Question
LoopWithDice.java : import java.util.*; public class LoopWithDice { /*Write a method inside this application called rollOdds() that takes 2 paramters: 1. die, a Die object
LoopWithDice.java :
import java.util.*;
public class LoopWithDice
{
/*Write a method inside this application called rollOdds() that takes 2
paramters:
1. die, a Die object
2. num, an integer
The method rolls the die num times and returns the number of times the
die landed on an odd face value. You MUST use a do-while loop for this
problem.
*/
public static int rollOdds(Die die,int num)
{
int total=0;//setup your counter
int count=0;//this var will count from [0-num[ (also called initial step)
do
{
die.roll();
if (die.getFaceValue() %2 ==1)
total++;
count++;//increment
}
while (count return total; } public static void main(String[] args) { Scanner scan=new Scanner(System.in); System.out.println("Enter the number of times to roll the die:"); int numOfTimes=scan.nextInt(); Die d1=new Die(); //here, let's invoke method rollOdds int oddCount=rollOdds(d1,numOfTimes); System.out.println("Total time the dia landed on an odd face: "+oddCount); } } Die.java : public class Die { private int faceValue; //private field using an access modifier called private public Die() { //faceValue=(int)(Math.random()*6)+1; roll(); } public Die(int value) { faceValue=value; } public int getFaceValue() { return faceValue; } public void setFaceValue(int value) { faceValue=value; } public void roll() { faceValue=(int)(Math.random()*6)+1; } public String toString() { return "Die with face: "+faceValue; } } Coin.java : public class Coin { private final int HEADS = 0; private final int TAILS = 1; private int face; public Coin() { flip(); } public void flip() { face = (int) (Math.random() * 2); } public boolean isHeads() { return (face == HEADS); } public String toString() { String faceName; if (face == HEADS) faceName = "Heads"; else faceName = "Tails"; return faceName; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
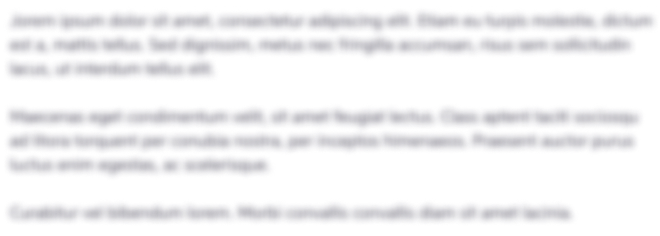
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started