Question
main.c ------------------------------------------------ #include global.h void main() { init(); parse(); exit(0); } -------------------------------------------------- emitter.c ------------------------------ #include global.h void emit(int t, int tval) { switch (t) {
main.c ------------------------------------------------ #include "global.h"
void main() {
init(); parse(); exit(0); } -------------------------------------------------- emitter.c ------------------------------ #include "global.h"
void emit(int t, int tval) { switch (t) { case '+': fprintf(fOutput,"pop r1 pop r2 add r2,r1 push r2 "); break;
case '-': fprintf(fOutput,"pop r1 pop r2 sub r2,r1 push r2 "); break;
case '*': fprintf(fOutput,"pop r1 pop r2 mult r2,r1 push r2 "); break;
case '/': fprintf(fOutput,"pop r1 pop r2 Rdiv r2,r1 push r2 "); break;
case DIV: fprintf(fOutput,"pop r1 pop r2 Div r2,r1 push r2 "); break;
case MOD: fprintf(fOutput,"pop r1 pop r2 Mod r2,r1 push r2 "); break;
case NUM: fprintf(fOutput,"push %d ", tval); break;
case ID: fprintf(fOutput,"push %s ", symtable[tval].lexptr); break;
default: fprintf(fOutput,"token %d, tokenval %d ", t, tval); }
} ----------------------------------------------------------------------- global.h -------------------------------------------- #ifndef global
#include
FILE *fInput; FILE *fOutput; FILE *fError;
#define BSIZE 128 #define NONE -1 #define EOS '\0'
#define NUM 256 #define DIV 257 #define MOD 258 #define ID 259 #define DONE 260
#define STRMAX 999 #define SYMMAX 100
int lookahead; int tokenval; int lineno;
struct entry { char *lexptr; int token; };
struct entry symtable[SYMMAX];
void init(); int lookup(char s[]); int insert(char s[], int tok); void error(char* m); void parse(); void expr(); void term(); void factor(); void match(int t); int lexan(); void emit(int t, int tval);
#endif // !global -------------------------------------------------------------------------- Init.c ---------------------------------- #include "global.h"
struct entry keywords[] = { "div", DIV, "mod", MOD, 0, 0 };
void init() { fOutput = fopen("output.obj", "w"); fInput = fopen("input.exp", "r"); fError = fopen("error.err", "w");
struct entry *p; for (p = keywords; p->token; p++) insert(p->lexptr, p->token); } --------------------------------------------------------------------------------- lexer.c --------------------------------------------- #include "global.h"
char lexbuf[BSIZE]; int lineno = 1; int tokenval = NONE;
int lexan() {
int t;
while (1) { t = getc(fInput); if (t == ' ' || t == '\t'); else if (t == ' ') lineno = lineno + 1; else if (isdigit(t)) { ungetc(t, fInput); fscanf(fInput,"%d", &tokenval); return NUM; }
else if (isalpha(t)) { int p, b = 0; while (isalnum(t)) { lexbuf[b] = t; t = getc(fInput); b = b + 1; if (b >= BSIZE) error("compiler error"); } lexbuf[b] = EOS; if (t != EOF) ungetc(t, fInput); p = lookup(lexbuf); if (p == 0) p = insert(lexbuf, ID); tokenval = p; return symtable[p].token; } else if (t == EOF) return DONE; else { tokenval = NONE; return t; } } } ------------------------------------------------------------------------------------ parse.c ----------------------------------------------- #include"global.h" int lookahead; void parse() { lookahead = lexan(); while (lookahead != DONE) { expr(); fprintf(fOutput," Done "); match(';'); } }
void expr() { int t; term();
while (1) switch (lookahead) { case '+': case '-': t = lookahead; match(lookahead); term(); emit(t, NONE); continue; default: return; } }
void term() { int t; factor(); while (1) switch (lookahead) { case '*': case '/': case DIV: case MOD: t = lookahead; match(lookahead); factor(); emit(t, NONE); continue; default: return; } }
void factor() { switch (lookahead) { case '(': match('('); expr(); match(')'); break; case NUM: emit(NUM, tokenval); match(NUM); break; case ID: emit(ID, tokenval); match(ID); break; default: error("syntax error"); } }
void match(int t) { if (lookahead == t) lookahead = lexan(); else error("syntax error"); } ------------------------------------------------------------------------------------ symbol.c ----------------------------------------- #include "global.h"
#define STRMAX 999 #define SYMMAX 100
char lexemes[STRMAX]; int lastchar = -1; struct entry symtable[SYMMAX]; int lastentry = 0;
int lookup(char s[])
{ int p; for (p = lastentry; p > 0; p = p - 1) if (strcmp(symtable[p].lexptr, s) == 0) return p;
return 0; }
int insert(char s[], int tok)
{ int len; len = strlen(s);
if (lastentry + 1 >= SYMMAX) error("symbol table full");
if (lastchar + len + 1 >= STRMAX) error("lexemes array full");
lastentry = lastentry + 1;
symtable[lastentry].token = tok;
symtable[lastentry].lexptr = &lexemes[lastchar + 1];
lastchar = lastchar + len + 1;
strcpy(symtable[lastentry].lexptr, s);
return lastentry; } ------------------------------------------------------------------ error.c ----------------------------------- #include "global.h"
void error(char* m) { fprintf(fError, "line %d: %s ", lineno, m); exit(1); }
Based on the code of small compiler given above modify it to accept int and double data types. The int data type is accepted by the DFA: digit+ digit start digit other The double type is accepted by the DFA: (.digit+) ? (e|E) ( + | - ) ? digit* ? You can just reuse the code in Lecture 2: 1. The Lexical Analysis Module lexer.c The lexical analyzer is a routine called lexan( ) that is called by the parser to find tokens. 2. The Parser Module parser.c This module implements the above grammar. 3. The Emitter Module emitter.c The emitter module consists of a single function emit(t, tval) that generates the output for token t with attribute value tval. 4. The Symbol-Table Modules symbol.c The symbol-table module symbol.c implements the data structure shown in Section 2.7. 5. The module init.c is used to preload symbol table with keywords. 6. The Error Module error.c The error module manages the error reporting, which prints a message on the current input line and then halts. In this project, you should do: 1. Add int and double data types. 2. Add supporting float inputs. 3. Modified the code continuing even an error is detected. 4. Print out how many lines that parser checked and how many errors detected. For example, if the input file has the contents: int x, y = 1234; double z123, z1234=1.2345, adb = 12.34e20; x = 5*3+(7+8)*(9-2); y = wetrt123 + (9-4)/(3-2); z12345 = x mod 10 div (45-12345); abcde = dr123 - xr456/t987 * v345 + (u mod 7) + vbcd div 12345; g789 = abcde MOD 20 DIV 10; 123131;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
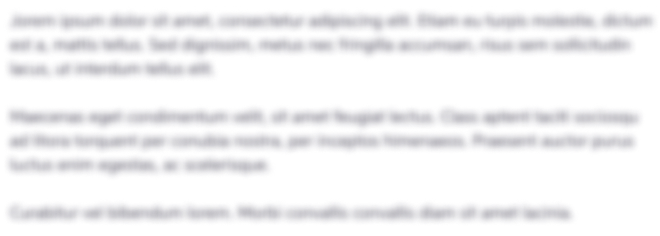
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started