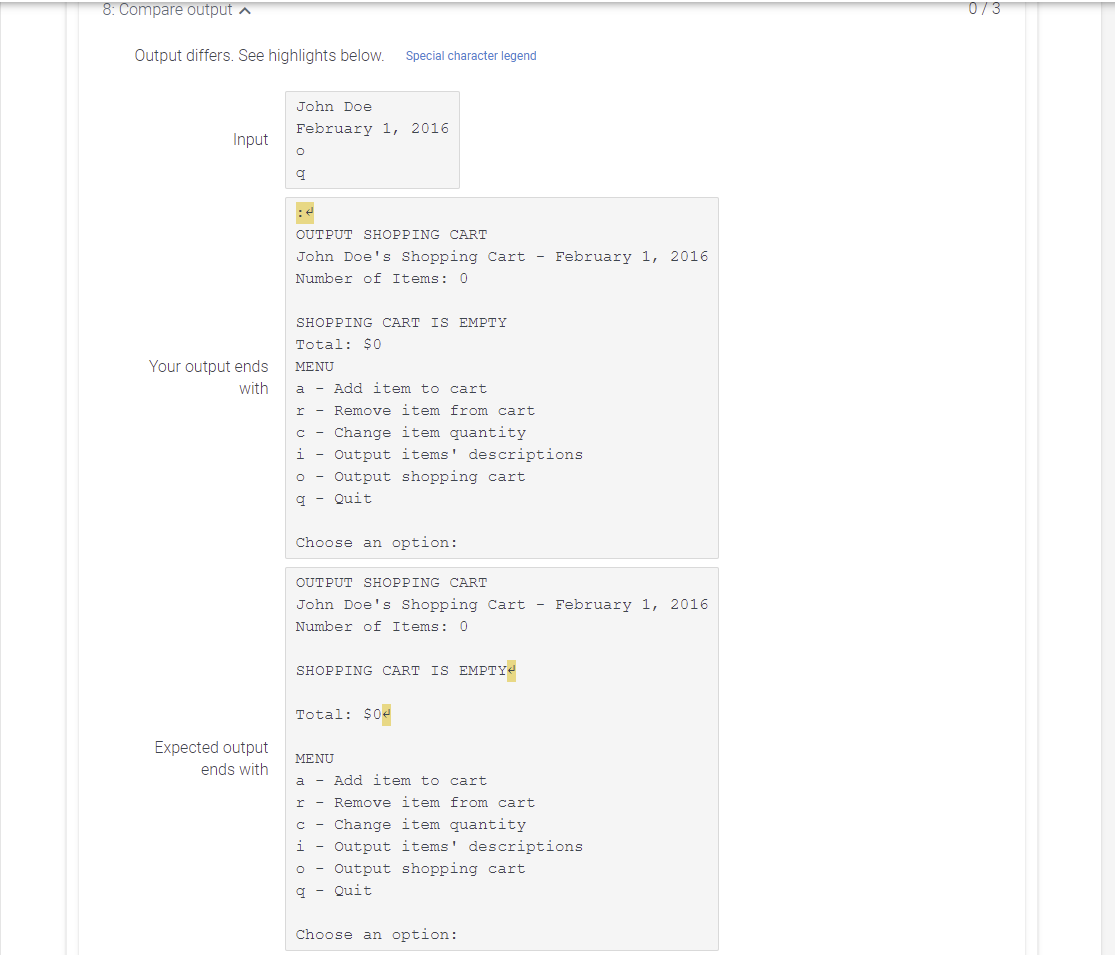
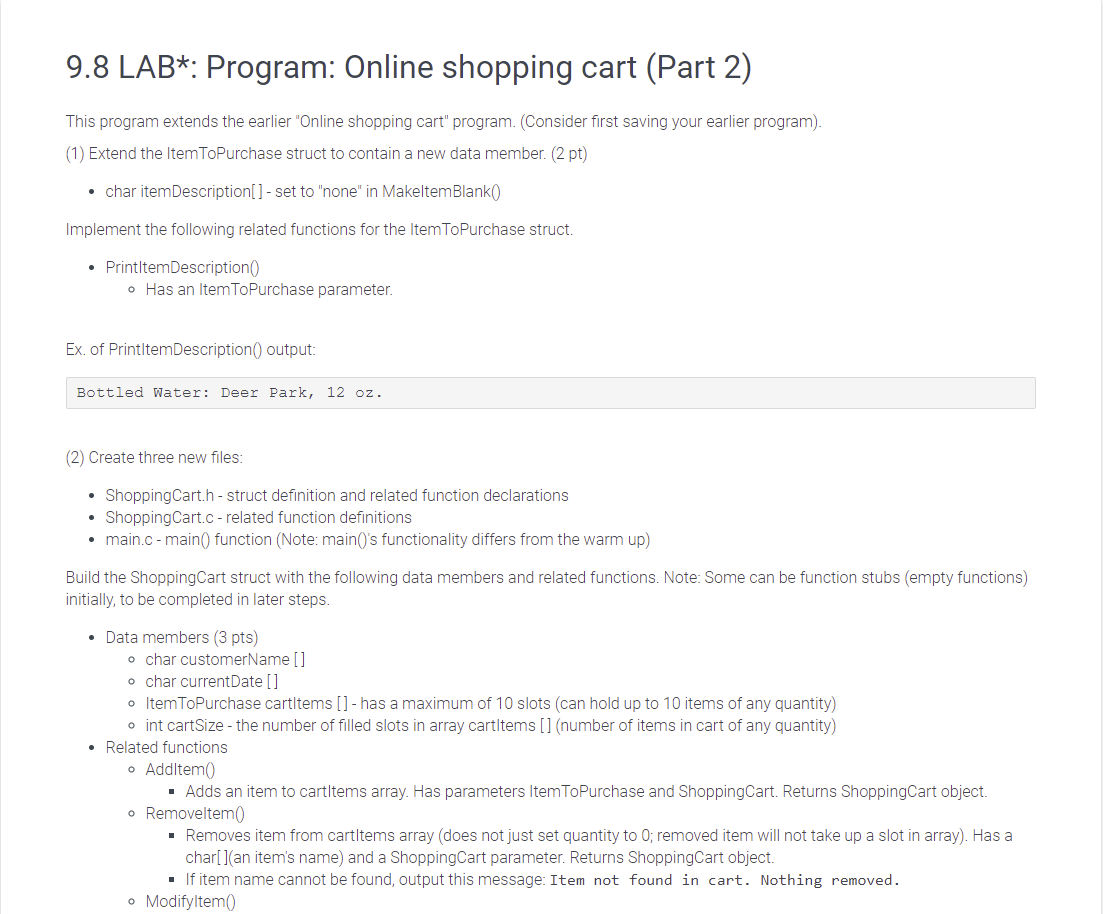
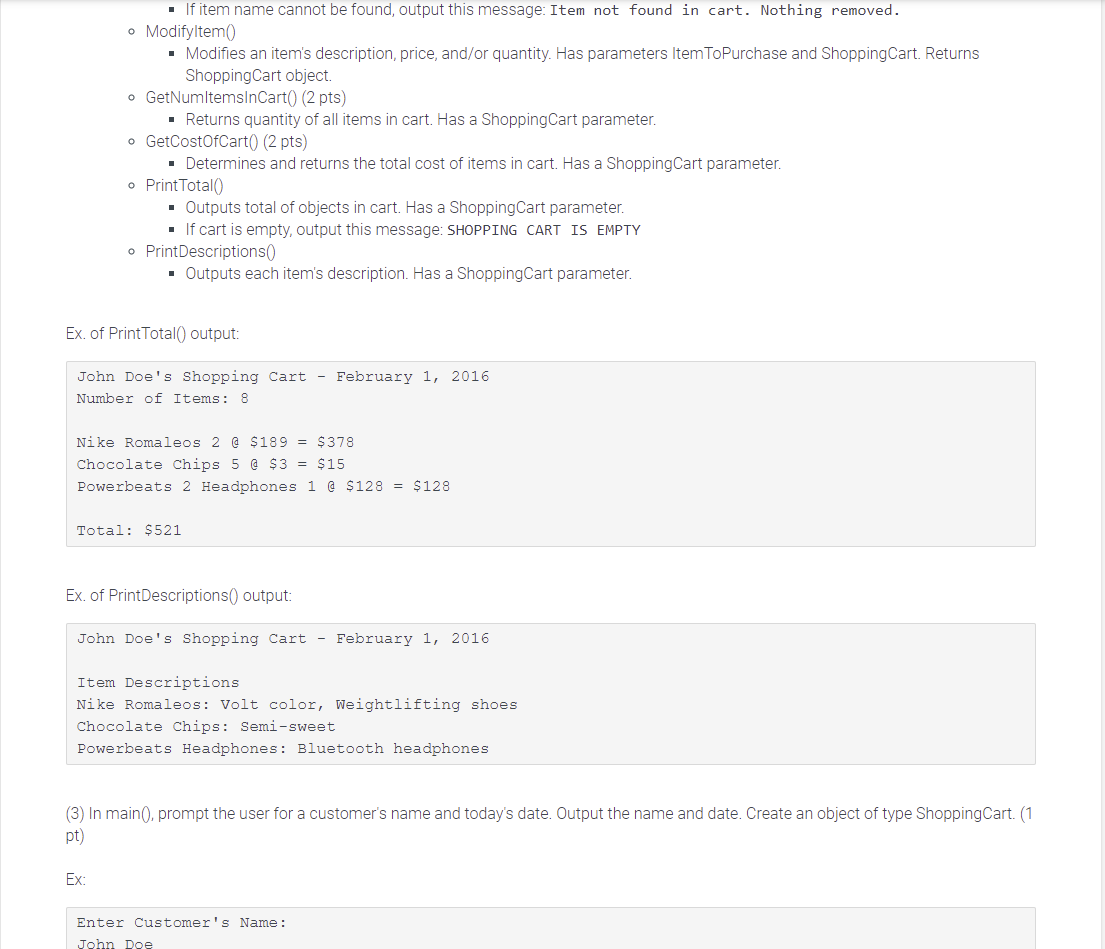
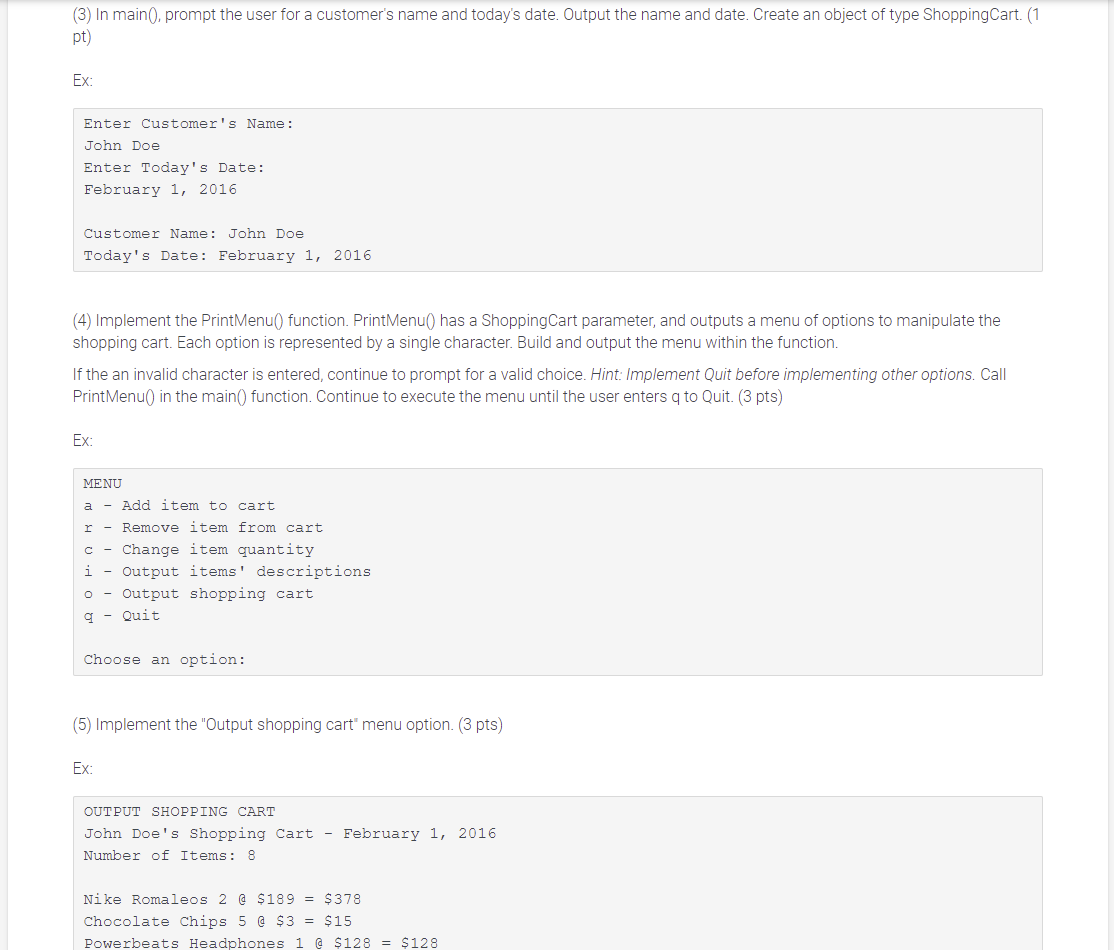
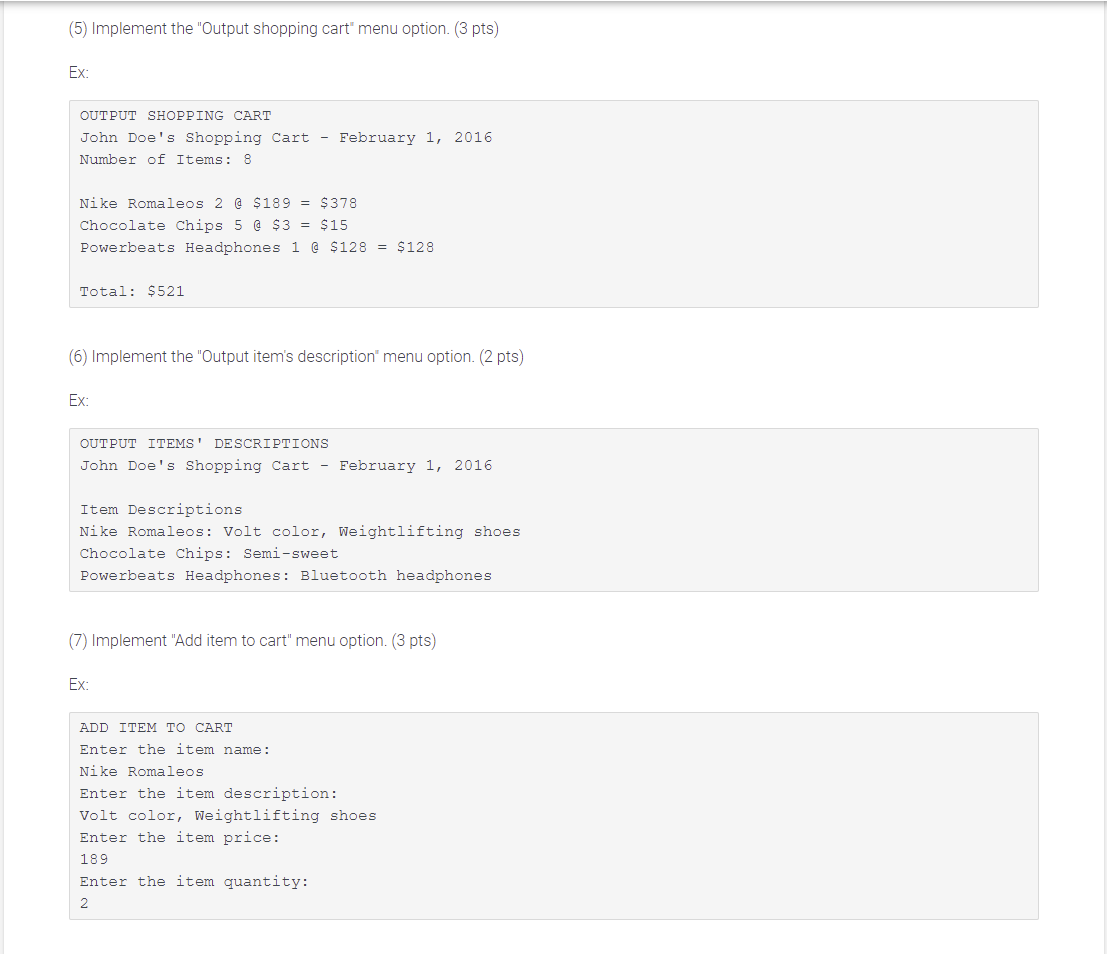
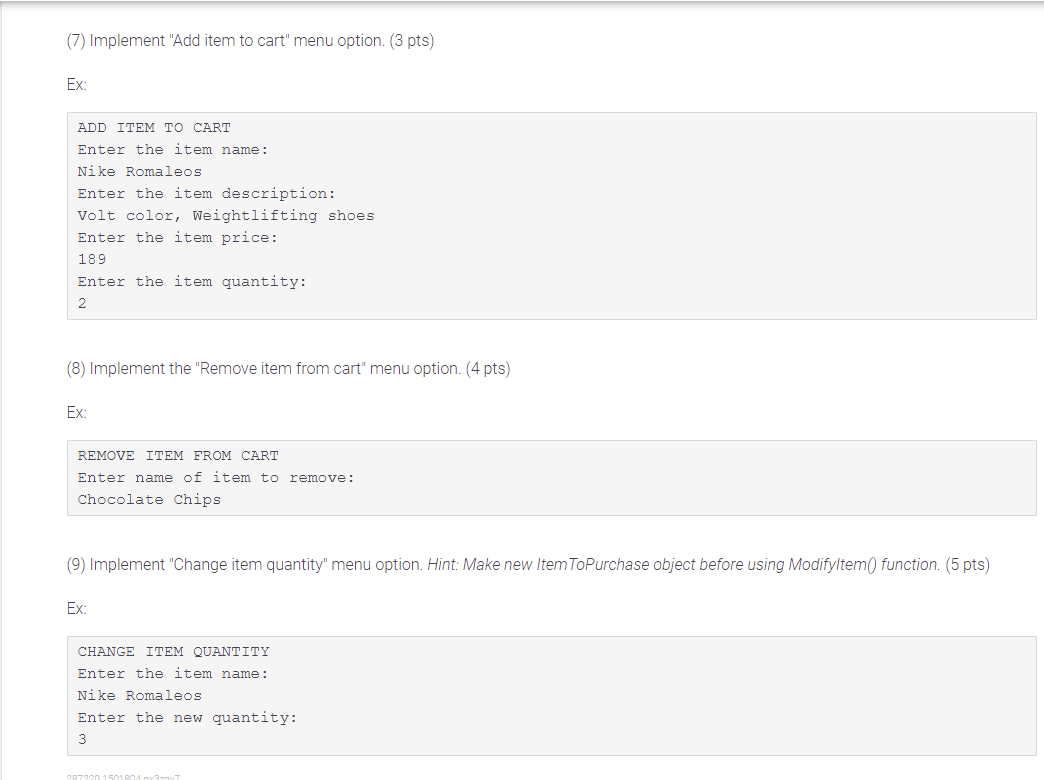
main c
#include #include "ItemToPurchase.h" #include "ShoppingCart.h"
void PrintMenu(ShoppingCart usrShopping) {
char myChar = ' ';
char c = ' ';
while (myChar != 'q') {
printf("MENU "); printf("a - Add item to cart "); printf("r - Remove item from cart "); printf("c - Change item quantity "); printf("i - Output items' descriptions "); printf("o - Output shopping cart "); printf("q - Quit ");
while (myChar != 'a' && myChar != 'r' && myChar != 'c' && myChar != 'i' && myChar != 'o' && myChar != 'q') {
printf("Choose an option: "); scanf(" %c", &myChar); }
if (myChar == 'a') {
while ((c = getchar()) != EOF && c != ' ');
char name[50]; char descr[50];
ItemToPurchase item;
printf("ADD ITEM TO CART ");
printf("Enter the item name: ");
//fgets(item.itemName, 50, stdin); fgets(name, 50, stdin);
int i = 0; while (name[i] != ' ') { item.itemName[i] = name[i]; ++i; } item.itemName[i] = '\0';
printf("Enter the item description: "); //fgets(item.itemDescription, 50, stdin); fgets(descr, 50, stdin);
i = 0; while (descr[i] != ' ') { item.itemDescription[i] = descr[i]; ++i; } item.itemDescription[i] = '\0';
printf("Enter the item price: "); scanf("%d", &item.itemPrice); printf("Enter the item quantity: "); scanf("%d", &item.itemQuantity);
usrShopping = AddItem(item, usrShopping);
