Question
Make a GUI for JAVA with this code and needs to have Exception Handling. public class Rational { private long numer; private long denom; public
Make a GUI for JAVA with this code and needs to have "Exception Handling".
public class Rational {
private long numer;
private long denom;
public Rational() {// TODO Auto-generated constructor stub
this.numer = 0;
this.denom = 1;
}
public Rational(long a)
{
this.numer = a;
this.denom = 1;
}
//
public Rational(long a, long b) throws ArithmeticException
{
this.numer = a;
this.denom = b;
long x = gcd(this.numer, this.denom);
// reduce numer and denom to simplest terms
this.numer /= x;
this.denom /= x;
}
//
public long getDenominator()
{
return denom;
}
//
public long getNumerator()
{
return numer;
}
//
public Rational add(Rational r)
{
// calculate the numerator of the final Rational number
long num = this.numer * r.getDenominator() + r.getNumerator() * this.denom;
// calculate the denominator of the final Rational number
long den = this.denom * r.getDenominator();
long x = gcd(num, den);
// reduce num and den to simplest terms
num /= x;
den /= x;
return new Rational(num, den);
}
//
public Rational subtract(Rational r)
{
// calculate the numerator of the final Rational number
long num = this.numer * r.getDenominator() - r.getNumerator() * this.denom;
// calculate the denominator of the final Rational number
long den = this.denom * r.getDenominator();
long x = gcd(num, den);
// reduce num and den to simplest terms
num /= x;
den /= x;
return new Rational(num, den);
}
//
public Rational multiply(Rational r)
{
// calculate the numerator of the final Rational number
long num = this.numer * r.getNumerator();
// calculate the denominator of the final Rational number
long den = this.denom * r.getDenominator();
long x = gcd(num, den);
// reduce num and den to simplest terms
num /= x;
den /= x;
return new Rational(num, den);
}
//
public Rational divide(Rational r) throws ArithmeticException
{
// calculate the numerator of the final Rational number
long num = this.numer * r.getDenominator();
// calculate the denominator of the final Rational number
long den = this.denom * r.getNumerator();
long x = gcd(num, den);
// reduce num and den to simplest terms
num /= x;
den /= x;
return new Rational(num, den);
}
//
// Find the Greatest Common Divisor of Two Integers
//
public long gcd(long p, long q)
{
if(p % q == 0)
return q;
else
return gcd(q, p % q);
}
public String toString()
{
if (denom == 1)
return "" + numer;
return numer + "/" + denom;
}
public int compareTo(Object obj)
{
if (obj == null) {
return -1;
}
if (!(obj instanceof Rational)) {
return 1;
}
if (this.equals((Rational)(obj)))
return 0;
return (this.subtract((Rational)(obj)).getNumerator() > 0 ? 1 : -1);
}
// Returns true if and only if object are the same number or reference the same object
public boolean equals(Object obj)
{
if (obj == null) {
return false;
}
if (obj == this) {
return true;
}
if (this.subtract((Rational)obj).getNumerator() == 0)
return true;{
return false;
}
}
}
public class RationalTester {
public static void main(String[] args) {
// TODO Auto-generated method stub
Rational ob1 = new Rational();
System.out.println("ob1 : " + ob1);
Rational ob2 = new Rational(5);
System.out.println(" ob2 : " + ob2);
Rational ob3 = new Rational(6, 4);
System.out.println(" ob3 : " + ob3);
Rational ob4 = ob1.add(ob2);
System.out.println(" ob1 + ob2 : " + ob4);
Rational ob5 = ob2.subtract(ob3);
System.out.println(" ob2 - ob3 : " + ob5);
Rational ob6 = ob2.multiply(ob3);
System.out.println(" ob2 * ob3 : " + ob6);
Rational ob7 = ob2.divide(ob3);
System.out.println(" ob2 / ob3 : " + ob7);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
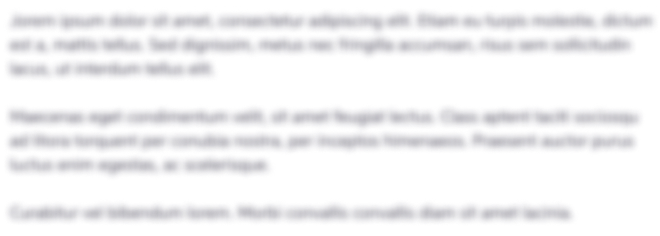
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started