Question
main: //************************************************************************************************************** // CLASS: Main // // DESCRIPTION // The Main class for Project 2. // // AUTHOR // Kevin R. Burger (burgerk@asu.edu) // Computer
main:
//************************************************************************************************************** // CLASS: Main // // DESCRIPTION // The Main class for Project 2. // // AUTHOR // Kevin R. Burger (burgerk@asu.edu) // Computer Science & Engineering // School of Computing, Informatics, and Decision Systems Engineering // Fulton Schools of Engineering // Arizona State University, Tempe, AZ 85287-8809 // Web: http://www.devlang.com //************************************************************************************************************** Main.java
package tuition;
//import required packages import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Scanner;
//define the class Main
public class Main {
private ArrayList
/** * * Instantiate a Main object and call run() on the object. * */ public static void main(String[] args) {
Main m = new Main();
m.run();
}
/** * * Calculates the tuition for each student. * * Write an enhanced for loop that iterates over each Student in * * pStudentList. For each Student, call calcTuition() on that Student. * * Note: this is a polymorphic method * * call. * * * * PSEUDOCODE * * EnhancedFor each student in pStudentList Do * * student.calcTuition() * * End EnhancedFor * */ @SuppressWarnings("unused")
private void calcTuition(ArrayList
for (Student s : StudentObj) {
s.calcTuition();
}
}
/** * * Reads the student information from "p02-students.txt" and returns the * list of students as an ArrayList * * object. * * * * PSEUDOCODE * * Declare and create an ArrayList object named studentList. * * Open "p02-students.txt" for reading using a Scanner object named in. * * While in.hasNext() returns true Do * * String studentType <- read next string from in * * If studentType is "C" Then * * studentList.add(readOnCampusStudent(in)) * * Else * * studentList.add(readOnlineStudent(in)) * * End If * * End While * * Close the scanner * * Return studentList * */ @SuppressWarnings("unused")
private ArrayList readFile() throws FileNotFoundException {
ArrayList
File file = new File("p02-students.txt");
try {
Scanner in = new Scanner(file);
while (in.hasNext()) {
String studentType = in.next();
if (studentType.equals("C")) {
studentList.add(readOnCampusStudent(in));
} else {
studentList.add(readOnlineStudent(in));
}
}
in.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
return studentList;
}
/** * * Reads the information for an on-campus student. * * PSEUDOCODE * * Declare String object id and assign pIn.next() to id * * Declare String object named lname and assign pIn.next() to lname * * Declare String object named fname and assign pIn.next() to fname * * Declare and create an OnCampusStudent object. Pass id, fname, and lname * as params to ctor. * * Declare String object named res and assign pIn.next() to res * * Declare double variable named fee and assign pIn.nextDouble() to fee * * Declare int variable named credits and assign pIn.nextInt() to credits * * If res.equals("R") Then * * Call setResidency(true) on student * * Else * * Call setResidency(false) on student * * End If * * Call setProgramFee(fee) on student * * Call setCredits(credits) on student * * Return student * */ private OnCampusStudent readOnCampusStudent(Scanner pIn) {
String id = pIn.next();
String lname = pIn.next();
String fname = pIn.next();
Student onCampusStudent = new OnCampusStudent(id, fname, lname);
String res = pIn.next();
double fee = pIn.nextDouble();
int credits = pIn.nextInt();
if (res.equals("R")) {
((OnCampusStudent) onCampusStudent).setResidency(true);
} else {
((OnCampusStudent) onCampusStudent).setResidency(false);
}
((OnCampusStudent) onCampusStudent).setProgramFee(fee);
onCampusStudent.setCredits(credits);
return (OnCampusStudent) onCampusStudent;
}
/** * * Reads the information for an online student. * * * * PSEUDOCODE * * Declare String object id and assign pIn.next() to id * * Declare String object named lname and assign pIn.next() to lname * * Declare String object named fname and assign pIn.next() to fname * * Declare and create an OnlineStudent object. Pass id, fname, lname as * params to the ctor., * * Declare String object named fee and assign pIn.next() to fee * * Declare int variable named credits and assign pIn.nextInt() to credits * * If fee.equals("T")) Then * * Call setTechFee(true) on student * * Else * * Call setTechFee(false) on student * * End If * * Call setCredits(credits) on student * * Return student * */ private OnlineStudent readOnlineStudent(Scanner pIn) {
