Question
Maintaint Student Scores using c# Here is the code but it will not complie..not sure what is wrong... cant get it to display sample students
Maintaint Student Scores using c#
Here is the code but it will not complie..not sure what is wrong... cant get it to display sample students and average, score total, score count..
Assignment:
When this application starts, it should read student data from a text file named StudentScores.txt in the C:\\C#.NET\\Files directory and display this data in the Students list box. If this directory or file doesnt exist, it should be created.
When students are added this application should write the student data to the StudentScores.txt file, overwriting any existing data.
This application should use a class named StudentDB to read data from and write data to the StudentScores.txt file.
StudentDB.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace MaintainStudentScores_3
{
public static class StudentDB
{
private const string dir = @\"C:C#.NET\\Files\\\";
private const string path = dir + \"StudentScores.Txt\";
public static void SaveStudent(List students)
{
StreamWriter textOut =
new StreamWriter(
new FileStream(path, FileMode.Create, FileAccess.Write));
foreach (Student student in students)
{
string scoresList = \"\";
for (int i =0; i
{
int value = student.ScoreList[i];
scoresList += (value + \".\");
}
textOut.Write(student.Name + \"|\");
textOut.WriteLine(scoresList.TrimEnd(','));
}
textOut.Close();
}
public static List GetStudents()
{
if (Directory.Exists(dir))
Directory.CreateDirectory(dir);
StreamReader textIn = new StreamReader(new FileStream(path, FileMode.OpenOrCreate, FileAccess.Read));
List students = new List();
while(textIn.Peek() != -1)
{
string row = textIn.ReadLine();
string[] columns = row.Split('|');
Student student = new Student();
List scoreList = new List();
student.ScoreList = scoreList;
if(columns[1] != \"\")
{
int[] scoreArray = columns[1].Split(',').Select(n => Convert.ToInt32(n)).ToArray();
scoreList = scoreArray.ToList();
student.ScoreList = scoreList;
}
else
{
student.ScoreList = new List();
}
student.Name = columns[0];
students.Add(student);
}
textIn.Close();
return students;
}
public static void LoadSampleStudents()
{
if(!Directory.Exists(dir))
{
Directory.CreateDirectory(dir);
}
if(File.Exists(path))
{
StreamWriter textOut = new StreamWriter(new FileStream(path, FileMode.Create, FileAccess.Write));
textOut.WriteLine(\"Joel Murach|97,71,83\");
textOut.WriteLine(\"Doug Lowel|99,93,97\");
textOut.WriteLine(\"Anne Prince|100,100,100\");
textOut.Close();
}
}
}
}
Student.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MaintainStudentScores_3
{
public class Student
{
private string name;
private List scoreList;
public Student()
{
}
public Student(string name, List scoreList)
{
this.Name = name;
this.ScoreList = scoreList;
}
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
public List ScoreList
{
get
{
return scoreList;
}
set
{
scoreList = value;
}
}
public string GetDisplayText()
{
string total = \"\";
foreach (int score in scoreList)
{
total += \"|\" + score;
}
return name + total;
}
Validator.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MaintainStudentScores_3
{
public static class Validator
{
public static string title = \"Entry Error\";
public static bool IsPresent(TextBox textBox, string name)
{
if(textBox.Text == String.Empty)
{
MessageBox.Show(name + \"is a required field\", title);
textBox.Focus();
return false;
}
return true;
}
public static bool IsInt32(TextBox textBox, string name)
{
int number = 0;
if(Int32.TryParse(textBox.Text, out number))
{
return true;
}
else
{
MessageBox.Show(name + \" must be an integer\", title);
textBox.Focus();
return false;
}
}
public static bool IsWithinRange(TextBox textBox, string name, int min, int max)
{
int number = Convert.ToInt32(textBox.Text);
if(number max)
{
MessageBox.Show(name + \" must be between\" + min + \" and\" + max + \".\", title);
textBox.Focus();
return false;
}
return true;
}
}
}
maintStudentScores.cs //main form
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MaintainStudentScores_3
{
public partial class maintStudentScores : Form
{
private List students = null;
public maintStudentScores()
{
InitializeComponent();
}
private void maintStudentScores_Load(object sender, EventArgs e)
{
students = StudentDB.GetStudents();
FillStudentListBox();
}
private void FillStudentListBox()
{
lstBStudents.Items.Clear();
foreach(Student s in students)
{
lstBStudents.Items.Add(s.GetDisplayText());
}
}
private void bttnAddNewStudent_Click(object sender, EventArgs e)
{
addNewStudent newNewStudentForm = new addNewStudent();
Student student = newNewStudentForm.GetNewStudent();
if(student != null)
{
students.Add(student);
StudentDB.SaveStudent(students);
FillStudentListBox();
}
}
}
}
addNewStudent.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace MaintainStudentScores_3
{
public partial class addNewStudent : Form
{
List tempList = new List();
public addNewStudent()
{
InitializeComponent();
}
private Student student = null;
public Student GetNewStudent()
{
this.ShowDialog();
return student;
}
private void bttnOk_Click(object sender, EventArgs e)
{
//Students = new List();
if (Validator.IsPresent(tBoxStudentName, \"Name\"))
{
student = new Student(tBoxStudentName.Text, tempList);
this.Close();
}
}
private void bttnAddScores_Click(object sender, EventArgs e)
{
if (IsValidData())
{
tempList.Add(Convert.ToInt32(tBoxScore.Text));
string total = \"\";
foreach(int scores in tempList)
{
total += Convert.ToString(scores) + \" \";
}
tBoxAllScores.Text = total;
}
}
private bool IsValidData()
{
return Validator.IsPresent(tBoxScore, \"Score\") && Validator.IsWithinRange(tBoxScore, \"score\", 0, 100);
}
private void bttnClear_Click(object sender, EventArgs e)
{
tempList.Clear();
tBoxAllScores.Text = String.Empty;
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
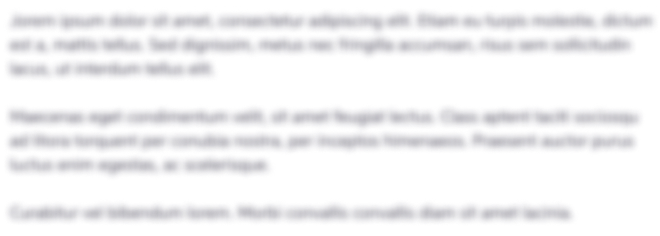
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started