printf(" "); myChar = ' ';
} else if (myChar == 'r') {
while ((c = getchar()) != EOF && c != ' ');
char temp[50]; char tempStr[50];
printf("REMOVE ITEM FROM CART ");
printf("Enter name of item to remove: ");
fgets(temp, 50, stdin);
int i = 0; while (temp[i] != ' ') { tempStr[i] = temp[i]; ++i; } tempStr[i] = '\0';
usrShopping = RemoveItem(tempStr, usrShopping);
myChar = ' ';
} else if (myChar == 'c') {
while ((c = getchar()) != EOF && c != ' ');
char temp[50]; char tempStr[50];
printf("CHANGE ITEM QUANTITY ");
printf("Enter the item name:"); fgets(temp, 50, stdin);
int i = 0; while (temp[i] != ' ') { tempStr[i] = temp[i]; ++i; } tempStr[i] = '\0';
usrShopping = ModifyItem(tempStr, usrShopping);
myChar = ' ';
} else if (myChar == 'i') { printf("OUTPUT ITEMS' DESCRIPTIONS "); PrintDescriptions(usrShopping); myChar = ' ';
} else if (myChar == 'o') { printf("OUTPUT SHOPPING CART "); PrintTotal(usrShopping); myChar = ' ';
}
} }
int main() {
ShoppingCart usrShopping; usrShopping.cartSize = 0;
printf("Enter Customer's Name: "); gets(usrShopping.customerName); printf("Enter Today's Date: "); gets(usrShopping.currentDate);
printf("Customer Name: %s ", usrShopping.customerName); printf("Today's Date: %s ", usrShopping.currentDate);
PrintMenu(usrShopping);
return 0; }
SHOPPING CART C
#include "ShoppingCart.h" #include #include
ShoppingCart AddItem(ItemToPurchase item, ShoppingCart cart) { cart.cartItems[cart.cartSize] = item; cart.cartSize = cart.cartSize + 1; return cart; }