String id = pIn.next();
String lname = pIn.next();
String fname = pIn.next();
Student onlineStudent = new OnlineStudent(id, fname, lname);
String fee = pIn.next();
int credits = pIn.nextInt();
if (fee.equals("T")) {
((OnlineStudent) onlineStudent).setTechFee(true);
} else {
((OnlineStudent) onlineStudent).setTechFee(false);
}
onlineStudent.setCredits(credits);
return (OnlineStudent) onlineStudent;
}
/** * * Calls other methods to implement the sw requirements. * * * * PSEUDOCODE * * Declare ArrayList object named studentList * * try * * studentList = readFile() * * calcTuition(studentList) * * Call Sorter.insertionSort(studentList, Sorter.SORT_ASCENDING) to sort the * list * * writeFile(studentList) * * catch FileNotFoundException * * Print "Sorry, could not open 'p02-students.txt' for reading.Stopping." * * Call System.exit(-1) * */ private void run() {
Scanner infile;
ArrayList
try {
infile = new Scanner(new File("p02-students.txt"));
while (infile.hasNext()) {
String type = infile.next();
if (type.equals("C")) {
OnCampusStudent ocs = new OnCampusStudent(infile.next(), infile.next(), infile.next());
ocs.setResidency(infile.next().equals("R"));
ocs.setProgramFee(infile.nextDouble());
ocs.setCredits(infile.nextInt());
ocs.calcTuition();
studs.add(ocs);
} else if (type.equals("O")) {
OnlineStudent ols = new OnlineStudent(infile.next(), infile.next(), infile.next());
ols.setTechFee(infile.next().equals("T"));
ols.setCredits(infile.nextInt());
ols.calcTuition();
studs.add(ols);
}
}
infile.close();
//Sorter sorter = new Sorter(); Sorter.insertionSort(studs, 0);
PrintWriter writer = new PrintWriter("p02-tuition.txt");
Student s;
for (int i = 0; i < studs.size(); i++) {
s = studs.get(i);
writer.printf("%-15s %-12s %-12s %8.2f ", s.getId(), s.getFname(), s.getLname(), s.getTuition());
}
writer.close();
System.out.println("created output file p02-tuition.txt");
} catch (FileNotFoundException e) {
System.out.println("Sorry, could not " + "open 'p02-students.txt' for reading. Stopping.");
}
}
/** * * Writes the output file to "p02-tuition.txt" per the software * requirements. * * PSEUDOCODE * * Declare and create a PrintWriter object named out. Open " * * p02-tuition.txt" for writing. * * EnhancedFor each student in pStudentList Do * * out.print(student id + " " + student last name + " " + student first * * name) * * out.printf("%.2f%n" student tuition) * * End EnhancedFor * * Close the output file * */ @SuppressWarnings("unused")
private void writeFile(ArrayList
File file = new File("p02-tuition.txt");
PrintWriter out = new PrintWriter(file);
for (Student s : pStudentList) {
out.print(s.getId() + " " + s.getLname() + " " + s.getLname());
out.printf("%.2f%n", s.getTuition());
}
out.close();
}
}
OnCampusStudent
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package student;
/** * * @author thndr */ public class OnCampusStudent extends Student {
//declare variables private boolean mResident;
private double mProgramFee;
//constuctor public OnCampusStudent(String id, String fname, String lname) {
//call the variables through super method super(id, fname, lname);
}
//accessor and mutator methods public void setResidency(boolean residency) {
mResident = residency;
}
public boolean getResidency() {
return mResident;
}
public void setProgramFee(double fee) {
mProgramFee = fee;
}
public double getProgramFee() {
return mProgramFee;
}
//Implement the method @Override
public void calcTuition() {
double t;
if (getResidency() == true) {
t = TuitionConstants.ONCAMP_RES_BASE;
} else {
t = TuitionConstants.ONCAMP_NONRES_BASE;
t = t + getProgramFee();
}
if (getCredits() > TuitionConstants.MAX_CREDITS) {
t = t + (getCredits() - TuitionConstants.MAX_CREDITS) * TuitionConstants.ONCAMP_ADD_CREDITS;
}
setTuition(t);
}
}
OnlineStudent
/* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package student;
/** * * @author thndr */ public class OnlineStudent extends Student {
//declare the variables private boolean mTechFee;
//Constructor public OnlineStudent(String id, String fname, String lname) {
