Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Let's walk through a complete example, from problem description, through problem solving to code and testing. Just read along and understand. Do not do
Let's walk through a complete example, from problem description, through problem solving to code and testing. Just read along and understand. Do not do any of this until you are asked. Read it carefully and make sure you understand. Problem Description: Firstly, we understand that for some people, things like body image, weight and health can be sensitive issues. There is no intention for the following scenario to be taken personally or thought of in any way that might cause concern. It is a useful scenario for the purpose of breaking a problem into functions, which is why it is used. According to cancer.org: BMI is used to broadly define different weight groups in adults years old or older. The same groups apply to both men and women. BMI Body Mass Index can be calculated by the formula: weight in kg height in m Remember means to the power of The rough weight group categories defined by BMI are: Underweight: BMI is less than Normal weight: BMI is to Overweight: BMI is to Obese: BMI is or more This list of categories was taken from the cancer.org website, but we understand boundary conditions and should write our conditions in a better way. Eg what category should a BMI of be The client wants a program that will ask for a person's height and weight, then tell them their BMI and weight category. Algorithm When we look at the problem, we can break it up into a number of main sections. This is decomposition. get inputs making sure they're valid calculate BMI determine weight category Each of these can be implemented using functions. Our main program algorithm will call the other functions. We should notice that getting the weight and getting the height are very similar tasks that could be done with almost no difference, so this is a good situation for a reusable function. Like we did in the lecture with getvalidage, we'll pass in parameters to define the low and high bounds of the input. Unlike that function, we'll also pass in the prompt so we can customise the function's printoutput function main height getvalidnumberHeight weight getvalidnumberWeight bmi calculatebmiheight weight category determineweightcategorybmi print bmi, category At this point, we have an algorithm that represents the whole program, but does not define any of the details. This is useful, but incomplete. Let's define the algorithms for each of the functions now. For the getvalidnumber function, we should see that this uses the normal errorchecking while loop pattern. When a valid number has been entered, we "return" it function getvalidnumberprompt low, high print prompt get number while number low or number high print error, prompt get number return number For the calculatebmi function, we should see that this is a simple calculationprocessing step. When a result has been calculated, we return it function calculatebmiheight weight return weight height The job of the determineweightcategory function is not to print, but to return the category that has been determined by the function. Upgraded pattern with functions For a situation like this, we want a single, mutually exclusive result, so it seems fine to use the usual ifelifelse decision structure: function determineweightcategorybmi if bmi return underweight else if bmi return normal else if bmi return overweight else return obese However, since we are now using a function, the "else" is redundant. If any one of these conditions is True, the function returns, so we know it will only get to the next condition if the previous one was False. If we run pylint to check the code for the above algorithm, we would get the message: Unnecessary "else" after "return", remove the "else" and deindent the code inside it noelsereturn So let's "upgrade" this pattern, noting that this ONLY makes sense for use within a function because of how return works: function determineweightcategorybmi if bmi return underweight if bmi return normal if bmi return overweight return obese We could "desk check" each of these pseudocode functions. Eg if we put a BMI value of into the determineweightcategory function, we should get "overweight". Code When it comes to coding functions, you can either write main first and then each function topdown or write each function and then put it all together in main bottomup There's no right answer, but we're going to do it bottomup and test our functions one at a time before putting them all together. Look at how very similar the Python code is to the algorithm for the first function we'll write. This would be ne
Let's walk through a complete example, from problem description, through problem solving to code and testing.
Just read along and understand. Do not do any of this until you are asked. Read it carefully and make sure you understand.
Problem Description:
Firstly, we understand that for some people, things like body image, weight and health can be sensitive issues.
There is no intention for the following scenario to be taken personally or thought of in any way that might cause concern.
It is a useful scenario for the purpose of breaking a problem into functions, which is why it is used.
According to cancer.org:
BMI is used to broadly define different weight groups in adults years old or older. The same groups apply to both men and women.
BMI Body Mass Index can be calculated by the formula:
weight in kg height in m
Remember means to the power of
The rough weight group categories defined by BMI are:
Underweight: BMI is less than
Normal weight: BMI is to
Overweight: BMI is to
Obese: BMI is or more
This list of categories was taken from the cancer.org website, but we understand boundary conditions and should write our conditions in a better way. Eg what category should a BMI of be
The client wants a program that will ask for a person's height and weight, then tell them their BMI and weight category.
Algorithm
When we look at the problem, we can break it up into a number of main sections. This is decomposition.
get inputs making sure they're valid
calculate BMI
determine weight category
Each of these can be implemented using functions.
Our main program algorithm will call the other functions.
We should notice that getting the weight and getting the height are very similar tasks that could be done with almost no difference, so this is a good situation for a reusable function.
Like we did in the lecture with getvalidage, we'll pass in parameters to define the low and high bounds of the input.
Unlike that function, we'll also pass in the prompt so we can customise the function's printoutput
function main
height getvalidnumberHeight
weight getvalidnumberWeight
bmi calculatebmiheight weight
category determineweightcategorybmi
print bmi, category
At this point, we have an algorithm that represents the whole program, but does not define any of the details. This is useful, but incomplete.
Let's define the algorithms for each of the functions now.
For the getvalidnumber function, we should see that this uses the normal errorchecking while loop pattern.
When a valid number has been entered, we "return" it
function getvalidnumberprompt low, high
print prompt
get number
while number low or number high
print error, prompt
get number
return number
For the calculatebmi function, we should see that this is a simple calculationprocessing step.
When a result has been calculated, we return it
function calculatebmiheight weight
return weight height
The job of the determineweightcategory function is not to print, but to return the category that has been determined by the function.
Upgraded pattern with functions
For a situation like this, we want a single, mutually exclusive result, so it seems fine to use the usual ifelifelse decision structure:
function determineweightcategorybmi
if bmi
return underweight
else if bmi
return normal
else if bmi
return overweight
else
return obese
However, since we are now using a function, the "else" is redundant. If any one of these conditions is True, the function returns, so we know it will only get to the next condition if the previous one was False.
If we run pylint to check the code for the above algorithm, we would get the message:
Unnecessary "else" after "return", remove the "else" and deindent the code inside it noelsereturn
So let's "upgrade" this pattern, noting that this ONLY makes sense for use within a function because of how return works:
function determineweightcategorybmi
if bmi
return underweight
if bmi
return normal
if bmi
return overweight
return obese
We could "desk check" each of these pseudocode functions.
Eg if we put a BMI value of into the determineweightcategory function, we should get "overweight".
Code
When it comes to coding functions, you can either write main first and then each function topdown or write each function and then put it all together in main bottomup
There's no right answer, but we're going to do it bottomup and test our functions one at a time before putting them all together.
Look at how very similar the Python code is to the algorithm for the first function we'll write. This would be ne
Step by Step Solution
There are 3 Steps involved in it
Step: 1
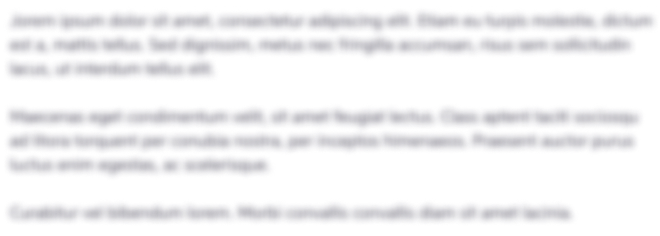
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started