Question
MIPS Decoder. For this assignment, you will write a program to accept machine code (as hexadecimal strings) from the user, decode and report certain features
MIPS Decoder.
For this assignment, you will write a program to accept machine code (as hexadecimal strings) from the user, decode and report certain features of the decoded MIPS instruction.
Main program: Your main program must perform the following tasks:
Accept a machine instruction word and its address as two strings from the user.
Extract the numeric value from the input strings.
Call subroutine get_type(details below) and print the format type (I/J/R) of the instruction. If the instruction is not supported, print "invalid".
Call subroutine get_dest_reg(details below) and print the destination register number of the instruction. The register number must be printed out as a decimal value. If there is no destination register, print "none". If the specified destination register is wrong or instruction is not supported, print "invalid".
Call subroutine get_next_pc(details below) and print the address(es) of the next instruction. The addresses must be printed out as hexadecimal values. If the instruction is not supported, print "invalid".
Assumptions:
o The inputs from the user (to specify an instruction word and its address) are strings of 8-character long excluding the ending newline. They are valid hexadecimal values, for example "00811002" or "A0A110DB".
o You can assume that the input address is always a valid one following MIPS conventions and there is no need for your program to check that. You do not need to check the range of the return of get_next_pc() either. o You can assume only capital case letters ('A' to 'F') will be used for the input.
o You can assume only a subset of MIPS instructions are supported: add, addi, sub, slt, lw, sw, beq, j.
Required subroutines:
int get_type(int instruction)
Parameter: instruction is a 32-bit unsigned number representing a machine instruction word.
Return: an integer with the following possible values
o 1-R type, 2-I type, 3-J type, 0-not supported
int get_dest_reg(int instruction)
Parameter: instruction is a 32-bit unsigned number representing a machine instruction word.
Return: an integer representing the register number which will be updated by executing of this instruction. A valid return should be within the range of [0,31]. Return 32 if no register gets updated by the instruction. Return 0 for invalid instructions or invalid destination register. Note: some instruction might update $rt instead of $rd.
int get_next_pc(int instruction, int addr)
Parameters: instruction is a 32-bit unsigned number representing a machine instruction word; addr is a 32-bit unsigned number representing the address (PC) of instruction.
Return:
o For sequential and jump instructions, return an integer representing the address of the next instruction we will fetch and execute in $v0;
o For branch instructions, return two integers representing the addresses of the next instruction we will fetch and execute if branch is taken (in $v1) and the next instruction if branch is not taken (in $v0).
o You can assume the next pc is never going to be zero and return 0 in $v0 for invalid instructions.
Coding Requirements:
You must mark clear the start and end of all three required subroutines.
You must declare all three required subroutines to be global. For example, use these two lines to start your subroutine get_type(): .globl get_type get_type:
Hints:
You may need to use la to load an address into a register.
ASCII encoding of symbols can be useful. You can turn on/off "ASCII" in MARS to inspect the memory content for the input string.
Feel free to define additional helper functions.
You may find system calls useful to read in string and/or print a value in hex or other formats. Print out binary patterns can help with debugging.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
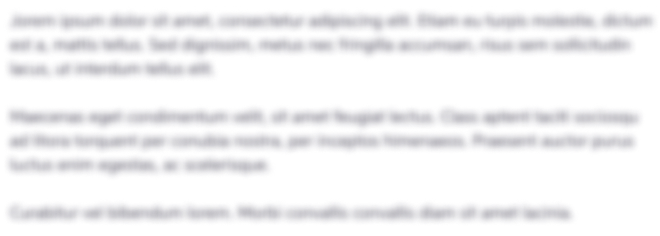
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started