super(id, fname, lname);
}
//accessor and mutato methods public boolean getTutionFee() {
return mTechFee;
}
public void setTechFee(boolean techfee) {
mTechFee = techfee;
}
//implemen the tution method @Override
public void calcTuition() {
double t = getCredits() * TuitionConstants.ONLINE_CREDIT_RATE;
if (getTutionFee() == true) {
t = t + TuitionConstants.ONLINE_TECH_FEE;
}
setTuition(t);
}
}
Sorter
//************************************************************************************************************** // CLASS: Sorter // // DESCRIPTION // Implements the insertion sort algorithm to sort an ArrayList<> of Students. // // AUTHOR // Kevin R. Burger (burgerk@asu.edu) // Computer Science & Engineering Program // Fulton Schools of Engineering // Arizona State University, Tempe, AZ 85287-8809 // http:www.devlang.com //************************************************************************************************************** package tuition;
import java.util.ArrayList;
public class Sorter {
public static final int SORT_ASCENDING = 0; public static final int SORT_DESCENDING = 1;
/** * Sorts pList into ascending (pOrder = SORT_ASCENDING) or descending * (pOrder = SORT_DESCENDING) order using the insertion sort algorithm. */ public static void insertionSort(ArrayList
/** * Returns true if we need to continue moving the element at pIndex until it * reaches its proper location. */ private static boolean keepMoving(ArrayList
/** * Swaps the elements in pList at pIndex1 and pIndex2. */ private static void swap(ArrayList
}
Student
//************************************************************************************************** // CLASS: Student //************************************************************************************************** package tuition;
public abstract class Student implements Comparable
//Decalre the variables private int mCredits;
private String mId;
private String mLname;
private String mFname;
private double mTuition;
//constructor public Student(String Id, String Fname, String Lname) {
mId = Id;
mFname = Fname;
mLname = Lname;
}
//accessor and mutator methods public int getCredits() {
return mCredits;
}
public void setCredits(int Credits) {
this.mCredits = Credits;
}
public String getId() {
return mId;
}
public void setId(String Id) {
this.mId = Id;
}
public String getLname() {
return mLname;
}
public void setLname(String Lname) {
this.mLname = Lname;
}
public String getFname() {
return mFname;
}
public void setmFname(String Fname) {
this.mFname = Fname;
}
public double getTuition() {
return mTuition;
}
protected void setTuition(double tuition) {
mTuition = tuition;
}
public abstract void calcTuition();
@Override
public int compareTo(Student pStudent) {
return getId().compareTo(pStudent.getId());
}
}
TutionConstants
//************************************************************************************************************** // CLASS: TuitionConstants // // DESCRIPTION // Constants that are used in calculating the tuition for on-campus and online students. Use these constants // in the OnCampusStudent and OnlineStudent classes. // // AUTHOR // Kevin R. Burger (burgerk@asu.edu) // Computer Science & Engineering // School of Computing, Informatics, and Decision Systems Engineering // Fulton Schools of Engineering // Arizona State University, Tempe, AZ 85287-8809 // Web: http://www.devlang.com //************************************************************************************************************** package tuition;
public class TuitionConstants {
public static final int ONCAMP_ADD_CREDITS = 350; public static final int MAX_CREDITS = 18; public static final int ONCAMP_NONRES_BASE = 12200; public static final int ONCAMP_RES_BASE = 5500; public static final int ONLINE_CREDIT_RATE = 875; public static final int ONLINE_TECH_FEE = 125;
}
P02-students.txt
C 8230123345450 Flintstone Fred R 0 12 C 3873472785863 Simpson Lisa N 750 18 C 4834324308675 Jetson George R 0 20 O 1384349045225 Szyslak Moe - 6 O 5627238253456 Flanders Ned T 3
p02-tuition.txt
C 8230123345450 Flintstone Fred R 0 12 C 3873472785863 Simpson Lisa N 750 18 C 4834324308675 Jetson George R 0 20 O 1384349045225 Szyslak Moe - 6 O 5627238253456 Flanders Ned T 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
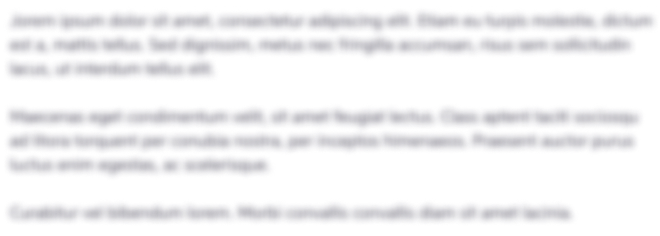
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started