Question
Main.java class Main { public static void main(String[] args) { ATM atm = new ATM(); atm.init(); atm.run(); } } ATM.java class ATM { private LCD
Main.java
class Main {
public static void main(String[] args) {
ATM atm = new ATM();
atm.init();
atm.run();
}
}
ATM.java
class ATM {
private LCD lcd;
private CardReader cardReader;
private Keypad keypad;
private CashDispenser cashDispenser;
public void init() {
System.out.println("ATM.init()");
lcd = new LCD();
lcd.init();
cardReader = new CardReader();
cardReader.init();
keypad = new Keypad();
keypad.init();
cashDispenser = new CashDispenser();
cashDispenser.init();
}
public void run() {
String BANK_NAME = "NewCo";
System.out.println("ATM Version 0.1.0");
System.out.println("Copyright (C) 2020 " + BANK_NAME);
System.out.println("Code by your name");
User user = new User();
while (true) {
user.io();
}
}
}
CardReader.java
class CardReader {
public void init() {
System.out.println("CardReader.init()");
}
}
CashDispenser.java
class CashDispenser {
private int num100s;
private int num20s;
private int num10s;
public void init() {
System.out.println("CashDispenser.init()");
}
public int getCash(int amount) {
num100s = amount / 100;
amount = amount % 100;
num20s = amount / 20;
amount = amount % 20;
num10s = amount / 10;
amount = amount % 10;
if (amount != 0) return -1;
return 0;
}
public String num100s() {
return "$100 Bills: " + num100s;
}
public String num20s() {
return "$20 Bills: " + num20s;
}
public String num10s() {
return "$10 Bills: " + num10s;
}
}
Keypad.java
import java.util.Scanner;
class Keypad {
public void init() {
System.out.println("Keypad.init()");
}
public int getAmount() {
Scanner scanner = new Scanner(System.in);
return scanner.nextInt();
}
}
LCD.java
class LCD {
public void init() {
System.out.println("LCD.init()");
}
public void print(String s) {
System.out.print(s);
}
}
User.java
class User {
private LCD lcd;
private CardReader cardReader;
private Keypad keypad;
private CashDispenser dispenser;
public User(LCD lcd, CardReader cardReader, Keypad keypad, CashDispenser dispenser) {
this.lcd = lcd;
this.cardReader = cardReader;
this.keypad = keypad;
this.dispenser = dispenser;
}
public void io() {
lcd.print("Welcome to ATM ");
lcd.print("Press ENTER to begin: ");
cardReader.readCard();
//Get amount to withdraw
lcd.print("Enter amount to withdraw: ");
int amount = keypad.getAmount();
// Withdraw the amount
int result = dispenser.getCash(amount);
if (result == -1) {
lcd.print("Cash dispense error%n");
}
else {
lcd.print("Cash dispense as follows: ");
lcd.print(dispenser.num100s() + " ");
lcd.print(dispenser.num20s() + " ");
lcd.print(dispenser.num10s() + " ");
}
}
}
Add code to the classes as needed to complete the ATM Machine.
Create Logger.java class that logs all ATM transactions to a file. Key features fo the Logger class should include:
Use of java.util.ArrayList to keep array of log entries. Each Log entry includes info such as:
- Date/Time of the entry
- Number of failed pin entry attempts
- Boolean showing if pin entered correctly
- Amount withdrawn
Write the Log Entry to a file named atm.log (using Object file format) after every ATM transaction
Create Config.java class that using java.util.HashMap to keep the following key/value pairs as String/String. The Config.java class should read in config info in XML format from a file named atm.xml, and use a java.util.Stack class to parse the atm.xml and confirm that it is in the correct format. The values parsed from the atm.xml should be put into a java.util.HashMap as key/value pairs.
- "
title to display on welcome screen goes here copyright info goes here developer info goes here - Later in your code the java.util.HashMap will provided needed values based on keys
Solution:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
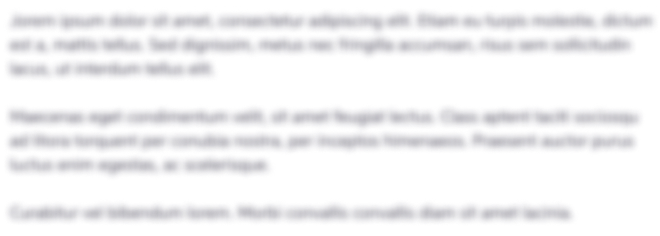
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started