Question
Mini-Project: Portfolio Generator In this activity, you will build a command-line tool that generates an HTML portfolio page from user input. ## Instructions * Your
Mini-Project: Portfolio Generator
In this activity, you will build a command-line tool that generates an HTML portfolio page from user input.
## Instructions
* Your application should prompt the user for information like their name, location, bio, LinkedIn URL, and GitHub URL. Feel free to add any additional prompts you think of.
* An HTML document containing the information collected from the prompts should be constructed and written to the file system. Make sure to add some CSS styling to the document.
* Youll need the following tools and technologies to accomplish this:
* `fs` for writing to the file system
* `inquirer` version 8.2.4 for collecting user input
* String template literals for generating a string version of the HTML document before it is written to the file system
sample code 1:
const inquirer = require('inquirer');
const fs = require('fs');
const generateHTML = ({ name, location, github, linkedin }) =>
`
Hi! My name is ${name}
I am from ${location}.
Example heading Contact Me
- My GitHub username is ${github}
- LinkedIn: ${linkedin}
`;
inquirer
.prompt([
{
type: 'input',
name: 'name',
message: 'What is your name?',
},
{
type: 'input',
name: 'location',
message: 'Where are you from?',
},
{
type: 'input',
name: 'hobby',
message: 'What is your favorite hobby?',
},
{
type: 'input',
name: 'food',
message: 'What is your favorite food?',
},
{
type: 'input',
name: 'github',
message: 'Enter your GitHub Username',
},
{
type: 'input',
name: 'linkedin',
message: 'Enter your LinkedIn URL.',
},
])
.then((answers) => {
const htmlPageContent = generateHTML(answers);
fs.writeFile('index.html', htmlPageContent, (err) =>
err ? console.log(err) : console.log('Successfully created index.html!')
);
});
Sample code 2:
const inquirer = require('inquirer');
// Node v10+ includes a promises module as an alternative to using callbacks with file system methods.
const { writeFile } = require('fs').promises;
// Use writeFileSync method to use promises instead of a callback function
const promptUser = () => {
return inquirer.prompt([
{
type: 'input',
name: 'name',
message: 'What is your name?',
},
{
type: 'input',
name: 'location',
message: 'Where are you from?',
},
{
type: 'input',
name: 'hobby',
message: 'What is your favorite hobby?',
},
{
type: 'input',
name: 'food',
message: 'What is your favorite food?',
},
{
type: 'input',
name: 'github',
message: 'Enter your GitHub Username',
},
{
type: 'input',
name: 'linkedin',
message: 'Enter your LinkedIn URL.',
},
]);
};
const generateHTML = ({ name, location, github, linkedin }) =>
`
Hi! My name is ${name}
I am from ${location}.
Example heading Contact Me
- My GitHub username is ${github}
- LinkedIn: ${linkedin}
`;
// Bonus using writeFileSync as a promise
const init = () => {
promptUser()
// Use writeFile method imported from fs.promises to use promises instead of
// a callback function
.then((answers) => writeFile('index.html', generateHTML(answers)))
.then(() => console.log('Successfully wrote to index.html'))
.catch((err) => console.error(err));
};
init();
Step by Step Solution
There are 3 Steps involved in it
Step: 1
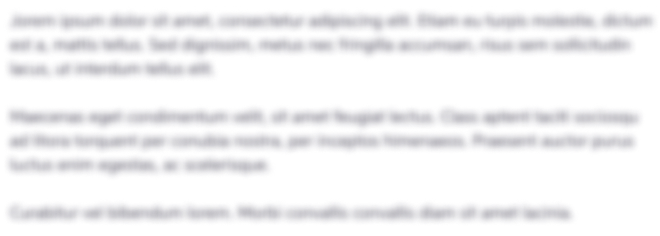
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started