Question
LINKEDLIST.C: include #include #include // Demo program. // The APIs may not be the best. typedef struct node_tag { int v; struct node_tag * next;
LINKEDLIST.C:
include
#include
#include
// Demo program.
// The APIs may not be the best.
typedef struct node_tag {
int v;
struct node_tag * next; // A pointer to this type of struct
} node; // Define a type. Easier to use.
node * create_node(int v) {
node *p = malloc(sizeof(node)); // Allocate memory
assert(p != NULL); // you can be nicer
// Set the value in the node.
p->v = v; // you could do (*p).v
p->next = NULL;
return p; // return
}
node * prepend(node * head, node * newnode) {
newnode->next = head;
return newnode;
}
node * find_node(node * head, int v) {
while (head != NULL) {
if (head->v == v)
return head;
head = head->next;
}
return head;
}
node * find_last(node * head) {
if (head != NULL) {
while (head->next != NULL)
head = head->next;
}
return head;
}
node * append(node * head, node * newnode) {
node *p = find_last(head);
if (p == NULL)
return newnode;
newnode->next = p->next;
p->next = newnode;
return head;
}
node * delete_node(node * head, int v) {
/* TODO implement me!! */
printf("Deleting a node is not supported. ");
return head;
}
node * delete_list(node * head) {
while (head != NULL) {
node *p = head->next;
free(head);
head = p;
}
return head;
}
void print_list(node *head) {
printf("[");
while (head) {
printf("%d, ", head->v);
head = head->next;
}
printf("] ");
}
void print_node(node * p) {
printf("%p: v=%-5d next=%p ", p, p->v, p->next);
}
void print_list_details(node *head) {
while (head) {
print_node(head);
head = head->next;
}
}
int main(int argc, char* argv[]) {
int i;
int res;
node *head = NULL;
res = scanf("%d", &i);
while (res == 1) {
if (i
head = delete_node(head, -i);
} else if (i == 0) {
printf("head = %p ", head);
print_list_details(head);
} else {
node *p;
p = find_node(head, i);
if (p != NULL) {
print_node(p);
}
else {
p = create_node(i);
head = (i & 1) ? prepend(head, p) : append(head, p);
}
}
print_list(head);
res = scanf("%d", &i);
}
head = delete_list(head);
return 0;
}
2. The linked list example you have studied in lecture reads integers from the standard input and maintains a list. The program performs the following operations on the list for each integer it reads: 1. For a positive integer, the program checks if the integer is already on the list. If so, the program displays information about the node that stores the integer. If not, the program adds the integer to the list, either at he beginning or at the end, depending on its parity. 2. If the input integer is 0, the program prints out the details of the list. 3. For a negative integer, the program removes its absolute value from the list if the absolute value is on the list. Otherwise, the list does not change. The list is displayed every time an integer is processed. Currently, the program supports features 1 and 2 but not 3. Complete the function delete_node( in the template linkedlist.c so that the absolute value of a negative integer can be removed from the list. Do not change the other functions. Below is an example session with the program. Note that memory addresses will differ between runs
Step by Step Solution
There are 3 Steps involved in it
Step: 1
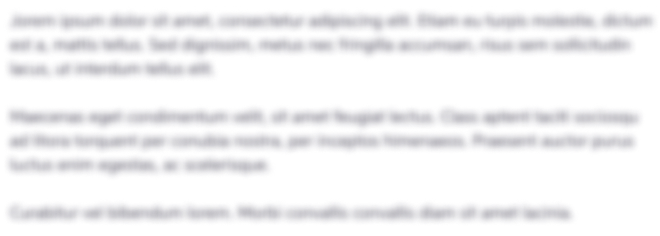
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started