Question
Looking for help with filling out the TODO portion of the following code snippet: void Stack::copy_of(const Stack& rhs){ //Makes an exact copy of Stack rhs
Looking for help with filling out the TODO portion of the following code snippet:
void Stack::copy_of(const Stack& rhs){ //Makes an exact copy of Stack rhs into this stack if (this != &rhs) { //makes sure that it does not make a copy of the stack itself while (!isEmpty()) pop(); // First empty the contents of this stack in case it has some items
if (!rhs.topPtr) topPtr = nullptr; // if rhs is an empty stack, this one will also be an empty stack else { //TODO // Make a copy of each node in rhs and link it to the list of this stack
} }
}
The header file it references is below:
class StackNode
{
public:
StackNode( int e = 0 , StackNode* n = nullptr){
item = e;
next = n;
}
int item;
StackNode* next;
};
class Stack{
public:
Stack(); // default constructor
Stack(const Stack& rhs); // copy constructor
~Stack(); // destructor
Stack& operator=(const Stack& rhs); // assignment operator
bool isEmpty();
void push(int newItem);
void pop();
int topAndPop();
int getTop() ;
void copy_of(const Stack& rhs);
private:
StackNode *topPtr; // pointer to the first node in the stack
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
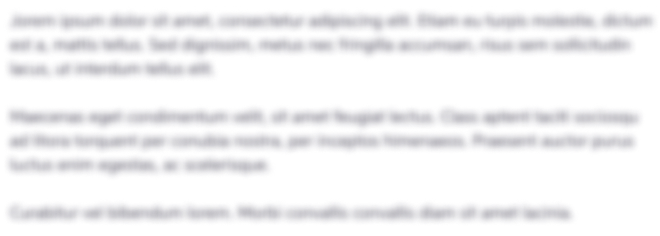
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started