Axis of Symmetry : x = -7.500
9 Vertex : ( -7.500 , -168.750)
10 y - intercept: (0.000 , 0.000)
11 x - intercepts: ( -15.000 , 0.000) and (0.000 , 0.000)
12 Shape : Concave upward
13 Roots : x = {0.000 , -15.000}
Listing 4: Sample Run
1 Enter the coefficient of the quadratic term -> 0
2 Enter the coefficient of the linear term -> 9
3 Enter the constant term -> 2.5
4
5 ERROR : The quadratic term must be nonzero .
Listing 5: Sample Run
1 Enter the coefficient of the quadratic term -> 12
2 Enter the coefficient of the linear term -> -7
3 Enter the constant term -> -12
4
5 For the quadratic equation 12 x ^2 - 7x - 12 = 0:
6
7 Discriminant: 625.000
8 Axis of Symmetry : x = 0.292
9 Vertex : (0.292 , -13.021)
10 y - intercept: (0.000 , -12.000)
11 x - intercepts: ( -0.750 , 0.000) and (1.333 , 0.000)
12 Shape : Concave upward
13 Roots : x = {1.333 , -0.750}
Listing 6: Sample Run
1 Enter the coefficient of the quadratic term -> 9
2 Enter the coefficient of the linear term -> 0
3 Enter the constant term -> 16
4
5 For the quadratic equation 9x ^2 + 16 = 0:
6
7 Discriminant: -576.000
8 Axis of Symmetry : x = -0.000
9 Vertex : ( -0.00000 , 16.000)
10 y - intercept: (0.000 , 16.000)
11 x - intercepts: none
12 Shape : Concave upward
13 Roots : x = {1.333 i , -1.333 i}
Listing 7: Sample Run
1 Enter the coefficient of the quadratic term -> 4
2 Enter the coefficient of the linear term -> -12
3 Enter the constant term -> 25
4
5 For the quadratic equation 4x ^2 - 12 x + 25 = 0:
6
7 Discriminant: -256.000
8 Axis of Symmetry : x = 1.500
9 Vertex : (1.500 , 16.000)
10 y - intercept: (0.000 , 25.000)
11 x - intercepts: none
12 Shape : Concave upward
13 Roots : x = {1.500+2.000i , 1.500 -2.000 i}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
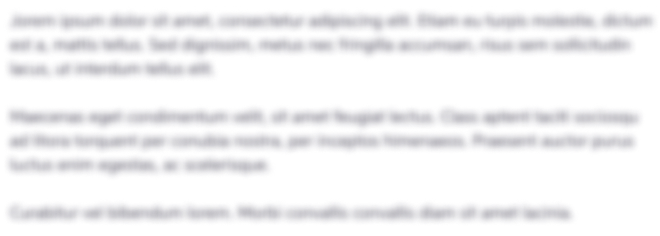
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started