Question
Make the following two classes (you can add other methods, Constructors, and instance variables, if necessary ): 1.) class Triangle Instance Variables: height, base Methods:
Make the following two classes (you can add other methods, Constructors, and instance variables, if necessary):
1.) class Triangle
Instance Variables: height, base
Methods: setHeight(), setBase(),getHeight(), getBase(), displayArea().
2.) class Circle
Instance Variables: radius
Methods: setRadius(),getRadius(),displayArea().
Use the following Tester class (you are NOT allowed to add any code to the Tester class)
public class Tester {
public static void main(String[] args) {
Triangle myTriangle = new Triangle();
Triangle yourTriangle = new Triangle();
Circle myCircle = new Circle(6);
Circle yourCircle = new Circle(6);
myTriangle.setHeight(8);
myTriangle.setBase(4.7);
myTriangle.displayArea();
System.out.println(myTriangle == yourTriangle);
System.out.println(myCircle == yourCircle);
System.out.println(myTriangle.equals(yourTriangle));
System.out.println(myCircle.equals(yourCircle));
System.out.println(myTriangle.toString());
System.out.println(Circle.radius);
System.out.println(Circle.displayArea(5));
System.out.println(yourCircle.displayArea());
}
}
The output should be:
0.0
false
false
false
true
This is a triangle
6.0
78.5
113.097
Step by Step Solution
There are 3 Steps involved in it
Step: 1
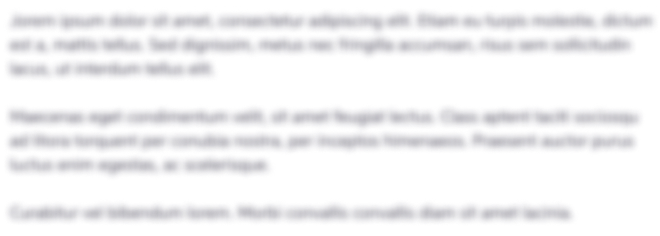
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started