Question
Looking for help on my current Java Project. It is to create a pacman game I have most of the code done I just need
Looking for help on my current Java Project.
It is to create a pacman game I have most of the code done I just need help on adding the dots for the pacman to eat and then the ghosts.
Here is the program requirments
You are going to use the maze from Lab 4 and recreate that maze. Then, instead of blank spaces, you are to put dots in there. Then, you should have a single player that can move through the maze collecting the dots. Feel free to use the collision detection from the first three labs.
You should have 3 lives. As you collect dots, the score should continually update on the screen.
Finally, you should have a couple of monsters that follow you around the screen autonomously. Remember, they are unable to move through walls. However, if they hit you lose a life and the level is over after all the dots are collected.
So if anyone can help with the dots and ghosts that would be appericated.
Code follows
import java.awt.Color; import java.awt.Dimension; import java.awt.Image; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.io.File; import java.io.FileNotFoundException; import java.util.Arrays; import java.util.Scanner; import javax.swing.ImageIcon; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane;
public class Maze extends JFrame implements KeyListener {
private static final String[] FILE = { "maze1.txt", "maze2.txt" }; private static final int mazeWidth = 50; private static final int mazeHeight = 50; private static final int LEFT = -1; private static final int RIGHT = 1; private static final int UP = -1; private static final int DOWN = 1; private int[][] maze; private JLabel[][] mazeLabel; private int row; private int col; private int entryX = -1; private int entryY = -1; private int exitX = -1; private int exitY = -1; private int currX = -1; private int currY = -1; private ImageIcon playerImg; private boolean hasWon;
public Maze() { super("Maze");
// Reads the maze from input file startMaze();
if (this.maze.length > 0) { // Finds entry and exit findStartEnd();
if ((this.entryX != -1) && (this.entryY != -1) && (this.exitX != -1) && (this.exitY != -1)) { // Draws maze drawMaze();
// Sets current position this.currX = this.entryX; this.currY = this.entryY;
// Place the player in the maze setPlayer(); } else // Prints if error reading the input from maze ie no 0's on border System.out.println("No Entry/Exit point(s) found."); } else { System.out.println("No maze found."); }
setResizable(false); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setLocationRelativeTo(null);
addKeyListener(this); requestFocus(); this.hasWon = false; }
private void startMaze() { // Selects a random file for the maze, this fullfils requirement#3 int n = (int) (Math.random() * 10) % 2;
// Use scanner file to read it the maze.txt file. I also had to look for help with the try exception as i was having trouble getting the file read in this was what was best suggested so // so this part isn't my code and i know we will learn about the try later but just wanted to give a heads up. Scanner file = null; try { file = new Scanner(new File(FILE[n]));
// This puts the file into the array, once again not my code here here had help with this part. this fullfills requiremnt 2 i think? String[] lines = new String[0]; while (file.hasNextLine()) { int len = lines.length; lines = Arrays.copyOf(lines, len + 1); lines[len] = file.nextLine().replaceAll("\\s+", ""); }
if (lines.length > 0) { this.row = lines.length; this.col = lines[0].length(); this.maze = new int[row][col];
for (int i = 0; i
private void drawMaze() {
// This draws the maze from the txt file. setLayout(null); getContentPane().setPreferredSize(new Dimension((col * mazeWidth), (row * mazeHeight))); pack(); ImageIcon image = new ImageIcon("brick.jpg"); // This resizes the image to the Maze, had help here as well with the Scale defualt I looked this up online. image = new ImageIcon(image.getImage().getScaledInstance(mazeWidth, mazeHeight, Image.SCALE_DEFAULT));
this.mazeLabel = new JLabel[row][col];
int y = 0; for (int i = 0; i
for (int j = 0; j
if (this.maze[i][j] == 1) this.mazeLabel[i][j].setIcon(image); else this.mazeLabel[i][j].setBackground(Color.WHITE);
// Adds Jlabel/Maze into the main panel add(this.mazeLabel[i][j]);
x += mazeWidth; } y += mazeHeight; } }
//This is the method/logic that finds the start of the maze and exit of the maze. Had some help with this part as well as i was having trouble ending the game private void findStartEnd() { for (int i = 0; i
if (((this.entryX == -1) && (this.entryY == -1)) || ((this.exitX == -1) && (this.exitY == -1))) { for (int i = 0; i
//Puts the player in the maze private void setPlayer() { playerImg = new ImageIcon("2000px-Pacman.svg.png"); playerImg = new ImageIcon(playerImg.getImage().getScaledInstance(mazeWidth, mazeHeight, Image.SCALE_DEFAULT)); this.mazeLabel[currX][currY].setIcon(playerImg); }
// checks the current loction of player private void setNewLocation(int newX, int newY) { this.mazeLabel[currX][currY].setIcon(null); this.mazeLabel[currX][currY].setBackground(Color.WHITE); currX = newX; currY = newY; this.mazeLabel[currX][currY].setIcon(playerImg); }
// This checks to see if a horizontal move is valid and if there is a open space private void checkHorizontal(int dir) { if (dir == LEFT) { if ((currY > 0) && (this.maze[currX][currY - 1] == 0)) { setNewLocation(currX, currY - 1); }
} else if (dir == RIGHT) { if ((currY
// This checks to see if a vertical move is valid and if there is a open space private void checkVertical(int dir) { if (dir == UP) { if ((currX > 0) && (this.maze[currX - 1][currY] == 0)) { setNewLocation(currX - 1, currY); } } else if (dir == DOWN) { if ((currX
// Player movement i use awsd for movement public void keyPressed(KeyEvent ke) { if (!hasWon) { switch (ke.getKeyCode()) { case KeyEvent.VK_A: checkHorizontal(LEFT); break; case KeyEvent.VK_W: checkVertical(UP); break; case KeyEvent.VK_D: checkHorizontal(RIGHT); break; case KeyEvent.VK_S: checkVertical(DOWN); } // Check if the player exits the maze if ((currX == exitX) && (currY == exitY)) { this.hasWon = true; JOptionPane.showMessageDialog(this, "You found the exit"); } repaint(); } }
@Override public void keyReleased(KeyEvent arg0) { }
@Override public void keyTyped(KeyEvent arg0) { } public static void main(String[] args) { Maze maze = new Maze(); maze.setVisible(true); } }
2000px-Pacman.svg.png
brick.jpg
Step by Step Solution
There are 3 Steps involved in it
Step: 1
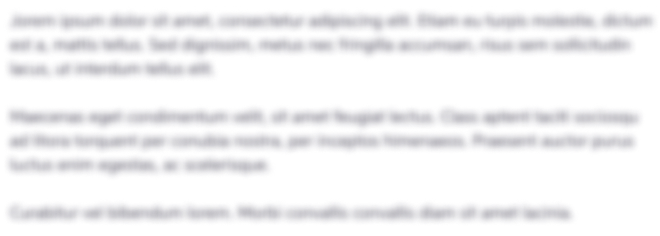
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started