Question
Milestone 3 Streamable Interface module and Publication class module An Interface is an abstract base class with only pure virtual functions. This milestone will include
Milestone 3
Streamable Interface module and Publication class module
An Interface is an abstract base class with only pure virtual functions.
This milestone will include the Date and Utils modules.
Before starting to develop milestone 3, please apply the following modifications to the Date module:
In Date.cpp
In Date::read() method: remove "flushing the keyboard" (i.e. cin.ignore(1000,' ')) at the end of your read function. The read function should not flush the keyboard when the reading process is done.
Add the following global variable (for testing purposes) to the top of the sdds namespace in Date.cpp.
bool sdds_test = false; int sdds_year = 2021; int sdds_mon = 12; int sdds_day = 25;
- replace systemYear and setToToday methods with the following:
int Date::systemYear()const { int theYear = sdds_year; if (!sdds_test) { time_t t = time(NULL); tm lt = *localtime(&t); theYear = lt.tm_year + 1900; } return theYear; } void Date::setToToday() { if (sdds_test) { m_day = sdds_day; m_mon = sdds_mon; m_year = sdds_year; } else { time_t t = time(NULL); tm lt = *localtime(&t); m_day = lt.tm_mday; m_mon = lt.tm_mon + 1; m_year = lt.tm_year + 1900; } errCode(NO_ERROR); }
in Date.h
- Add the following to the bottom of the sdds namespace; This will make the global variables added to Date.cpp, truly global for any code including "Date.h".
extern bool sdds_test; extern int sdds_year; extern int sdds_mon; extern int sdds_day;
Types of publications in Seneca Library
Seneca Library holds two types of Publication; Periodical (like newspapers and Magazines) and Books.
Publications are not loaned out to members. Members can check them out and read them in the library. These publications are put back on their shelves at the end of the day.
Books can be borrowed and returned within 15 days. After 15 days, the member will be charged daily for a late penalty.
General definitions of the library system.
Create a header file called "Lib.h" to hold all the general values of the system. Add the following and any other general values you find necessary to this header file. This header file should be included anywhere any of these values are needed.
const int SDDS_MAX_LOAN_DAYS = 15; // maximum number of day a publication can be borrowed with no penalty const int SDDS_TITLE_WIDTH = 30; // The width in which the title of a publication should be printed on the console const int SDDS_AUTHOR_WIDTH = 15; // The width in which the author name of a book should be printed on the console const int SDDS_SHELF_ID_LEN = 4; // The width in which the shelf id of a publication should be printed on the console const int SDDS_LIBRARY_CAPACITY = 5000; // Maximum number of publications the library can hold.
Streamable Interface Modules.
Create an Interface called Streamable to enforce implementation of the following methods. Any class inherited from Streamable will have the capability to be inserted into or extracted from a stream.
Streamable Pure Virtual funcitons
Add the following pure virtual function to Streamable:
write pure virtual function
This method is not capable of modifying the Streamable object. write receives and returns a reference of an ostream object.
Functions overriding this function will write into an ostream object.
read pure virtual function
read receives and returns a reference of an istream object.
Functions overriding this function will read from an istream object.
conIO
This method is not capable of modifying the Streamable object. conIo receives a reference of an ios object and returns a Boolean.
Functions overriding this function will determine if the incoming ios object is a console IO object or not.
Boolean conversion operator overload (pure virtual)
Boolean conversion overloads of this method will return if the Streamable object is in a valid state or not.
destructor
Add an empty virtual destructor to this interface to guaranty that the descendants of the Streamable are removed from memory with no leak.
Insertion operator overload
Overload the insertion operator so a constant object of type Streamable can be written on an ostream object only if the Streamable object is in a valid state. Otherwise, the function will quietly ignore the insertion action.
Extraction operator overload
Overload the extraction operator so an object of type Streamable can be read from an istream object.
Publication class module
The publication class is a general encapsulation of any periodic publication.
Later by adding an author to the descendant of the Publication class we will encapsulate a Book for the system.
Publication Attributesm_title
m_title is a c-string to hold a dynamic title for the publication.
To ease the implementation, let's assume this dynamic array can not be more than 255 characters long.
This attribute is null by default.
m_shelfId
Hold the location of the publication in the library. m_shelfId is a c-string that is exactly 4 characters long.
MS3 V1.1
This attribute is an empty string by default.
m_membership
This attribute is an integer to hold a 5 digit membership number of members of the library.
In this class, if the membership number is '0', it means the publication is available and is not checked out by any members.
If the membership number is a five-digit number, it means the publication is checked out by the member holding that membership number.
This attribute is zero by default.
m_libRef
This serial number is used internally to uniquely identify each publication in the system.