ShoppingCart RemoveItem(char name[], ShoppingCart cart) { int i = 0; char itemFound = 'n';
for (i = 0; i
if (strcmp(name, cart.cartItems[i].itemName) == 0) {
itemFound = 'y'; for (int j = i; j
if (itemFound == 'y') { cart.cartSize = cart.cartSize - 1; }
if (itemFound == 'n') { printf("Item not found in cart. Nothing removed. "); } return cart;
}
ShoppingCart ModifyItem(char item[50], ShoppingCart cart) { int quantity; char itemFound = 'n';
printf("Enter the new quantity:"); scanf("%d", &quantity);
int i = 0; for (i = 0; i
if (strcmp(item, cart.cartItems[i].itemName) == 0) {
itemFound = 'y'; cart.cartItems[i].itemQuantity = quantity; } } if (itemFound == 'n') { printf("Item not found in cart. Nothing modified."); } return cart;
}
int GetNumItemsInCart(ShoppingCart cart) { return cart.cartSize; }
int GetCostOfCart(ShoppingCart cart) { int total = 0; int temp = 0;
for (int i = 0; i
void PrintTotal(ShoppingCart cart) { int total = 0; int numOfItems = 0;
for (int i = 0; i
printf("%s\'s Shopping Cart - %s ", cart.customerName, cart.currentDate); printf("Number of Items: %d ", numOfItems);
if (cart.cartSize == 0) { printf("SHOPPING CART IS EMPTY "); printf("Total: $0 ");
} else { for (int i = 0; i
void PrintDescriptions(ShoppingCart cart) {
printf("%s's Shopping Cart - %s ", cart.customerName, cart.currentDate);
printf("Item Descriptions "); for (int i = 0; i
}
SHOPPING CARD H
#ifndef SHOPPING_CART_H #define SHOPPING_CART_H
#include "ItemToPurchase.h" typedef struct ShoppingCart_struct { char customerName [50]; char currentDate [50]; ItemToPurchase cartItems [10]; int cartSize; } ShoppingCart;
