Question
Make sure the program is asking for user input. (1)Objective: Implement java.util.Comparator to determine the order/match of arbitrary objects. (1 points) Write a java program
Make sure the program is asking for user input.
(1)Objective: Implement java.util.Comparator to determine the order/match of arbitrary objects. (1 points)
Write a java program to determine the order of two shape objects by comparing their area.
For example: determine the order of
(a)A 20x5 rectangular.
(b)A circle with radius 6.
Allow user to input length & width for the rectangular and radius for the circle to test your program. Use the Circle.java, Rectangular.java and Shape.java files found below.
//******************************************************************** // Circle.java // //********************************************************************
import java.text.*;
public class Circle extends Shape { private int radius, x, y;
//----------------------------------------------------------------- // Constructor: Sets up this circle with the specified values. //----------------------------------------------------------------- public Circle (int size) { radius = size; } //----------------------------------------------------------------- // Diameter mutator. //----------------------------------------------------------------- public void setRadius (int size) { radius = size; }
//----------------------------------------------------------------- // Diameter accessor. //----------------------------------------------------------------- public int getRadius () { return radius; } //--------------------------------------------------------------------------- // Returns the calculated value of the area. //--------------------------------------------------------------------------- public double computeArea() { return 3.1416*(radius)*(radius); } //--------------------------------------------------------------------------- // Returns the calculated value of the perimeter. //---------------------------------------------------------------------------
public double computePerimeter() { return 3.1416 * 2 * radius; }
}
//****************************************************************************** // Rectangle.java Author: Lewis/Loftus // // Solution to Programming Project 9.11 //******************************************************************************
import java.text.*;
public class Rectangle extends Shape { protected double width; protected double length; protected static DecimalFormat form = new DecimalFormat("0.##");
//--------------------------------------------------------------------------- // Sets up the rectangle by entering its width and length. //--------------------------------------------------------------------------- public Rectangle(double wid, double len) { width = wid; length = len; }
//--------------------------------------------------------------------------- // Returns the double value of the width. //--------------------------------------------------------------------------- public double getWidth() { return width; } //--------------------------------------------------------------------------- // Returns the double value of the length. //--------------------------------------------------------------------------- public double getLength() { return length; } //--------------------------------------------------------------------------- // Returns the calculated value of the area. //--------------------------------------------------------------------------- public double computeArea() { return length * width; } //--------------------------------------------------------------------------- // Returns the calculated value of the perimeter. //--------------------------------------------------------------------------- public double computePerimeter() { return 2 * (length + width); }
//--------------------------------------------------------------------------- // Returns pertinent information about the rectangle. //--------------------------------------------------------------------------- public String toString() { return "Rectangle: width is " + form.format(width) + ", length is " + form.format(length) + " perimeter is " + form.format(computePerimeter()) + ", area is " + form.format(computeArea()); } }
//****************************************************************************** // Shape.java // //******************************************************************************
public abstract class Shape { abstract public double computeArea(); abstract public double computePerimeter(); }
Make sure the program asks for user input to get length & width for the rectangular and radius for the circle.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
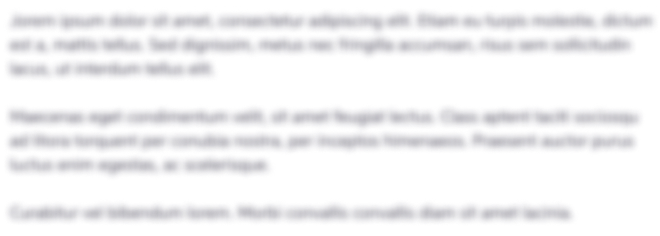
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started