Question
Make a GUI and build on the previous assign.Need this in java. I have added the code from Assign2 below. Also, please add lots of
Make a GUI and build on the previous assign.Need this in java. I have added the code from Assign2 below. Also, please add lots of comments so that I know what you're doing because I am fairly new to java
This is the code from the previous assignment that you're supposed to build on to and make sure to change the instance variables to protected here:
public class Money { private int dollars; // dollars and cents are the 2 instance data variables private int cents; public void add(Money moneyIn) // adding current object to money.In { this.dollars = this.dollars + moneyIn.dollars; //this. operator means the current object this.cents = this.cents + moneyIn.cents; adjust(); //calling adjust so that it stays within range } public void subtract(Money moneyIn) //subtract moneyIn from current object { this.dollars = this.dollars - moneyIn.dollars; // current object dollar is this.dollars and moneyIn object dollars is moneyIn.dollars this.cents = this.cents - moneyIn.cents; adjust(); //invoke adjust method to make sure it's not negative } public int compareTo(Money moneyIn) // compares current object with moneyIn { if (this.dollars moneyIn.dollars || this.cents > moneyIn.cents) return 1; //if current object > money.In else return 0; //if current object == money.In } public boolean equals(Money moneyIn) //to see if current object == moneyIn { if (this.dollars == moneyIn.dollars && this.cents == moneyIn.cents) return true; //if current object == moneyIn
else return false; //current object !==moneyIn } public String toString() { // after the adjust function your this.cents=33 and this.dollars=10 String centsString; String dollarsString; if(this.dollars == 0) { dollarsString = "0"; } else { dollarsString = Integer.toString(this.dollars); // dollars = 5 and cents = 5; toString: 5$.2cc // dollarsString = "5" (add quotation marks for String) } if(this.cents == 0) { centsString = "00"; } else if (this.cents > 0 && this.cents
this.dollars = dollarsIn; // moneyObj.dollars = 7 this.cents = centsIn; // money.Obj.cents = 33 adjust();
} public Money (Money other) // passing a parameter other of data type money. // So 'other' parameter now has dollars and cents as class variables and 8 functions // My 'other' is assigned to moneyObj; other=moneyObj { dollars = other.dollars; // dollars alone means it is this.dollars; current object's dollar; current object here is moneyObj2 cents = other.cents; // moneyObj2.dollars=moneyObj.dollars = 7 // moneyObj2.cents=moneyObj.cents = 33 }
private void adjust() // adjusting dollars and cents { while (this.cents > 99) { this.cents = this.cents - 100; // so that cents are not above 99 this.dollars++; // this.dollars = this.dollars + 1 } if(this.cents 0) { this.dollars = this.dollars - 1; this.cents = this.cents+100; } if(this.dollars 0) { this.dollars = 0; this.cents = 0; } } }
This assignment builds on the Money class from assignment 2 and builds a simple GUI interface Required package name: assign4 Submit all your Java files 1. Implement the hierarchy for the Money and Change classes Change Money dollars: int quarters: int dimes: int cents: int nickels: int add(Money): void pennies: int subtract (Money): void singles: int compare To(Money): int N fives: int equals(Money): boolean makeChange(): void toString0: String getQuarters0: int Money (int, int) getDimes(): int Money (Money) getNickets(): int adjust 0: void getSingles(): int getFives int 0: get Pennies(): int Change(int, int) Notice that there is only one small change made to the Money class: both instance variables are now protected rather than private. The method makeChange should set the instances variables in the Change class so that the least number of bills and coins are set. This means the change for $1.52 should be 1 single, 2 quarters and 2 cents-not 152 pennies. There is also a simple constructor for Change that can use the parent constructor if desired. 2. Create a simple GUI for a program to make and display change using the Change class aboveStep by Step Solution
There are 3 Steps involved in it
Step: 1
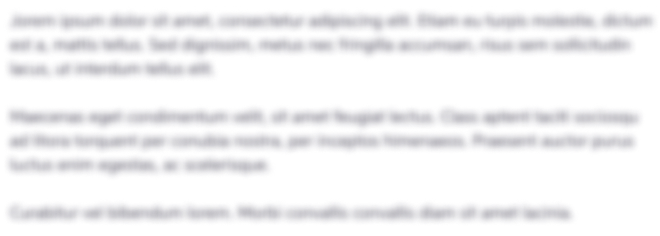
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started