ShoppingCart AddItem(ItemToPurchase item, ShoppingCart cart); ShoppingCart RemoveItem(char name[], ShoppingCart cart);
ShoppingCart ModifyItem(char item[50], ShoppingCart cart);
int GetNumItemsInCart(ShoppingCart cart);
int GetCostOfCart(ShoppingCart cart);
void PrintTotal(ShoppingCart cart);
void PrintDescriptions(ShoppingCart cart);
#endif
ITEM TO PURCHASE C
#include "ItemToPurchase.h" #include #include
void MakeItemBlank(ItemToPurchase* item){ strcpy((*item).itemName, "none"); strcpy((*item).itemDescription, "none"); (*item).itemPrice=0; (*item).itemQuantity=0; }
// 1.2 void PrintItemCost(ItemToPurchase item){ printf("%s %d @ $%d = $%d ", item.itemName, item.itemQuantity, item.itemPrice, (item.itemPrice*item.itemQuantity)); }
void PrintItemDescription(ItemToPurchase item){ printf("%s: %s. ", item.itemName, item.itemDescription); }
ITEM TO PURCHASE h
#ifndef ITEM_TO_PURCHASE_H #define ITEM_TO_PURCHASE_H
typedef struct ItemToPurchase_struct { char itemName[50]; char itemDescription[50]; int itemPrice; int itemQuantity; } ItemToPurchase;
void MakeItemBlank(ItemToPurchase* item);
void PrintItemCost(ItemToPurchase item);
void PrintItemDescription(ItemToPurchase item);
#endif
(I NEED SOMEONE TO HELP ME FIX MY CODE IT HAS SPACES IN PLACES IT DOESNT CORRESPOND, I NEED IT BY 11 central time, IF you cant PLEASE I NEED IT )
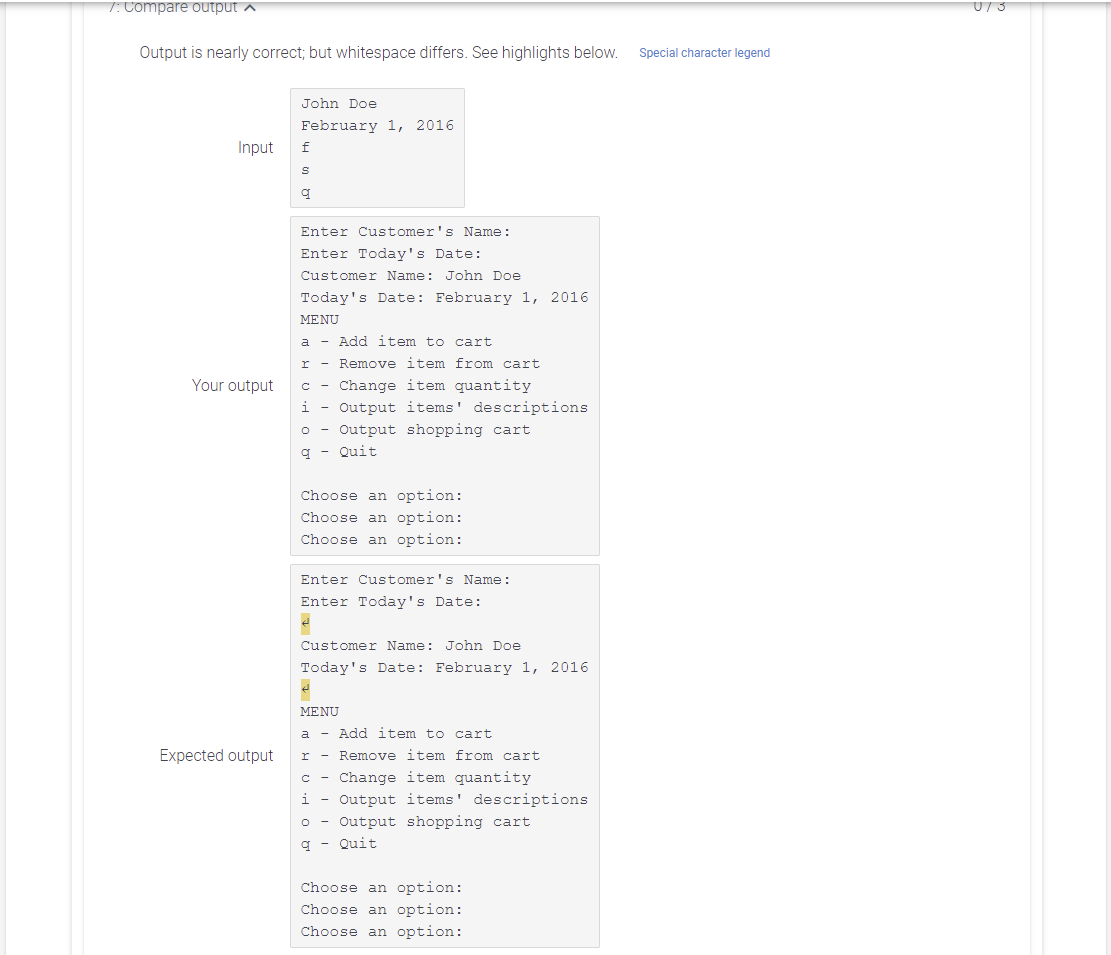
