Question
Linux Objective: Write a VCard++ Program Making VCard++ VCards are a text file format recognized for importing and exporting address book items. VCards are typically
Linux
Objective: Write a VCard++ Program
Making VCard++
VCards are a text file format recognized for importing and exporting address book items. VCards are typically used on Macs, but are also recognized by G Mail. In this assignment, you will write a program named vcard which will ask the user a series of questions in order to construct the vcard. The information asked of your user will include:
name
e-mail address
optional telephone number, street address and organization.
Procedure
vcard will allow the user to either (1) enter a single vcard and either create a new file with it, or (2) append to an existing vcard file with the new entry. Your vcard program should create the working current vcard in a temporary file. Then, append it to the final file when it is complete and all data has been entered correctly. The following is one approach for completing your own implementation:.
Code to check user input: Each piece of the vcard will be produced by a separate function. The function will ask the user for the data and check it. If the data is okay, the function will output the data and succeed. If the data is not correct, the function fails. There will be a handful (about six) of these small functions.
A master function to output a single vcard. It will call each of the functions to output a piece. The master function will succeed if all of it's subordinate functions succeed. If any subordinate function fails, the master function will fail immediately.
The main program is responsible for opening the vcard file. This file must be given to vcardon the command-line. It may be an existing file, or a new file. The main program must ensure the vcard file is writeable before any work is done. If so, it then creates a temporary file, opens standard output to the temporary file, and then calls the master function. If the master function succeeds, the temp file is appended to the vcard file. Finally, the temp file is deleted. The program exists with success or failure status, depending on whether it appended a vcard to the file.
Your function must be free of side-effects and have a clean interface. Notice that the functions do not need arguments: each function will write it's data to standard output, which will be pre-opened by the main program.
Here is an example Vcard:
BEGIN:VCARD VERSION:3.0 FN:Mr. Greg Boyd N:Boyd;Greg;;Mr.; ORG:City College of San Francisco;Computer Science TEL;TYPE=work,voice:415-239-3139 TEL;TYPE=cell:(415)444-4444 EMAIL;TYPE=INTERNET:gboyd@ccsf.edu BDAY:1980-01-14 NOTE:Greg was in my Android class Fall 2016 END:VCARD
Breaking this down into sections:
The following fields are required for a VCard:
Header section. Required. Text exactly as indicated | BEGIN:VCARD VERSION:3.0 |
---|---|
Footer section. Required. Text exactly as indicated | END:VCARD |
Name section. Required. FN is a formatted name. N is a name deconstructed into pieces: Last;First;Middle(s);Prefix;Suffix. The example is Dr. Ann Marie Sue Gonzales. | FN:Dr. Ann Marie Sue Gonzales N:Gonzales;Ann;Marie Sue;Dr.; |
The following fields are optional for a VCard:
Org section. Format is ORG:Company;Department;Sub-department | ORG:ABC Co.;Marketing; |
---|---|
Tel section. Adds a telephone type. We will limit this to cell phones for this assignment We will also limit phone numbers to US numbers. (see notes for phone number verification) | TEL;TYPE=cell:(415)444-4444 |
email. simple (see notes for verification) | EMAIL;TYPE=INTERNET:gboyd@ccsf.edu |
Birthday (Bday). YYYY-MM-DD (see notes) | BDAY:1980-01-14 |
Note - as indicated. Disallow , \ and ; | NOTE:Greg was in my class Fall 2016 |
Notes
There are a lot of things to worry about in this program if you want to completely validate input. Do the best you can. Here are some details that you can neglect but may come up in your own implementation.
Although you should try to validate the birth date, you don't have to ensure that the day entered is legal given the month. Just make sure that the day is within [1, 31].
You don't have to check every entry for commas and semi-colons, just check the two fields where it might reasonably be expected: the Org field and the Note field.
Don't forget that all user-entered fields excepts the name are optional! Don't force the user to enter a field that is not required.
Do the best you can but no need to stress about all the details. You could endlessly improve your script!
Hint: Here is a function you can use to escape delimiters if they appear in user-input:
fix() { # precede each instance in $1 of , and ; with \ # and output the result. Use with command-substitution. echo "$1" | sed 's/\([,\;]\)/\\\1/g' }
For the birthday, you can have your user enter the pieces individually as indicated, then construct it. Note: day and month must be two-digits (i.e. 02 for 2). Make it easy for the user. An idea for presenting extra credit in class: Allow the user to enter month names and convert them, or, better, allow them to enter a reasonably formatted date then check it with your own regular expression. At the very least, you must ensure that the month and year make sense. (can they have a year in the future?). Here is sample code for the bday function provided here
Note that in this assignment, any error is fatal. We don't have loops yet, so the user cannot re-enter their data. If they make a mistake, tell them what happened, then return a failure status and the vcard should not be added to the file.
Testing
One way to test your vcard result is to load the file into G mail, Go to contacts -> more-> import -> vcard format. It will take you to the old contacts page to enter it. If you give it a vcard like that above it should fill in the fields appropriately.
Some Test Cases
BEGIN:VCARD VERSION:3.0 N:Ginsburg;Ruth;Bader;Ms.; FN: Ruth Bader Ginsburg ORG:SCOTUS;Justice\, Associate TEL;TYPE=CELL:8002221111 EMAIL;TYPE=INTERNET:rbg@scotus.gov BDAY:1933-03-13 END:VCARD BEGIN:VCARD VERSION:3.0 N:Trump;Donald;J;Mr.; FN: Donald J Trump ORG:Executive Branch\, US Government;President TEL;TYPE=HOME,VOICE:(202)456-1414 TEL;TYPE=WORK,VOICE:202.456.1414 EMAIL;TYPE=INTERNET:prez@whitehouse.gov BDAY:1946-06-14 NOTE:Current president\; Barrack H. Obama was president until Jan 20\, 2017. END:VCARD
Step by Step Solution
There are 3 Steps involved in it
Step: 1
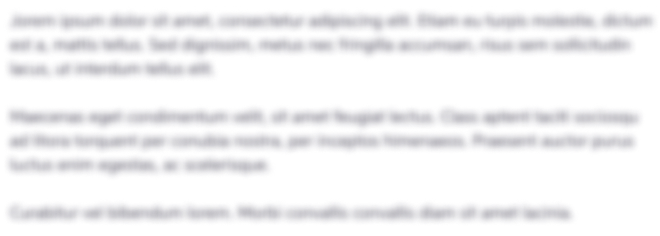
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started