Question
Let's modify our previous lab FuelCostSelection.py to the following specifications (the changes are in blue bold): Calculate the Fuel Costs for a Road Trip Fuel
Let's modify our previous lab FuelCostSelection.py to the following specifications (the changes are in blue bold):
Calculate the Fuel Costs for a Road Trip
Fuel Costs
We can estimate the cost of fuel for a Road Trip with a given number of inputs. If we know total miles our Road trip (m), how many miles per gallon our vehicle gets (mpg) and the fuel cost per gallon(CPG), we can estimate total costs for fuel required complete our road trip from point A to point B.
FuelCost = m/mpg * cpg
Where, the terms in the formula are:
m is total miles of the road trip mpg is miles per gallon of vehicle cpg is the cost per gallon for fuel, set in our Selection Structure
In our program logic, cpg is set by the following Selection Structure: Unleaded gas up to 89.00 Octane -> $6.00 per gallon Unleaded gas over 89.01 - 90.99 Octane -> $8.00 per gallon Unleaded gas over 91.00 - 94.99 Octane -> $10.45 per gallon High Octane racing fuel, 95.00 - 120.00 Octane -> 23.94 per gallon High Octane jet fuel, anything above 120 Octane -> 36.98 per gallon
Write a Python program that
takes input: Continues to take input until a sentinel value of 0 is entered (Iteration loop structure) for m (miles); input mpg and Octane
Processing: Calculates Fuel Costs (see formula above)
Output: Total miles of road trip, mpg, cpg, Octane and calculated Fuel Costs, for each trip
[15 points] submit FuelCostLoop.py **be sure to format your output to 2 decimal places.....
**be sure you have corrected your Selection Structure from previous lab if I asked you to**
Current Code
#FuelCost.py def fuel_cost(m, mpg, octane):
if octane <= 89.00: cpg = 6.00 elif octane >= 89.01 and octane <= 90.99: cpg = 8.00 elif octane >= 91.08 and octane <= 94.99: cpg = 10.45 elif octane >= 95.08 and octane <= 120.80: cpg = 23.94 elif octane > 120: cpg = 36.98
fuel_cost = m/mpg * cpg return fuel_cost
CPG: float = 6.00
m = float(input("Enter the total miles of the road trip: "))
mpg = float(input("Enter the miles per gallon of the vehicle: "))
octane = float(input("Enter the octane level: "))
fuel_cost = m / mpg * CPG
print("Total miles of road trip: {:.2f}".format(m))
print("Miles per gallon of vehicle: {:.2f}".format(mpg))
print("Cost per gallon of fuel: ${:.2f}".format(CPG))
print("Octane level: {:.2f}".format(octane))
print("Fuel cost for the trip: ${:.2f}".format(fuel_cost))
Step by Step Solution
There are 3 Steps involved in it
Step: 1
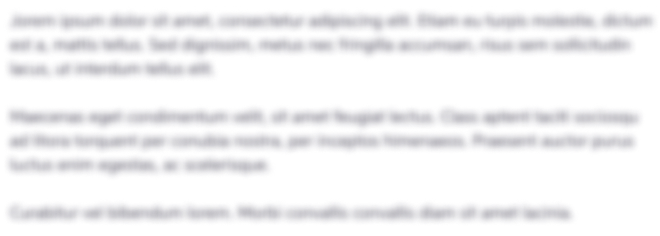
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started