8: Compare output 073 Output differs. See highlights below. Special character legend John Doe February 1, 2016 Input O a OUTPUT SHOPPING CART John Doe's Shopping Cart Number of Items: 0 February 1, 2016 Your output ends with SHOPPING CART IS EMPTY Total: $0 MENU a Add item to cart r - Remove item from cart C - Change item quantity i Output items' descriptions 0 - Output shopping cart q Quit Choose an option: OUTPUT SHOPPING CART John Doe's Shopping Cart Number of Items: 0 February 1, 2016 SHOPPING CART IS EMPTY Total: $0 Expected output ends with r MENU Add item to cart Remove item from cart Change item quantity i - Output items' descriptions O - Output shopping cart q - Quit Choose an option: 9.8 LAB*: Program: Online shopping cart (Part 2) This program extends the earlier "Online shopping cart" program. (Consider first saving your earlier program) (1) Extend the ItemToPurchase struct to contain a new data member. (2 pt) char item Description[ ] - set to "none" in Makeltem Blanko Implement the following related functions for the Item ToPurchase struct. PrintItem Description o Has an Item ToPurchase parameter Ex. of PrintItem Description() output: Bottled Water: Deer Park, 12 oz. (2) Create three new files: Shopping Carth-struct definition and related function declarations Shopping Cart.c-related function definitions main.c- main() function (Note: main()'s functionality differs from the warm up) Build the Shopping Cart struct with the following data members and related functions. Note: Some can be function stubs (empty functions) initially, to be completed in later steps. Data members (3 pts) o