Question
// method to return the top element of the stack public Object peek() { if (!isEmpty()) return stack[top]; return null ; } // method to
// method to return the top element of the stack
public Object peek() {
if (!isEmpty())
return stack[top];
return null;
}
// method to insert element at top of the stack
public void push(Object data) {
if (!isFull()) {
top++;
stack[top] = data;
} else
System.out.println(" Stack is full");
}
// method to delete top element of the stack
public Object pop() {
if (!isEmpty()) {
Object data = stack[top];
top--;
return data;
} else {
System.out.println(" Empty Stack");
return null;
}
}
// method to get the number of elements in the stack
public int getSize() {
return (top + 1);
}
// method to get the total capacity of the stack
public int getCapacity() {
return stack.length;
}
// method to get the remaining number of elements that can be inserted in the
// stack
public int getRemainingCapacity() {
return (getCapacity() - getSize());
}
// method to check if the stack is full
public boolean isFull() {
return (getRemainingCapacity() == 0);
}
// method to check if stack is empty
public boolean isEmpty() {
return (top == -1);
}
// method to return the string representation of the stack
public String toString() {
if (isEmpty()) {
return "Empty Stack";
} else {
String stkStr = "";
int i = top;
while (i >= 0) {
stkStr += stack[i] + " ";
i--;
}
return stkStr;
}
}
// method to add all the elements of the passed array to the stack, return true
// if successful else false
public boolean addAll(Object[] arr) {
if (getRemainingCapacity() == arr.length) {
for (int i = 0; i
push(arr[i]);
return true;
}
return false;
}
// method to return if the passed object is present in the stack
public boolean contains(Object obj) {
for (int i = top; i >= 0; i--)
if (stack[i].equals(obj)) {
return true;
}
return false;
}
// method to return if all the elements in passed object array are present in
// the stack or not
public boolean containsAll(Object[] arr) {
for (int i = 0; i
if (!contains(arr[i])) {
return false;
}
return true;
}
// method to return an array representation of the stack where index 0 is the
// bottom of the stack
public Object[] toArray() {
if (isEmpty()) {
return null;
}
Object stk[] = new Object[top + 1];
for (int i = 0; i
stk[i] = stack[i];
return stk;
}
}
Java Code
Need Junit 4 test Cases for the above methods example of one of them is
Step by Step Solution
There are 3 Steps involved in it
Step: 1
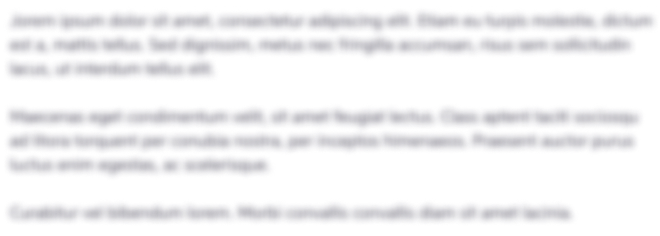
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started