Question
Lets define a datatype for storing time HH:MM We want to create this new datatype for representing time in the 24-hour format. It is reasonable
Lets define a datatype for storing time HH:MM
We want to create this new datatype for representing time in the 24-hour format. It is reasonable to define it to have two integer fields:
h for the number of hours, and
m for the number of minutes.
Notice the semicolon after the closing }, it is necessary:
struct Time { int h; int m; };
Alright, lets try to use this new fancy type. There are several ways for creating variables of this type Time. The most explicit one is to create a variable and then update its fields individually:
Time now; // creates a new variable now.h = 17; // assigns its hours field now.m = 45; // assigns its minutes field
The variable is called now, and it is storing 5:45 PM. The fields of now can be accessed as now.h ans now.m.
Alternatively, we can create a variable and immediately initialize all its fields with the following literal syntax (the order of values in curly braces is important):
Time t = { 17, 45 };
Lets write a printer function that prints a Time value on the screen in HOURS:MINUTES format:
void printTime(Time time) { cout << time.h << ":" << time.m; }
One convenient feature, which you might not need but should be aware of, is that the assignment operator works for structs, it is copying the all elements of the struct, field by field:
Time morningClass = {8, 10}; Time myAlarm; // make another variable myAlarm = morningClass; // copying printTime(morningClass); // will print 8:10 printTime(myAlarm); // will print 8:10 as well // You may be late for the class tho
A remark on C++ 11 and using C++ stuct literals
Prior to C++ 2011 standard, to use a struct, one first had to create a variable of the struct type, like in the examples above. The curly brace syntax for struct literals, such as {8, 10}, was allowed only when initilizing a struct variable, not at an arbitrary place in the program.
Thus, for instance, one couldnt create a struct object on the fly and pass it into a function, or return it from a function:
printTime({8, 10}); // was illegal return {17, 45}; // was illegal
You had to write explicitly:
Time t1 = {8, 10}; // first, had to create and initialize printTime(t1); // then call the function Time t2 = {17, 45}; // create return t2; // then return
The C++ 11 standard allows creating and initializing struct objects on the fly without making a variable to store it, so the previously illegal code is now accepted and valid. To compile your program according to the C++11 standard, use an additional command line option -std=c++11:
g++ -std=c++11 program.cpp
Task A. Simple functions for time
Create a new program time.cpp. (Copy the struct Time declaration in your program, it should be placed before main() function.)
Implement two new functions:
int minutesSinceMidnight(Time time); int minutesUntil(Time earlier, Time later);
The first function should return the number of minutes from 0:00AM until time.
The second function should receive two Time arguments earlier and later and report how many minutes separate the two moments. For example, when passing 10:30AM and 1:40PM:
minutesUntil( {10, 30}, {13, 40} ) // ==> should return 190 minutes
(A caveat: If the earlier moment of time happens to be after the later moment of time, report a negative number of minutes. Although its not difficult to achieve this if your implementation for the first function is correct.)
For testing purposes, implement a simple user interface:
$ ./time Enter first time: 10 30 Enter second time: 13 40 These moments of time are X and Y minutes after midnight. The interval between them is Z minutes.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
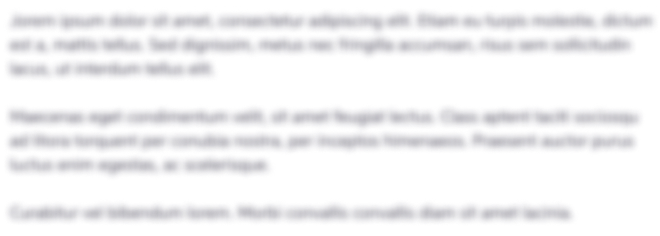
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started