char customerName [] o char current Date [] o ItemToPurchase cartItems [] - has a maximum of 10 slots (can hold up to 10 items of any quantity) o int cartSize - the number of filled slots in array cartitems [] (number of items in cart of any quantity) Related functions o AddItem Adds an item to cartItems array. Has parameters Item ToPurchase and Shopping Cart. Returns Shopping Cart object. o Removeltemo Removes item from cartitems array (does not just set quantity to 0; removed item will not take up a slot in array). Has a char[lan item's name) and a Shopping Cart parameter. Returns Shopping Cart object. If item name cannot be found output this message: Item not found in cart. Nothing removed. o Modifyltem If item name cannot be found output this message: Item not found in cart. Nothing removed. o Modifyltem Modifies an item's description, price, and/or quantity. Has parameters Item To Purchase and Shopping Cart. Returns Shopping Cart object. o GetNumItemsInCart((2 pts) Returns quantity of all items in cart. Has a Shopping Cart parameter. o GetCostOfCart0 (2 pts) Determines and returns the total cost of items in cart. Has a Shopping Cart parameter. o Print Total Outputs total of objects in cart. Has a Shopping Cart parameter. If cart is empty, output this message: SHOPPING CART IS EMPTY o Print Descriptions Outputs each item's description. Has a Shopping Cart parameter. Ex. of PrintTotal output: John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128 Total: $ 521 Ex. of PrintDescriptions() output: