Question
MainActivity.java import android.support.v4.view.ViewPager; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.TableLayout; public class MainActivity extends AppCompatActivity { private TabLayout tabLayout; ViewPagerAdapter viewPagerAdapter; //This is our viewPager private
MainActivity.java import android.support.v4.view.ViewPager; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.TableLayout;
public class MainActivity extends AppCompatActivity { private TabLayout tabLayout; ViewPagerAdapter viewPagerAdapter; //This is our viewPager private ViewPager viewPager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); viewPager = (ViewPager) findViewById(R.id.pager); viewPagerAdapter = new ViewPagerAdapter(getSupportFragmentManager()); viewPager.setAdapter(viewPagerAdapter); tabLayout = (TabLayout) findViewById(R.id.tabLayout); tabLayout.setupWithViewPager(viewPager); } }
activity_main.xml
ViewPagerAdapter.java
package com.example.rns_2.movie;
import android.support.v4.app.Fragment; import android.support.v4.app.FragmentManager; import android.support.v4.app.FragmentPagerAdapter;
/** * Created by RNS-2 on 10/8/2017. */
public class ViewPagerAdapter extends FragmentPagerAdapter {
public ViewPagerAdapter(FragmentManager fm) { super(fm); }
@Override public Fragment getItem(int position) { Fragment fragment = null; if (position == 0) { fragment = new MovieFragment(); } else if (position == 1) { fragment = new Games(); } else if (position == 2) { fragment = new Songs(); } return fragment; }
@Override public int getCount() { return 3; }
@Override public CharSequence getPageTitle(int position) { String title = null; if (position == 0) { title = "Movie"; } else if (position == 1) { title = "Tab-2"; } else if (position == 2) { title = "Tab-3"; } return title; } }
MovieFragment.java package com.example.rns_2.movie;
import android.os.Bundle; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup;
/** * A simple {@link Fragment} subclass. */ public class MovieFragment extends Fragment {
public MovieFragment() { // Required empty public constructor }
@Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { // Inflate the layout for this fragment return inflater.inflate(R.layout.fragment_movie, container, false); }
} fragment_movie.xml
/> >> Question : Task 0: Front-page Activity (5 points). You can reuse the one built from the last homework. You need to build an Activity (called front page), which loads a front-page Fragment. Inside this Fragment, you need to have the following buttons (you can decide the layout and feel free to add other decoration items): About me button: when it is clicked, your about me Fragment will be loaded. You need to change the orientation of your device to check whether it works properly. A button for Task 1: when it is clicked, the corresponding Activity will come up (same operation with the menu on the Action Bar or App Bar). Task 1 (80): Please load the movie data into a RecyclerView. Each row of the RecyclerView should contain at least the following information: the image of movie, title, description, and a CheckBox. You can design your own layout. There should be four buttons above the RecyclerView. You need to implement the following functionalities: 1. (20) Get the RecyclerView to work; each row should be a CardView. 2. (5) When the CheckBox is clicked, its status will be toggled. However, when the row is clicked, the CheckBox will not be toggled. 3. (5) When the Select All button is clicked, all the CheckBoxes will be checked. When the Clear All button is clicked, all the CheckBoxes will be unchecked. 4. (5) When the Delete button is clicked, all the selected movies will be deleted from the list. Users should be able to see the animation effect. 5. (10) When a list item is long clicked, that item will be duplicated and placed right below the current item (i.e., a new movie item with duplicated content will appear). Users should be able to see the animation effect. 6. (10) When a list item is clicked, a Fragment will be loaded into the current activity, displaying the details of the selected movie. 7. (5) When the phones orientation changes, the selected movies should stay selected. 8. (10) When the "Sort" button is clicked, all movies will be sorted by the release (the most recent movie should be the top of the list). The top 5 movies and the bottom 5 movies should have different layouts from other middle movies (There should be 3 layouts for each group). 9. (5) Try an Item animation from wasabeef library https://github.com/wasabeef/recyclerview-animators 10. (5) Try an adapter animation from wasabeef library * Tips: You have handle your MovieData class to copy(insert duplicate item)/delete/set or reset checkbox. Whenever data is changed, you have to notify to RecyclerViewAdapter * You don't need to create Tablet or landscape
Step by Step Solution
There are 3 Steps involved in it
Step: 1
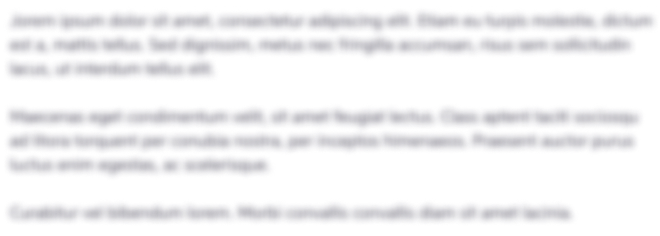
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started