Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
MainDriver_ReverseList.cpp // List Reverse #include /* cin, cout, */ #include /* srand, rand */ #include /* time */ #include IntList.h /* user defined list operations
MainDriver_ReverseList.cpp
// List Reverse #include/* cin, cout, */ #include /* srand, rand */ #include /* time */ #include "IntList.h" /* user defined list operations */ using namespace std; int main() { // # of nodes the users wants in the list int node_count; // Create an instance of IntList. IntList myList; // Build a static list. myList.appendNode(2); // Append 2 to the list myList.appendNode(4); // Append 4 to the list myList.appendNode(6); // Append 6 to the list // Display the nodes. cout > node_count; /* initialize random seed: */ srand (time(NULL)); for (int i=0; i
IntList.h
// Specification file for the IntList class #ifndef INTLIST_H #define INTLIST_H class IntList { private: // Declare a structure for the list struct ListNode { int value; struct ListNode *next; }; ListNode *head; // List head pointer // Destroy function void destroy(); public: // Constructor IntList() { head = NULL; } // Copy constructor IntList(const IntList &); // Destructor ~IntList(); // List operations void appendNode(int); void insertNode(int); void deleteNode(int); void print(); void reverse(); }; #endif
IntList.cpp
// Implementation file for the IntList class #include// For cout and NULL #include "IntList.h" using namespace std; //************************************************** // Copy constructor * //************************************************** IntList::IntList(const IntList &listObj) { ListNode *nodePtr; // Initialize the head pointer. head = NULL; // Point to the other object's head. nodePtr = listObj.head; // Traverse the other object. while (nodePtr) { // Append a copy of the other object's // node to this list. appendNode(nodePtr->value); // Go to the next node in the other object. nodePtr = nodePtr->next; } } //************************************************** // appendNode appends a node containing the * // value pased into num, to the end of the list. * //************************************************** void IntList::appendNode(int num) { ListNode *newNode, *nodePtr; // Allocate a new node & store num newNode = new ListNode; newNode->value = num; newNode->next = NULL; // If there are no nodes in the list // make newNode the first node if (!head) head = newNode; else // Otherwise, insert newNode at end { // Initialize nodePtr to head of list nodePtr = head; // Find the last node in the list while (nodePtr->next) nodePtr = nodePtr->next; // Insert newNode as the last node nodePtr->next = newNode; } } //************************************************** // The print function displays the value * // stored in each node of the linked list * // pointed to by head. * //************************************************** void IntList::print() { ListNode *nodePtr; nodePtr = head; while (nodePtr) { cout value next; } } //************************************************** // The insertNode function inserts a node with * // num copied to its value member. * //************************************************** void IntList::insertNode(int num) { ListNode *newNode, *nodePtr, *previousNode = NULL; // Allocate a new node & store num newNode = new ListNode; newNode->value = num; // If there are no nodes in the list // make newNode the first node if (!head) { head = newNode; newNode->next = NULL; } else // Otherwise, insert newNode { // Initialize nodePtr to head of list and // previousNode to NULL. nodePtr = head; previousNode = NULL; // Skip all nodes whose value member is less // than num. while (nodePtr != NULL && nodePtr->value next; } // If the new node is to be the 1st in the list, // insert it before all other nodes. if (previousNode == NULL) { head = newNode; newNode->next = nodePtr; } else // Otherwise, insert it after the prev node { previousNode->next = newNode; newNode->next = nodePtr; } } } //************************************************** // The deleteNode function searches for a node * // with num as its value. The node, if found, is * // deleted from the list and from memory. * //************************************************** void IntList::deleteNode(int num) { ListNode *nodePtr, *previousNode; // If the list is empty, do nothing. if (!head) return; // Determine if the first node is the one. if (head->value == num) { nodePtr = head->next; delete head; head = nodePtr; } else { // Initialize nodePtr to head of list nodePtr = head; // Skip all nodes whose value member is // not equal to num. while (nodePtr != NULL && nodePtr->value != num) { previousNode = nodePtr; nodePtr = nodePtr->next; } // If nodePtr is not at the end of the list, // link the previous node to the node after // nodePtr, then delete nodePtr. if (nodePtr) { previousNode->next = nodePtr->next; delete nodePtr; } } } void IntList::reverse() { ListNode *newHead = NULL, *newNode, *nodePtr, *tempPtr; // Traverse the list, building a // copy of it in reverse order. nodePtr = head; while (nodePtr) { // Allocate a new node & store the // value of the current list node in it. newNode = new ListNode; newNode->value = nodePtr->value; newNode->next = NULL; // Shift the existing nodes in the new // list down one, inserting the new // node at the top. if (newHead != NULL) { tempPtr = newHead; newHead = newNode; newNode->next = tempPtr; } else { newHead = newNode; } // Go to the next node in the list. nodePtr = nodePtr->next; } // Destroy the existing list. destroy(); // Make head point to the new list. head = newHead; } //************************************************** // The destroy function destroys all the nodes * // in the list. * //************************************************** void IntList::destroy() { ListNode *nodePtr, *nextNode; nodePtr = head; while (nodePtr != NULL) { nextNode = nodePtr->next; delete nodePtr; nodePtr = nextNode; } head = NULL; } //************************************************** // Destructor * // This function deletes every node in the list. * //************************************************** IntList::~IntList() { destroy(); } Problem Statement: Understand Linked list code by tracing the code that Reverses a linked list I have provided the solution to code that builds and reverses a linked list. You are to modify this code to build and keep a sorted list. Then you must trace the code to show how the list is being reversed. You must do the following Study the code to understand how to write code to randomly generate numbers and to use linked list code. Compile and execute the code. Enter the number "4" when asked in order to randomly generate 2 additional numbers. Capture a screenshot (ctrl-alt-printScreen) to capture the output Paste into a word document that has your name, date, course and instructor at the top right hand corner. Notice that the list is not sorted. Add an appropriate label above it (i.e. Unsorted Screen Capture) Now modify the code to create a sorted list and then reverse it. You should only need to change 4 lines of code in the "Driver" code Capture a screenshot (ctrl-alt-printScreen) to capture the output Paste it in the MS Word document after the first screenshot with an appropriate label above it (i.e. Sorted Screen Capture). Print the Word Document. Trace the linked list program code that you modified. Show memory locations (integer, link pointer) similar to how I showed in class. Use a separate sheet of paper to show your trace of the code. This should be drawn node representations. .3.cpp source code files uploaded to the link on blackboard * Word Document with screen capture should be uploaded to blackboard * Snapshot of trace work should be uploaded to blackboard . After all documents have been uploaded, click the submit buttorn Rubric 20 points: Code Changes 50 Points: Screen display capture 30 points: Show detailed hand-trace of linked list building, traversal, reversal of list ere are the values in nyList lease enter the nunber of values you would like to add to your list ere are the neu values in nylist: Reversing the lint Here are the valuei in nylist: 2 Problem Statement: Understand Linked list code by tracing the code that Reverses a linked list I have provided the solution to code that builds and reverses a linked list. You are to modify this code to build and keep a sorted list. Then you must trace the code to show how the list is being reversed. You must do the following Study the code to understand how to write code to randomly generate numbers and to use linked list code. Compile and execute the code. Enter the number "4" when asked in order to randomly generate 2 additional numbers. Capture a screenshot (ctrl-alt-printScreen) to capture the output Paste into a word document that has your name, date, course and instructor at the top right hand corner. Notice that the list is not sorted. Add an appropriate label above it (i.e. Unsorted Screen Capture) Now modify the code to create a sorted list and then reverse it. You should only need to change 4 lines of code in the "Driver" code Capture a screenshot (ctrl-alt-printScreen) to capture the output Paste it in the MS Word document after the first screenshot with an appropriate label above it (i.e. Sorted Screen Capture). Print the Word Document. Trace the linked list program code that you modified. Show memory locations (integer, link pointer) similar to how I showed in class. Use a separate sheet of paper to show your trace of the code. This should be drawn node representations. .3.cpp source code files uploaded to the link on blackboard * Word Document with screen capture should be uploaded to blackboard * Snapshot of trace work should be uploaded to blackboard . After all documents have been uploaded, click the submit buttorn Rubric 20 points: Code Changes 50 Points: Screen display capture 30 points: Show detailed hand-trace of linked list building, traversal, reversal of list ere are the values in nyList lease enter the nunber of values you would like to add to your list ere are the neu values in nylist: Reversing the lint Here are the valuei in nylist: 2
Step by Step Solution
There are 3 Steps involved in it
Step: 1
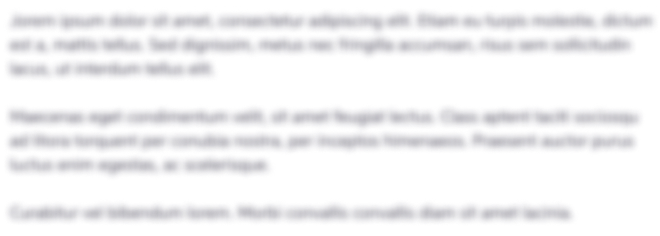
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started