John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats Headphones: Bluetooth headphones (3) In main, prompt the user for a customer's name and today's date. Output the name and date. Create an object of type Shopping Cart (1 pt) Ex: Enter Customer's Name: John Doe (3) In main, prompt the user for a customer's name and today's date. Output the name and date. Create an object of type Shopping Cart (1 pt) Ex: Enter Customer's Name: John Doe Enter Today's Date: February 1, 2016 Customer Name: John Doe Today's Date: February 1, 2016 (4) Implement the PrintMenu() function. PrintMenu() has a Shopping Cart parameter, and outputs a menu of options to manipulate the shopping cart. Each option is represented by a single character. Build and output the menu within the function. If the an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. Call PrintMenu() in the main function. Continue to execute the menu until the user enters q to Quit. (3 pts) Ex: MENU a - Add item to cart r - Remove item from cart C - Change item quantity i - Output items' descriptions - Output shopping cart q - Quit Choose an option: (5) Implement the "Output shopping cart" menu option. (3 pts) Ex: OUTPUT SHOPPING CART John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats Headphones 1 @ $128 = $128 (5) Implement the "Output shopping cart' menu option. (3 pts) Ex OUTPUT SHOPPING CART John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats Headphones 1 @ $128 = $128 Total: $521 (6) Implement the "Output item's description" menu