Question
Make JAVADOC for this code plz like comments or something. ===================== package sample; /* * Java Program to Implement Circular Doubly Linked List */ /**
Make JAVADOC for this code plz like comments or something.
=====================
package sample;
/*
* Java Program to Implement Circular Doubly Linked List
*/
/**
* Class Node
* This class is used to represent a node in the circular doubly linked list
**/
class DLNode
{
protected int data;
protected DLNode next, prev;
/* Constructor */
public DLNode()
{
next = null;
prev = null;
data = 0;
}
/* Constructor */
public DLNode(int data, DLNode next, DLNode prev)
{
this.data = data;
this.next = next;
this.prev = prev;
}
/* Function to set link to next node */
public void setLinkNext(DLNode n)
{
next = n;
}
/* Function to set link to previous node */
public void setLinkPrev(DLNode p)
{
prev = p;
}
/* Function to get link to next node */
public DLNode getLinkNext()
{
return next;
}
/* Function to get link to previous node */
public DLNode getLinkPrev()
{
return prev;
}
/* Function to set data to node */
public void setData(int d)
{
data = d;
}
/* Function to get data from node */
public int getData()
{
return data;
}
}
/* Class linkedList */
public class CircularDoublyLinkedList {
private DLNode head;
private DLNode tail ;
public int size;
/* Constructor */
public CircularDoublyLinkedList()
{
head = null;
tail = null;
size = 0;
}
/* Function to check if list is empty */
public boolean isEmpty()
{
return head == null;
}
/* Function to get size of list */
public int getSize()
{
return size;
}
/* Function to insert element at begining */
public void addAtHead(int val)
{
DLNode nptr = new DLNode(val, null, null);
if (head == null)
{
nptr.setLinkNext(nptr);
nptr.setLinkPrev(nptr);
head = nptr;
tail = head;
}
else
{
nptr.setLinkPrev(tail);
tail.setLinkNext(nptr);
head.setLinkPrev(nptr);
nptr.setLinkNext(head);
head = nptr;
}
size++ ;
}
/*Function to insert element at tail */
public void addAtTail(int val)
{
DLNode nptr = new DLNode(val, null, null);
if (head == null)
{
nptr.setLinkNext(nptr);
nptr.setLinkPrev(nptr);
head = nptr;
tail = head;
}
else
{
nptr.setLinkPrev(tail);
tail.setLinkNext(nptr);
head.setLinkPrev(nptr);
nptr.setLinkNext(head);
tail = nptr;
}
size++;
}
/* Function to insert element at position */
public void addAtIndex(int val , int index)
{
DLNode nptr = new DLNode(val, null, null);
if (index == 0)
{
addAtHead(val);
return;
}
DLNode ptr = head;
for (int i = 1; i < size; i++)
{
if (i == index)
{
DLNode tmp = ptr.getLinkNext();
ptr.setLinkNext(nptr);
nptr.setLinkPrev(ptr);
nptr.setLinkNext(tmp);
tmp.setLinkPrev(nptr);
}
ptr = ptr.getLinkNext();
}
size++ ;
}
/* Function to delete node at position */
public void removeAtIndex(int index)
{
if (index == 0)
{
if (size == 1)
{
head = null;
tail = null;
size = 0;
return;
}
head = head.getLinkNext();
head.setLinkPrev(tail);
tail.setLinkNext(head);
size--;
return ;
}
if (index == size-1)
{
tail = tail.getLinkPrev();
tail.setLinkNext(head);
head.setLinkPrev(tail);
size-- ;
}
DLNode ptr = head.getLinkNext();
for (int i = 2; i <= size; i++)
{
if (i == index)
{
DLNode p = ptr.getLinkPrev();
DLNode n = ptr.getLinkNext();
p.setLinkNext(n);
n.setLinkPrev(p);
size-- ;
return;
}
ptr = ptr.getLinkNext();
}
}
/* Function to return a string representation of the circular doubly linked list*/
public String toString()
{
System.out.print(" Circular Doubly Linked List = ");
DLNode ptr = head;
if (size == 0)
{
System.out.print("empty ");
return "";
}
if (head.getLinkNext() == head)
{
return head.getData() + " -> "+ptr.getData()+ " ";
}
String res = head.getData() + " -> ";
ptr = head.getLinkNext();
while (ptr.getLinkNext() != head)
{
res += ptr.getData()+ " -> ";
ptr = ptr.getLinkNext();
}
res += ptr.getData()+ " -> ";
ptr = ptr.getLinkNext();
res += ptr.getData()+ " ";
return res;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
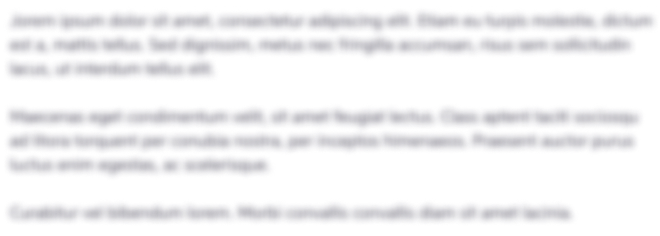
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started