Question
Linked Stack project Implement StackDriver program : Presents menu and calls methods in the interface. Revise pop() to handle empty stack. Complete isEmpty() method :
Linked Stack project
Implement StackDriver program: Presents menu and calls methods in the interface.
Revise pop() to handle empty stack.
Complete isEmpty() method: Assure seamless integration into pop, display and peek
Code display() method
Code:
class LinearNode.java
package jsjf;
/** * Represents a node in a linked list. * * @author Java Foundations * @version 4.0 */ public class LinearNode
/** * Creates an empty node. */ public LinearNode() { next = null; element = null; }
/** * Creates a node storing the specified element. * @param elem element to be stored */ public LinearNode(T elem) { next = null; element = elem; }
/** * Returns the node that follows this one. * @return reference to next node */ public LinearNode
/** * Sets the node that follows this one. * @param node node to follow this one */ public void setNext(LinearNode
/** * Returns the element stored in this node. * @return element stored at the node */ public T getElement() { return element; }
/** * Sets the element stored in this node. * @param elem element to be stored at this node */ public void setElement(T elem) { element = elem; } } class LinkedStack.java
package jsjf;
import jsjf.exceptions.*;
/** * Represents a linked implementation of a stack. * * @author Java Foundations * @version 4.0 */ public class LinkedStack
/** * Creates an empty stack. */ public LinkedStack() { count = 0; top = null; }
/** * Adds the specified element to the top of this stack. * @param element element to be pushed on stack */ public void push(T element) { LinearNode
temp.setNext(top); top = temp; count++; }
/** * Removes the element at the top of this stack and returns a * reference to it. * @return element from top of stack * @throws EmptyCollectionException if the stack is empty */ public T pop() throws EmptyCollectionException { if (isEmpty()) throw new EmptyCollectionException("stack");
T result = top.getElement(); top = top.getNext(); count--;
return result; }
/** * Returns a reference to the element at the top of this stack. * The element is not removed from the stack. * @return element on top of stack * @throws EmptyCollectionException if the stack is empty */ public T peek() throws EmptyCollectionException { // To be completed as a Programming Project return null; // temp }
/** * Returns true if this stack is empty and false otherwise. * @return true if stack is empty */ public boolean isEmpty() { // To be completed as a Programming Project return true; // temp }
/** * Returns the number of elements in this stack. * @return number of elements in the stack */ public int size() { // To be completed as a Programming Project return 0; // temp }
/** * Returns a string representation of this stack. * @return string representation of the stack */ public String toString() { // To be completed as a Programming Project return ""; // temp } } class StackADT.java
package jsjf;
/** * Defines the interface to a stack collection. * * @author Java Foundations * @version 4.0 */ public interface StackADT
/** * Removes and returns the top element from this stack. * @return the element removed from the stack */ public T pop();
/** * Returns without removing the top element of this stack. * @return the element on top of the stack */ public T peek();
/** * Returns true if this stack contains no elements. * @return true if the stack is empty */ public boolean isEmpty();
/** * Returns the number of elements in this stack. * @return the number of elements in the stack */ public int size();
/** * Returns a string representation of this stack. * @return a string representation of the stack */ public String toString(); } EmptyCollectionException.java
package jsjf.exceptions;
/** * Represents the situation in which a collection is empty. * * @author Java Foundations * @version 4.0 */ public class EmptyCollectionException extends RuntimeException { /** * Sets up this exception with an appropriate message. * @param collection the name of the collection */ public EmptyCollectionException(String collection) { super("The " + collection + " is empty."); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
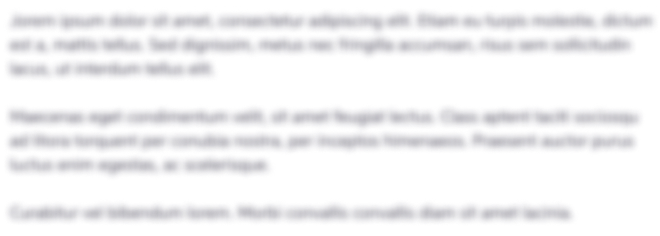
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started