Question
Write a function that reads a Float24_t value: Float24_t float24_read(void) A legitimate float24 value string is of the form: mantissabexponent where the mantissa (m) and
Write a function that reads a Float24_t value:
Float24_t float24_read(void)
A legitimate float24 value string is of the form:
"mantissabexponent"
where the mantissa (m) and the exponent (e) may have a prefix of a single "-" or "+" or no prefix and is then followed by digits of 0-9 (base 10). Remember the actual value is m×2e , noting the base 2 for the exponent.
A non-legitimate symbol, including a space, new-line () or EOF terminates the value for conversion. In such cases, where the input string is malformed, then return a Float24_t with a mantissa of 0 and exponent of -128.
Your function should call float24_normalise to ensure it is within acceptable scales, but ensure that the abs(exponent) saturates at ±127 (i.e. if the magnitude of the exponent exceeds 127 then it should be set at 127).
Note that you must use getchar(), not scanf(), and is recommended to use the function isdigit().
As with the last question, your answer should include all functions you've written up to this point.
code used so far:
#include
#include
#include
#include
typedef struct {
int16_t mantissa;
int8_t exponent;
} Float24_t;
Float24_t float24_init(int16_t mantissa, int8_t exponent)
{
Float24_t newFloat;
newFloat.mantissa = mantissa;
newFloat.exponent = exponent;
return newFloat;
}
Float24_t float24_normalise(int32_t oversizeMantissa, int8_t exponent)
{
Float24_t newFloat;
newFloat.exponent = exponent;
if (oversizeMantissa > 32767 || oversizeMantissa <= -32768) {
do {
oversizeMantissa /= 2;
newFloat.exponent++;
} while (oversizeMantissa > 32767 || oversizeMantissa < -32768);
}
newFloat.mantissa = oversizeMantissa;
return newFloat;
}
Float24_t float24_multiply(Float24_t num1, Float24_t num2)
{
int32_t mantissa = num1.mantissa * num2.mantissa;
int32_t exponent = num1.exponent + num2.exponent;
return float24_normalise(mantissa, exponent);
}
Float24_t float24_add(Float24_t num1, Float24_t num2)
{
int32_t c = num1.exponent > num2.exponent ? num1.exponent : num2.exponent;
int32_t m = (int) floor((1 * num1.mantissa / pow (2, c - num1.exponent) + 1.0 * num2.mantissa / pow ( 2, c - num2.exponent)));
return float24_normalise(m, c);
}
void float24_print (Float24_t value)
{
printf("%d * 2 ^ %d", value.mantissa, value.exponent);
}
Step by Step Solution
3.21 Rating (154 Votes )
There are 3 Steps involved in it
Step: 1
Float 24 t float 24 read void Float 24 t new Float int 16 t mant issa 0 int 8 t exponent 128 int sig...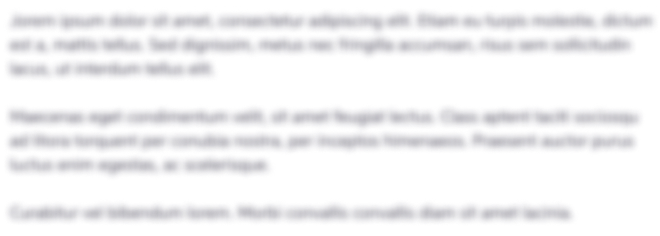
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started