This attribute is -1 by default.
m_date
A Date object.
In periodical publications, this date is used for the publish date of the item.
In Books, this date is used to keep the date on which the book was borrowed by the member.
The Date, by default, is set to the current date.
Constructor (default)
Sets all the attributes to their default values.
MethodsModifiers
virtual void set(int member_id); // Sets the membership attribute to either zero or a five-digit integer. void setRef(int value); // Sets the **libRef** attribute value void resetDate(); // Sets the date to the current date of the system.
Queries
virtual char type()const; //Returns the character 'P' to identify this object as a "Publication object" bool onLoan()const; //Returns true is the publication is checkout (membership is non-zero) Date checkoutDate()const; //Returns the date attribute bool operator==(const char* title)const; //Returns true if the argument title appears anywhere in the title of the //publication. Otherwise, it returns false; (use strstr() function in
Streamable pure virtual function implementationsbool conIO(ios& io)const
Returns true if the address of the io object is the same as the address of either the cin object or the cout object.
ostream& write(ostream& os) const
If the os argument is a console IO object (use conIO()), print shelfId, title, membership and date attributes as the following format (title is left-justified padded with dots)
MS3 V1.1.1
1 2 3 4 5 6 7 1234567890123456789012345678901234567890123456789012345678901234567890 | P001 | The Toronto Star.............. | 34037 | 2021/11/17 | | P007 | Macleans Magazine............. | N/A | 2021/11/11 |
Otherwise:
Print the type() and then libRef, shelfId, title, membership and date attributes in a tab-separated format.
P 2001 P001 The Toronto Star 34037 2021/11/17
No newline is printed afterwards either way.
istream& read(istream& istr)
Read all the values in local variables before setting the attributes to any values.
First, clear all the attributes by freeing the memory and setting everything to their default values.
Then, if the istr argument is a console IO object (use conIO()) read the attributes as follows:
- prompt: "Shelf No: "
- read the shelf number up to its limit (see Lib.h).
- if the number is not exactly the length it is supposed to be, set the istr to a fail state manually.
- prompt: "Title: "
- read the title
- prompt: "Date: "
- read the date
in this case the libRef value is left with its default value.
Otherwise, assume reading begins with libRef attribute as if the type 'P' is not in the file.
- read the libRef number
- skip the tab
- read the shelf number
- skip the tab
- read the title
- skip the tab
- read the membership
- skip the tab
- read the date
Either way if the date is in an invalid state set the istr to a fail state manually
After the process of reading is done if istr is in a valid state:
- Dynamically store the title into the title attribute
- copy the shelf id into the shelfId attribute
- set the membership
- set the date
- set the libRef attribute
At the end return the istr argument.
operator bool() const
Returns true if neither of the title or shelfId attributes is null or empty. Otherwise, it will return false.
The Rule of three
Make sure this object complies with the guidelines of the Rule of three so the copying and assignment and destruction are done safely and without any memory leak.
the Tester program
// Final Project Milestone 3 // Periodical Publication // File ms3_tester.cpp // Version 1.0 // Author Fardad Soleimanloo // Revision History // ----------------------------------------------------------- // Name Date Reason // Fardad 2021/11/15 Preliminary release ///////////////////////////////////////////////////////////////// #include
Tester Output
MS3 tester output
MS3 Submission and the due date
If you would like to successfully complete the project and be on time, start early and try to meet all the due dates of the milestones.
Upload your source code and the tester program (Utils.cpp, Utils.h, Date.cpp, Date.h, Streamable.cpp, Streamable.h, Publication.cpp, Publication.h,Periodicals.txt, and ms3_tester.cpp) to your matrix account. Compile and run your code using the g++ compiler as shown in the introduction and make sure that everything works properly.
Then, run the following command from your account (replace profname.proflastname with your professor's Seneca userid):
~profname.proflastname/submit 2??/prj/m?
and follow the instructions.
- 2?? is replaced with your subject code
- m? is replaced with your milestone
The submit program's options:
~prof_name.prof_lastname/submit DeliverableName [-submission options]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
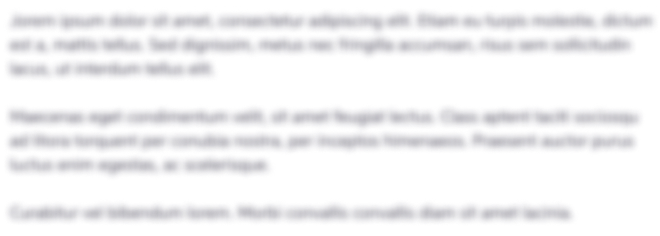
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started