Question
Line Class Write a class called Line that represents a line segment between two Point(s). Your Line objects should have the following : 1. data
Line Class Write a class called Line that represents a line segment between two Point(s). Your Line objects should have the following :
1. data fields: These internal variables have private accessibility.
i. p1 : represents one location in coordinate space.
ii. p2 : represents another location in coordinate space.
2. constructors: The overloaded constructors will construct and initialize a Line object in coordinate space with the values specified:
i. The first constructor is the default constructor of the Line class. It calls the third constructor, setting the values to (0, 0, 1, 1). public Line()
ii. The second constructs a new Line with the two given end Point(s). public Line(Point p1, Point p2)
iii. The third constructs a new Line that contains the given two Point objects specified with the values (x1,y1) and (x2,y2) respectively.
public Line(int x1, int y1, int x2, int y2) 3. mutators: These methods modify the values of the data fields p1,p2.
i. setEndPoints : This method sets the end point location of a Line object. public void setEndPoints(Point p1, Point p2)
ii. translate: This method translates each end points x-value by dx along the x-axis and each end points y-value by dy along the y-axis, giving it a resulting location at (x1 + dx, y1 + dy, x2 + dx, y2 + dy) public void translate(int dx, int dy) 4.
accessors: These are methods that retrieves the information contained within the data. Similar to read-only operations.
i. getP1 : This method returns the Line first end point. public Point getP1()
ii. getP2 : This method returns the Line second end point. public Point getP2()
iii. isCollinear : This method returns true if the given Point is Collinear with the Point(s) of the Line. If the slope of each pair of points is the same for each pair, then the points are collinear. Note that this fails if the points have the same x coordinates, so you will have to handle this as a special case in your code. In addition, you may want to round all slope values to a reasonable accuracy, such as four decimal places past the decimal point, since the double type is imprecise. public boolean isCollinear(Point p)
iv. magnitude: This method returns the magnitude or length of the Line. The magnitude of a line is equal to ( (x2 -x1) 2 + (y2- y1)2 ). public double magnitude()
v. slope : This method returns the slope of the Line. The slope of a line between two points (x1, y1) and (x2, y2) is equal to (y2- y1)/(x2-x1). If x2 equals x1 the denominator is zero (0) and the slope is undefined, so this method must throw an exception in this case. public double slope() vi. toString : This method returns a string representation of the Line object, such as [(1, 2) , (6, 8)] . public String toString()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
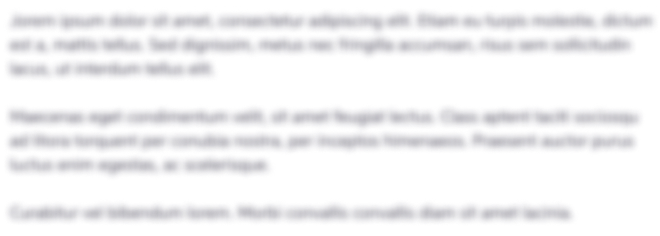
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started