option. (2 pts) Ex: OUTPUT ITEMS' DESCRIPTIONS John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate chips: Semi-sweet Powerbeats Headphones: Bluetooth headphones (7) Implement "Add item to cart" menu option. (3 pts) Ex: ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2 (7) Implement "Add item to cart" menu option. (3 pts) Ex: ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2 (8) Implement the "Remove item from cart" menu option. (4 pts) Ex: REMOVE ITEM FROM CART Enter name of item to remove: Chocolate Chips (9) Implement "Change item quantity" menu option. Hint: Make new Item To Purchase object before using Modifyltem() function. (5 pts) Ex: CHANGE ITEM QUANTITY Enter the item name: Nike Romaleos Enter the new quantity: 3 7: Compare output A U73 Output is nearly correct; but whitespace differs. See highlights below. Special character legend John Doe February 1, 2016 f Input S a Enter customer's Name : Enter Today's Date: Customer Name: John Doe Today's Date: February 1, 2016 MENU a - Add item to cart r - Remove item from cart - Change item quantity i Output items' descriptions 0 - Output shopping cart q - Quit Your output Choose an option: Choose an option: Choose an option: Enter customer's Name: Enter Today's Date: Customer Name: John Doe Today's Date: February 1, 2016 Expected output MENU a - Add item to cart r - Remove item from cart c - change item quantity i - Output items' descriptions 0 - Output shopping cart q - Quit Choose an option: Choose an option: Choose an option